Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial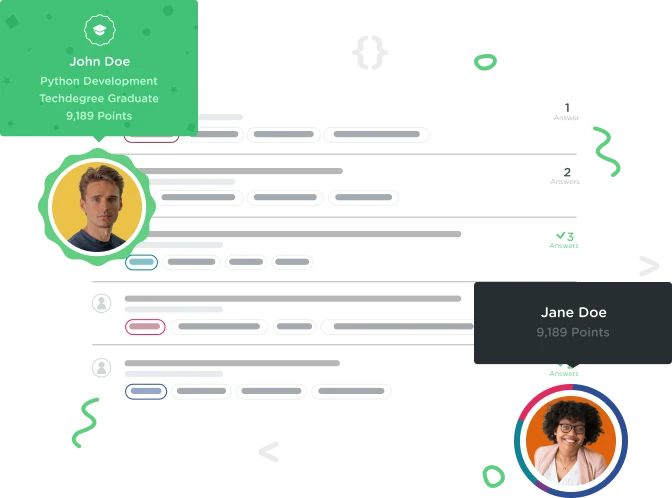

Daniel Lambrecht
iOS Development Techdegree Student 5,302 PointsSwift countdown timer of 60 seconds
Hi, i am currently in the 3. project in the swift techdegree. In there i am told to make a countdown timer so that the user only has 60 seconds to complete his/her answer. I have never made it before, and as far as i know it hasnt been shown in the videos yet. Therefore i took my search to Google, but with very little result. i have found som NSTimer code examples that doesnt work, and tried different things for a couple of days now.
with no luck so far, i really hope that one of you guys can help me out a bit.
Thanks!! :)
4 Answers
Dan Lindsay
39,611 PointsNot sure if you got this figured out or not, but here is code for a working countdown timer that displays on a UILabel and is activated from a UIButton.
import UIKit
class ViewController: UIViewController {
@IBOutlet weak var timerLabel: UILabel!
var countdownTimer: Timer!
var totalTime = 60
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
}
func startTimer() {
countdownTimer = Timer.scheduledTimer(timeInterval: 1, target: self, selector: #selector(updateTime), userInfo: nil, repeats: true)
}
func updateTime() {
timerLabel.text = "\(timeFormatted(totalTime))"
if totalTime != 0 {
totalTime -= 1
} else {
endTimer()
}
}
func endTimer() {
countdownTimer.invalidate()
}
func timeFormatted(_ totalSeconds: Int) -> String {
let seconds: Int = totalSeconds % 60
let minutes: Int = (totalSeconds / 60) % 60
// let hours: Int = totalSeconds / 3600
return String(format: "%02d:%02d", minutes, seconds)
}
@IBAction func startTimerPressed(_ sender: UIButton) {
startTimer()
}
}
Hope this helps!
Dan
Dan Lindsay
39,611 PointsHello Daniel,
You will need to use the *Timer* class(it’s included in an import of UIKit), to create your countdownTimer variable. Timer has a function called *scheduledTimer* that you will use to initialize your countdownTimer. One parameter of *scheduledTimer* takes a selector, where you will select the method(a separate function that you create) to update your time. To end an instance of *Timer*, you can use the *invalidate()* method from the class. Also, since you are more than likely displaying your counter on a UILabel, you will have to format the time to a String that has the look you want. You will need another variable to hold the value you are starting your countdown from as well.
I didn’t want to just straight up give you all the code, but if you still can’t figure out how to set one up from the hints I have given, let me know and I will paste in my example of a countdown timer, as I have a basic working countdown timer figured out, and I will check on this thread throughout the day to see if you get it working. Let me know!
Dan
Dan Lindsay
39,611 PointsNo idea why this posted the way it did, should have been just text, as I didn't use any backticks. Weird.
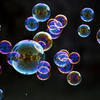
Jialong Zhang
9,821 Pointsthanks, great codes, Can you tell me how to calculate time in a millisecond? maybe round 3 decimal place.

Ivan Ivanov
iOS Development with Swift Techdegree Student 3,870 PointsThanks for the code. Was of great for me too. Just wanted to know whether you also have the error "Argument of '#selector' refers to instance method 'updateTime()' that is not exposed to Objective-C" which is solved by adding @objc in front of the function updateTime()?
Daniel Lambrecht
iOS Development Techdegree Student 5,302 PointsDaniel Lambrecht
iOS Development Techdegree Student 5,302 PointsThank you so much for everything i only had the chance to sit down and look at this now, i think i will give it a try without using your code firstly.
but its great that its there!
thanks again! :)