Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial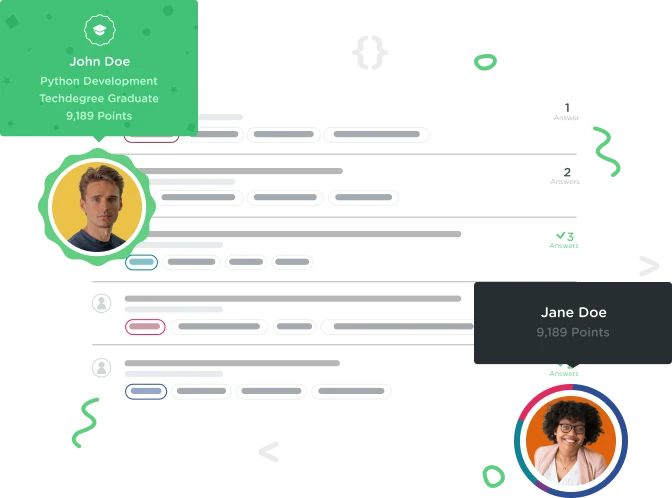
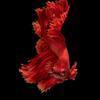
Michael Williams
Courses Plus Student 8,059 PointsSwift Inheritance: overriding func not working
Following are some errors from the code that follows. First, I understand what's going on with the last error: I'm returning something when it's expecting me to return nothing because it hasn't been defined. To fix it, I need to add a return value for the function I'm overriding. However, the question is why do I still have to have a return value that's been inherited? Shouldn't that be implied?
swift_lint.swift:40:19: error: method does not override any method from its superclass override func fullName(){
^ swift_lint.swift:29:8: note: potential overridden instance method 'fullName()' here func fullName() -> String {
^ swift_lint.swift:41:16: error: unexpected non-void return value in void function return "Dr. \(lastName)" ^~~~~~~~~~~~~~~~~
class Person {
let firstName: String
let lastName: String
init(firstName: String, lastName: String) {
self.firstName = firstName
self.lastName = lastName
}
func fullName() -> String {
return "\(firstName) \(lastName)"
}
}
// Enter your code below
class Doctor: Person {
override init(firstName: String, lastName: String) {
super.init(firstName: firstName, lastName: lastName)
}
override func fullName() String {
return "Dr. \(lastName)"
}
}
let someDoctor = Doctor(firstName: "Turd", lastName: "Ferguson")
2 Answers
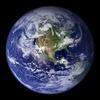
tromben98
13,273 PointsHi Michael!
If you look through the function you can notice that you forgot to mark the return type with the return arrow :
->
class Person {
let firstName: String
let lastName: String
init(firstName: String, lastName: String) {
self.firstName = firstName
self.lastName = lastName
}
func fullName() -> String {
return "\(firstName) \(lastName)"
}
}
// Enter your code below
class Doctor: Person {
override init(firstName: String, lastName: String) {
super.init(firstName: firstName, lastName: lastName)
}
// Look here!!!!!!!!!!
override func fullName() -> String {
return "Dr. \(lastName)"
}
}
let someDoctor = Doctor(firstName: "Turd", lastName: "Ferguson")
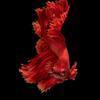
Michael Williams
Courses Plus Student 8,059 PointsHi there. Thanks for your help. I understand that the return portion is missing, but my question is why does it have to be there if we're inheriting it from the superclass? Because writing out the return type is repeating code.

Jeffrey Hallett
Courses Plus Student 1,619 PointsI am pretty sure this is because you need to print the "Dr."