Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial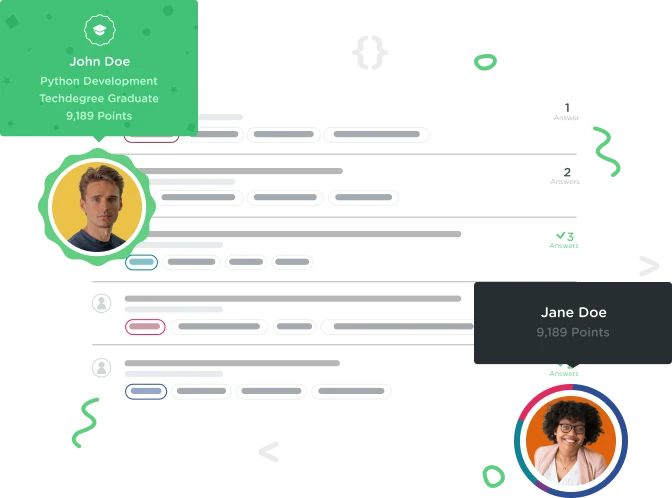
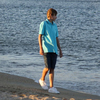
Tom Coomer
1,331 PointsSwift In-App Purchase
I am trying to add an In App Purchase to my app that I am writing in Swift.
I have the following code but I am unsure on how to create a purchase button:
let product_value = "co.uk.smudgeinc.reactiontimer.addgames"
override func viewDidLoad() {
super.viewDidLoad()
self.fetchAvailableProducts()
// Do any additional setup after loading the view.
}
func fetchAvailableProducts() {
let productID:NSSet = NSSet(object: self.product_value);
let productsRequest:SKProductsRequest = SKProductsRequest(productIdentifiers: productID);
productsRequest.delegate = self;
productsRequest.start();
}
func productsRequest (request: SKProductsRequest, didReceiveResponse response: SKProductsResponse) {
println("here!")
var count : Int = response.products.count
if (count>0) {
var validProducts = response.products
var validProduct: SKProduct = response.products[0] as SKProduct
if (validProduct.productIdentifier == self.product_value) {
println(validProduct.localizedTitle)
println(validProduct.localizedDescription)
println(validProduct.price)
} else {
println(validProduct.productIdentifier)
}
} else {
println("nothing")
}
}
func canMakePurchases() -> Bool
{
return SKPaymentQueue.canMakePayments()
}
func purchaseMyProduct(product: SKProduct) {
if (self.canMakePurchases()) {
println("Purchases are allowed ...")
var payment: SKPayment = SKPayment(product: product)
var defaultQueue: SKPaymentQueue = SKPaymentQueue ()
defaultQueue.addTransactionObserver(self)
defaultQueue.addPayment(payment)
} else {
println("Purchases are disabled in your device")
}
}
func paymentQueue(queue: SKPaymentQueue!, updatedTransactions transactions: [AnyObject]!) {
for transaction:AnyObject in transactions {
if let trans:SKPaymentTransaction = transaction as? SKPaymentTransaction{
switch trans.transactionState {
case .Purchased:
SKPaymentQueue.defaultQueue().finishTransaction(transaction as SKPaymentTransaction)
break;
case .Failed:
SKPaymentQueue.defaultQueue().finishTransaction(transaction as SKPaymentTransaction)
break;
// case .Restored:
//[self restoreTransaction:transaction];
default:
break;
}
}
}
}
//Button
@IBAction func purchase(sender: AnyObject) {
}
I have created the button but can not work out what I should put inside. Thnaks
1 Answer
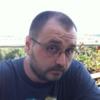
Robert Bojor
Courses Plus Student 29,439 PointsHi Tom,
I would say that if you have multiple products you should also have multiple buttons... Each button action should trigger the ** purchaseMyProduct** function with a product identifier.
I guess you can use different buttons that call the same IBAction and the difference is the tag of the button. Each tag should correspond to a product index inside an array. This way you can retrieve the product object and send it to the purchaseMyProduct function.
I'm still in the process of going through Swift myself so I'll be able to come back with some code once I'm done.