Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial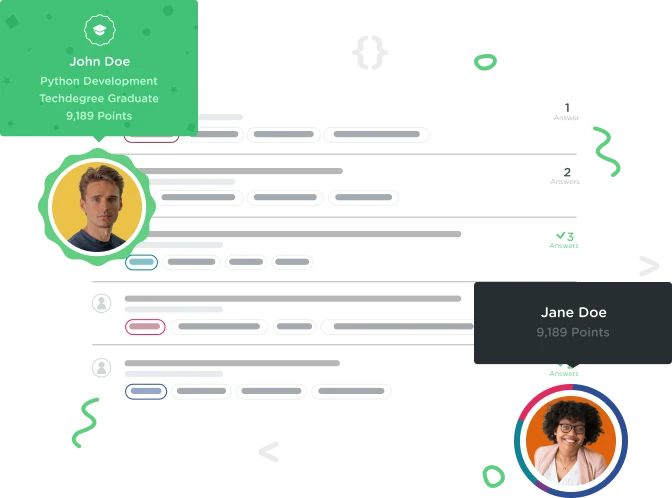

Luke Bearden
15,597 PointsSwift: Parameters and Tuples Extra Credit - Solution?
The task: Write a function that accepts a Dictionary as parameter and returns a named tuple. The dictionary should contain the following keys: title, artist and album.
I was able to get it working without any errors using the code below, but I'm not really sure what's going on, especially why the ! is needed. Any explanations or other solutions out there?
import UIKit
let favoriteSong = [
"title": "Haiti",
"artist": "Arcade Fire",
"album": "Funeral"
]
func returnTuple(songDict: [String: String]) -> (title: String, artist: String, album: String) {
return (songDict["title"]!, songDict["artist"]!, songDict["album"]!)
}
let song = returnTuple(favoriteSong)
song.title
song.artist
song.album
6 Answers

Thomas Nilsen
14,957 PointsI'd rather write it like this:
func returnTuple(songDict: [String: String]) -> (title: String?, artist: String?, album: String?) {
return (songDict["title"], songDict["artist"], songDict["album"])
}
Because if you for some reason didn't have the dictionary as a constant, and removed say, the first key/value pair, this code would still run, while yours would crash, badly.
I'd also love to hear what someone with more experience would say: Amit Bijlani and Pasan Premaratne
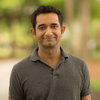
Amit Bijlani
Treehouse Guest TeacherA dictionary returns an optional which you will learn about in the next stage which should clear things up.
I would prefer Thomas Nilsen's solution because you never know when the value is going to be nil
. Keeping the optional makes it safe.

John Stephanites
2,007 PointsI read that you do not need the [String: String] because it is inferred. Is it better to actually put it in here?
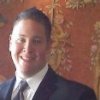
Brian Finkelstein
1,767 PointsHi All,
Sorry in advance if this is a noob question but after some research and testing of this on my own I still can't grasp this concept.
Can someone please explain in the simplest way possible how songDict: [String: String] is the correct syntax for passing returnTuple() the dictionary?
I haven't reached the optionals stage yet (most likely will tomorrow) but was curious the optimal way to solve this without using them as this was extra credit for the tuples stage. Thanks in advance!

Brock Ormond
7,176 Pointsas for the syntax, it kinda works like this:
nameOfTheDictionaryWhileInTheFunc: [keyDataType: valuePairDataType]
the portion in brackets tells the compiler that the value being passed a dictionary with a specific structure. All entries in the problem above are Strings in this case so it's [String:String]
Another example:
let cardRank = { 1: "Ace", 2:"Two", . . . 11:"Jack", 12:"Queen", 13:"King" }
sending this as a parameter requires something like this:
func pullingRank(rankDictionary:[Int, String]) {}
the keyDataType here is an Int (1...13), the valuePairDataType is String ("Ace", "Jack", etc)
When passing arrays or dictionary in Swift func as parameters, it needs to know the 'structure' of the dictionary that it is working with.
As for the optionals, that's probably the best way. Better to return nil that code breaking flat out after all.
Hope that answered your question, Brain.

Jonathan Mitchell
1,209 PointsWhat is the purpose of having the "!" in the return statement?

John Stephanites
2,007 PointsThese extra credits are very hard for me. Should I be going back or keep moving forward? Am I missing something and should know this?

Jerome Doyle
8,855 Pointsjust following along you will understand how it works in the future.
programming skills from doing and reviewing.
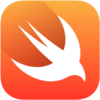
Antonija Pek
5,819 PointsPlease, somebody explain to me every word and symbol in this:
func returnTuple(yourDictionaryWithSongs: [String: String]) -> (title: String?, artist: String?, album: String?) {}
Brock Ormond
7,176 PointsBrock Ormond
7,176 PointsWhat if the Dictionary had different types of values within it (meaning not every line in the dictionary was a [String:String] format)?
Say that favoriteSong also had: "year": 2001, "sale price": 19.99, "best seller that year": true
How would you pass that dictionary in as an argument? I get the key value should be the same data type; but that is not necessary true with the value.
Greg Neborsky
1,020 PointsGreg Neborsky
1,020 PointsWhy we need "?" in "String?" I don't understand.
Brock Ormond
7,176 PointsBrock Ormond
7,176 PointsThe '?' added to a datatype (ex String?) changes that datatype into an optional, it will have either data of that type or nil. You add it to the return type to use it as an error catch; if it returns nil, then something went wrong; but prevents the app from crashing.
Greg Neborsky
1,020 PointsGreg Neborsky
1,020 PointsThanks! I've been impatient and asked before watched the next video, where Amit explained everything.
Frances Angulo
5,311 PointsFrances Angulo
5,311 PointsAt this stage in the course, how were we expected to come up with this solution? I have yet to be introduced, from what I recall, to concepts like:
-Utilizing a question mark in the tuple definitions -Referencing multiple items from a dictionary using the brackets -Possibly integrating the exclamation points after items
Did I miss a video? These concepts don't seem like 'stretch' learning because from what I can tell we haven't seen them at all?