Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial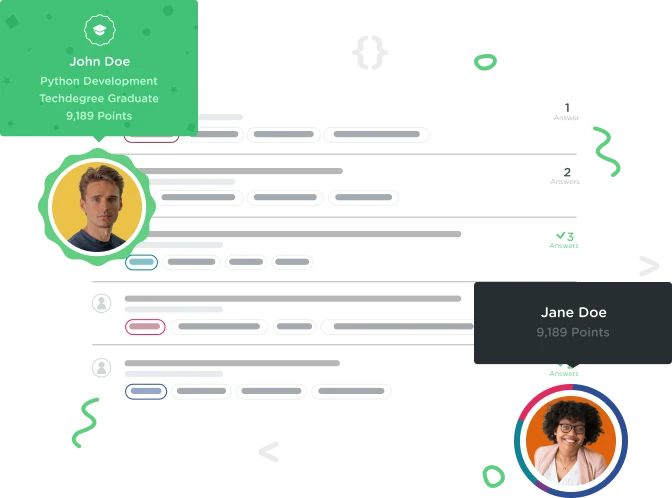
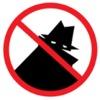
Anonymous Person
5,297 PointsSwift Protocol Challenge help?
I dont understand why my code wont be accepted, I have followed all the directions and I keep getting an error that my code needs brackets. After I add brackets, I get an error that my code has unnecessary brackets. Please help me
protocol UserType {
var name: String { get }
var age: Int { get set }
}
struct Person: UserType {
var name: String { get }
var age: Int { get set }
}
let somePerson = UserType()
2 Answers

Greg Kaleka
39,021 PointsHi AP,
In order to conform to the protocol UserType, you need to do two things:
- Declare that Person conforms to the protocol
- Include the properties and methods laid out in the protocol
You've done 1 by adding the : UserType
. For 2, all you have to do is declare the properties like this:
struct Person: UserType {
var name: String
var age: Int
}
Then, when creating an instance, you need to do the "memberwise initializer" that comes with Structs. You've seen this before. It looks like this:
let somePerson = Person(name: "Anonymous Person", age: 893)
In the protocol, the get and set declarations are just saying that by conforming to this protocol, name will be read only, while age will be read and write. If you wanted to, you could explicitly implement getter and setter methods in your Person struct, but it's not necessary, since Swift already has a way to do this (this is why things like dot notation and assignment "just work" - Swift is creating getter and setter methods under the hood).
Hope this helps!
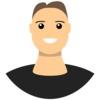
Samson Cohen
12,263 PointsHi there! Looks like you're on the right track, but maybe it would help if you gave your struct some data to work with.
Also keep in mind that you don't need to use { get } or { set } or anything like that in a struct. As far as I know, that syntax is normally just used within protocols.
Anyway, here's the code that I created for this challenge! Try it out:
protocol UserType {
var name: String { get }
var age: Int { get set }
}
struct Person: UserType {
var name = "Bill Smith"
var age = 24
}
let somePerson = Person()

Greg Kaleka
39,021 PointsHey Samson,
Your solution passes, but I'd encourage you to think about whether default values make sense when creating structs and classes. Sure I can use your Person struct and create any combination of names and ages I want, but what if I forget to add a name and age? 24 year old Bill Smith floating around, or would you rather have the compiler let you know you've left something out?
I know this is a trivial example for a code challenge, but as you do more coding, you'll often find that setting a default value is the easy solution to the compiler yelling at you about not having an initializer, or not being able to use a property somewhere, but it's often not the best solution. As a best practice, you should only set default values where it actually makes sense for the property to equal that value most of the time. For example, in a blogging app, you could have a publishedOn property that defaulted to the current time. Just some food for thought :).
Also, regarding getters and setters - you're definitely right that in this instance, there's no need to use them. However, just as an FYI, there are times when you'll use them, and that's for computed properties. Check out the Apple docs on this subject.
Happy coding!
-Greg