Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial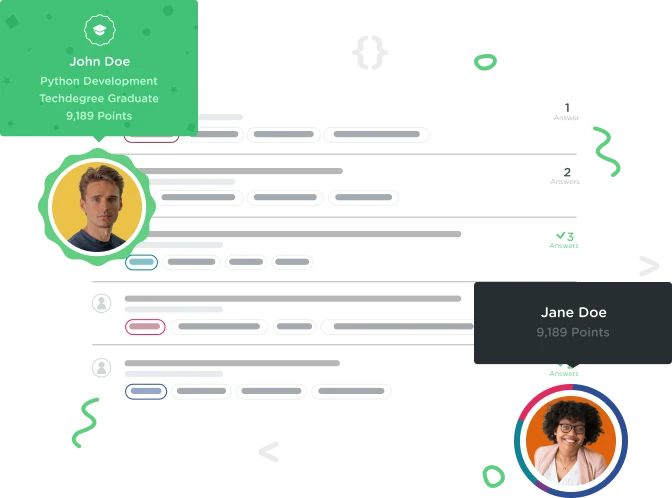

Austin Betzer
2,747 PointsSwift Recap part 1 swift 3.0
What am I doing wrong?
struct Tag {
let name: String
}
struct Post {
let title: String
let author : String
let tag: Tag
init(Title: String, Author: String, Tag: tag) {
self.Title = title
self.Author = author
self.Tag = tag
}
}
let firstPost = Post(title: "The Great", author: "author", tag: tag)
3 Answers

Giorgos Karyofyllis
21,276 Pointsstruct Tag {
let name: String
}
struct Post {
let title: String
let author: String
let tag: Tag
func description() -> String {
return "\(title) by \(author). Filed under \(tag.name)"
}
}
let firstPost = Post(title: "Title", author: "Author", tag: Tag(name: "tag"))
let postDescription = firstPost.description()
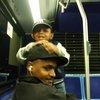
Xavier D
Courses Plus Student 5,840 PointsHi,
I haven't done this challenge in a .while, and you didn't post what exactly the challenge wanted from you. Luckily, I have my notes on it. So when I took this challenge, its notes said:
//Challenge Task 1 of 2
//
//In the editor you've been provided with a Tag type.
//Create a struct named Post with three stored properties:
//title of type String
//author of type String
//tag of type Tag
//
//Create an instance of Post and assign it to a constant named firstPost.
It appears that you were unsuccessful in creating a Post instance because you did not give a proper value for the struct's 3rd parameter when you tried to assign and instance of it to the firstPost constant. The improper value is 'tag', and the parameter want's an argument that's of a "Tag" type. Thus it want's a value that inherits from your struct called "Tag" . The value that you gave called "tag" is not mentioned anywhere in your code. So to mention it, create an instance of your Tag struct and assign that instance to a constant called 'tag', and should be good from there. Remember that your Tag instance will want a string for it's argument.
Also, that initializer written in your Post object is not necessary and may confuse things--structs already come with a member-wise one for so that init can go.
Heads up for the second part. I think the challenge will want you to write a method called "description" that returns a string, which should be placed within your Post object--this function will also not be taking in arguments.
Hope this helps!

Jaroslaw Adamowicz
11,634 PointsHi,
first thing is you should use lowercase for members of struct, so you have:
init(Title: String, Author: String, Tag: tag) {
self.Title = title
self.Author = author
self.Tag = tag
}
but you should have:
init(Title: String, Author: String, Tag: tag) {
self.title = title
self.author = author
self.tag = tag
}
Second thing is you cannot just use tag, you have to use Tag(name:), so you have :
let firstPost = Post(title: "The Great", author: "author", tag: tag)
while you should have :
let firstPost = Post(title: "The Great", author: "author", tag: Tag(name:"someTag"))
Third thing is you don't need init method at all, because you already have defult implicit one (every struct has), so this :
let firstPost = Post(title: "Title", author: "Author", tag: Tag(name: "tag"))
will work with your current method or without it. And that's what really matters in this excercise.
Cheers!