Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial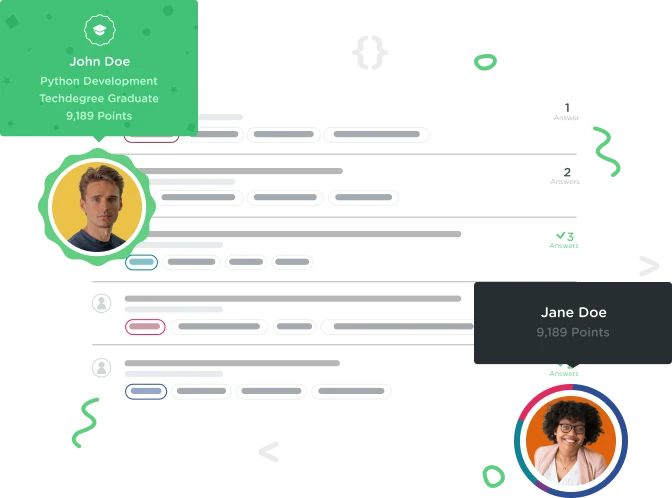
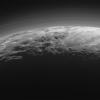
tremaine
2,645 PointsSwift Practice
I decided to practice my new Swift skills but am wondering as to why my code returns an optional.
This is what I came up with:
//: Swift Practice
import Cocoa
/*
Create a dictionary of teas and a dictionary of coffees available
Create a function to pass the name of the tea or coffee to check to the isCoffeeOrTeaAvailable function and iterate through coffee or tea dictionary to see if the coffee or tea is available and then return the name(value) back to the asking/calling function
Create a function to pass a number(Int) of ounces wanted to buy to the priceToCalculate to calculate the price
Create a function to call other functions created based on certain criteria
*/
////////////////////////////////////////////
//Dictionary of Teas and Coffees available//
////////////////////////////////////////////
let isTeaAvailable: [String: String] = [
"Breakfast": "Breakfast Tea",
"Lemon": "Lemon Tea",
"Blk": "Black Tea",
"Gin": "Ginger Tea"
]
let isCoffeeAvailable: [String: String] = [
"Col": "Coloumbia",
"Per": "Peru",
"Ken": "Kenya",
"Esp": "Espresso"
]
/////////////////////////////////////////////////////////
/// Function to interate of tea & coffee dictionaries ///
//////////// to check for availablity ///////////////////
/////////////////////////////////////////////////////////
func isCoffeeOrTeaAvailable(name: String) -> String {
if (isCoffeeAvailable[name] != nil){
return "\(isCoffeeAvailable[name])"
}else if (isTeaAvailable[name] != nil){
return "\(isTeaAvailable[name])"
}else {
return "This coffee or tea is NOT available"
}
}
/////////////////////////////////////////////////////////
///Function to caluculate price based on pass in ounces//
/////////////////////////////////////////////////////////
func priceToCalculate(ouncesWanted ounces: Int) -> Double {
// If 1oz = 1 * 2 - Only Tea
// If 2oz = 2 * 2 - Only Tea
// If 6oz = 6 * 2 - Only Coffee
// If 120z = 12 * 2 - Only Coffee
let price = 2.00
switch ounces{
case 1: return Double(ounces) * price
case 2: return Double(ounces) * price
case 6: return Double(ounces) * price
case 12: return Double(ounces) * price
default: return 0.00
}
}
/////////////////////////////////////////////////////
/// Function to call both isCoffeeOrTeaAvailable ////
///// and priceToCalculate to return both name //////
////////////// and price or neither /////////////////
/////////////////////////////////////////////////////
func priceOfTeaOrCoffee(nameOfTeaOrCoffeeToCheck name: String, ouncesToBuy: Int) -> (String) {
let nameOfTeaOrCoffee = isCoffeeOrTeaAvailable(name)
if nameOfTeaOrCoffee == "This coffee or tea is NOT available"{
return "This coffee or tea is NOT available"
}else if (isTeaAvailable[name] != nil) && ((ouncesToBuy == 1) || (ouncesToBuy == 2)){
return "\(nameOfTeaOrCoffee) costs \(priceToCalculate(ouncesWanted: ouncesToBuy)) "
}else if (isCoffeeAvailable[name] != nil) && (( ouncesToBuy == 6) || (ouncesToBuy == 12)){
return "\(nameOfTeaOrCoffee) costs \(priceToCalculate(ouncesWanted: ouncesToBuy))"
}else{
return "\(nameOfTeaOrCoffee) is NOT available in that size "
}
}
//////////////////////
/// Function Calls ///
//////////////////////
let priceOfSixOuncesOfEspresso = priceOfTeaOrCoffee(nameOfTeaOrCoffeeToCheck: "Esp", ouncesToBuy: 6)
let priceOfTwelveOuncesOfEspresso = priceOfTeaOrCoffee(nameOfTeaOrCoffeeToCheck: "Esp", ouncesToBuy: 12)
priceOfSixOuncesOfEspresso
I'm not sure why the code is coming out all one color, but that's not relevant. Why am I getting an Optional? Is there a video I need to re-review again? Thanks if you can help!
1 Answer
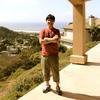
Richard Lu
20,185 PointsHey tremaine,
In swift, when we want to retrieve a value from a dictionary there is a chance that the key in that dictionary is not available. For example:
let aDictionary = [
"a": 1,
"b": 2,
"c", 3
]
let value = aDictionary["a"] // value is equaled to an optional Int
let value = aDictionary["d"] // value is equaled to nil
Remember, in order for a var/let to equal nil, it must be an optional value.
var something: Int? = nil // this is good
var somethingelse: Int = nil // the compiler will give you an error
In your case, you tried to retrieve a value from a dictionary in
func isCoffeeOrTeaAvailable(name: String) -> String {
if (isCoffeeAvailable[name] != nil){
return "\(isCoffeeAvailable[name])"
}else if (isTeaAvailable[name] != nil){
return "\(isTeaAvailable[name])"
}else {
return "This coffee or tea is NOT available"
}
}
In order to fix the method you could use if let unwrapping (optional binding), which I'm sure Pasan will introduce in a later video, below.
func isCoffeeOrTeaAvailable(name: String) -> String {
if let value = isCoffeeAvailable[name] {
return "\(value)"
}else if let value = isTeaAvailable[name] {
return "\(value)"
}else {
return "This coffee or tea is NOT available"
}
}
Hope this helps. Happy coding! :)
tremaine
2,645 Pointstremaine
2,645 PointsI'm sure Pasan will get to it too. Pasan is an excellent teacher. Thanks so much for your explanation Richard I'll test it out and practice with it. Cheers!