Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial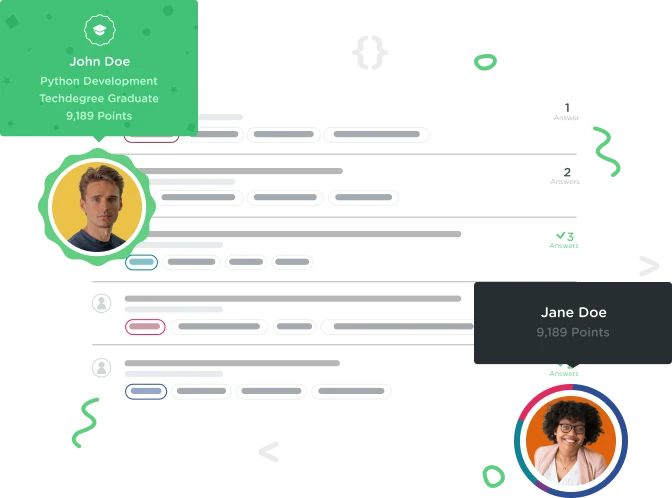
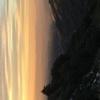
Sergio Romero
3,665 PointsSwift Classes with Custom Types
I need help I have been stuck on this code challenge for a few days I just don't understand it, can someone help me? Code Challenge: "In the editor you've been provided with a struct named Location that models a coordinate point using longitude and latitude values.
For this task we want to create a class named Business. The class contains two constant stored properties: name of type String and location of type Location.
In the initializer method pass in a name and an instance of Location to set up the instance of Business. Using this initializer, create an instance and assign it to a constant named someBusiness."
struct Location {
let latitude: Double
let longitude: Double
}
class Business {
let name: String
let location: Location
init(name: String, latitude: Double, longitude: Double){
self.name = name
self.location = Location(latitude: Double, longitude: Double)
}
}
let someBusiness = Business(name: "Sergio", location: (14.0, 10.0))
2 Answers
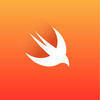
Steven Deutsch
21,046 PointsHey Sergio Romero,
The first problem is with your initializer call for creating an instance of Business. Your initializer takes 3 arguments, name of type String, latitude of type Double, and longitude of type Double. This means when calling your initializer you must pass in 3 values of these same types.
The second problem is how you set up your Business initializer. Inside of it you are trying to create an instance of Location and assign it to location property of Business. This is fine but once again you can't create an instance without passing it in the values it requires. What you need to do is use the values you are passing into the Business initializer, longitude and latitude, to create the Location instance. Take a look at the code below:
struct Location {
let latitude: Double
let longitude: Double
}
class Business {
let name: String
let location: Location
init(name: String, latitude: Double, longitude: Double){
self.name = name
// The values you pass into the init will be used to create the Location instance
self.location = Location(latitude: latitude, longitude: longitude)
}
}
// Notice I'm calling the Business initializer and passing it the 3 values it requires
let someBusiness = Business(name: "Sergio", latitude: 40.0, longitude: 40.0)
Hope this helps. Good Luck!
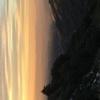
Sergio Romero
3,665 Pointsthanks Steven. I understand more than I did 20 minutes ago.
Sergio Romero
3,665 PointsSergio Romero
3,665 PointsThank You!!! I understand this but at the same time I don't get why you did (latitude: latitude, longitude: longitude).
Steven Deutsch
21,046 PointsSteven Deutsch
21,046 PointsHey sergio,
Those are the names of the arguments you are passing into the Business initializer. We are using those values by their names to create the instance of Location. It's the same as using the name argument you pass in and assigning it to the property self.name.
Seth Roope
6,471 PointsSeth Roope
6,471 PointsWhat is the point of using the "Location" struct if you are just going to initialize "latitude" and "longitude"? Shouldn't you be initializing "location" as an instance of "Location"? Looks like you could just get rid of the struct altogether in your code...