Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial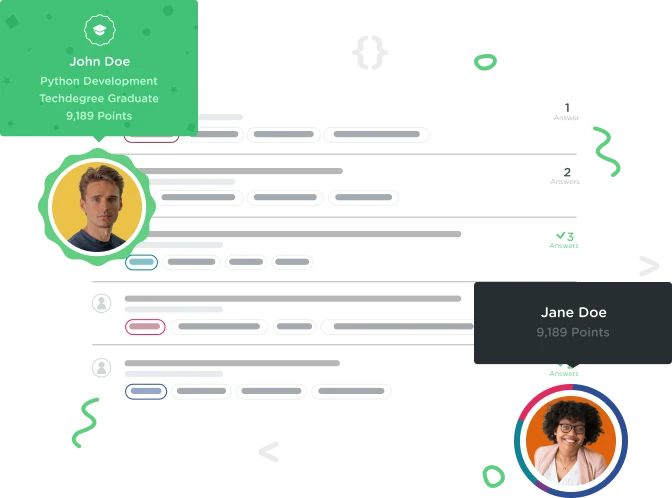
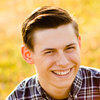
Caleb Kleveter
Treehouse Moderator 37,862 PointsSwift re-cap part 2.
Can someone explain this challenge to me? I am thoroughly confused over it. Great job Pasan :).
Here are the instructions:
In the editor you've been provided with two classes - Point to represent a coordinate point and Machine. The machine has a move method that doesn't do anything because most machines are motionless.
Your task is to subclass Machine and create a new class named Robot. In the Robot class, override the move method and provide the following implementation. If you enter the string "Up" the y coordinate of the Robot's location increases by 1. "Down" decreases it by 1. If you enter "Left", the x coordinate of the location property decreases by 1 while "Right" increases it by 1.
Note: If you use a switch statement you can use the break statement in the default clause to exit the current iteration.
class Point {
var x: Int
var y: Int
init(x: Int, y: Int){
self.x = x
self.y = y
}
}
class Machine {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(direction: String) {
print("Do nothing! I'm a machine!")
}
}
// Enter your code below
Here is the error I got:
swift_lint.swift:31:12: error: binary operator '~=' cannot be applied to operands of type 'String' and 'Point'
case "Up": y += 1
^~~~
swift_lint.swift:31:18: error: use of unresolved identifier 'y'
case "Up": y += 1
^
swift_lint.swift:32:12: error: binary operator '~=' cannot be applied to operands of type 'String' and 'Point'
case "Down": y -= 1
^~~~~~
swift_lint.swift:32:20: error: use of unresolved identifier 'y'
case "Down": y -= 1
^
swift_lint.swift:33:12: error: binary operator '~=' cannot be applied to operands of type 'String' and 'Point'
case "Left": x -= 1
^~~~~~
swift_lint.swift:33:20: error: use of unresolved identifier 'x'
case "Left": x -= 1
^
swift_lint.swift:34:12: error: binary operator '~=' cannot be applied to operands of type 'String' and 'Point'
case "Right": x += 1
^~~~~~~
swift_lint.swift:34:21: error: use of unresolved identifier 'x'
case "Right": x += 1
^
This is the code:
class Robot: Machine {
func move() {
switch location {
case "Up": y += 1
case "Down": y -= 1
case "Left": x -= 1
case "Right": x += 1
default: break
}
}
}
9 Answers

Chase Marchione
155,055 PointsFully accounting for:
- The overriding of the Machine class's move function (with the direction String as a parameter.)
- The switch statement being named for the data we are investigating (the direction String data), rather than location.
- x and y being of the location Point.
class Robot: Machine {
override func move(direction: String) {
switch direction {
case "Up": location.y += 1
case "Down": location.y -= 1
case "Left": location.x -= 1
case "Right": location.x += 1
default: break
}
}
}
Hope this helps!
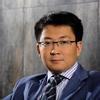
Andrei Li
34,475 PointsHere is my solution:
class Point {
var x: Int
var y: Int
init(x: Int, y: Int) {
self.x = x
self.y = y
}
}
class Machine {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(_ direction: String) {
print("Do nothing! I am a machine!")
}
}
// Enter your code below
class Robot: Machine {
override func move(_ direction: String) {
switch direction {
case "Up": return location.y += 1
case "Down": return location.y -= 1
case "Left": return location.x -= 1
case "Right": return location.x += 1
default: break
}
}
}
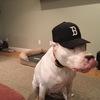
Robert Cadorette
9,275 PointsCan someone explain why there is no init - super init needed with creating the Robot sub-class? Thank you

Jeffrey Rosenthal
4,539 PointsYou want to create a new class, Robot, that inherits from the Machine class and overrides the move method that comes with the Machine class.
so basically:
class Robot: Machine {
override func move() {
//whatever
}
}
check out the following link for some clear examples of subclassing and overriding: https://developer.apple.com/library/ios/documentation/Swift/Conceptual/Swift_Programming_Language/Inheritance.html
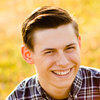
Caleb Kleveter
Treehouse Moderator 37,862 PointsI added to my post.

Jeffrey Rosenthal
4,539 PointsMy immediate suspicion is scoping issues. Try self.x in the switch case.
Almost sure
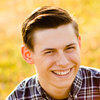
Caleb Kleveter
Treehouse Moderator 37,862 PointsDidn't work. You can test it in the challenge.

Martin Granger
14,521 PointsI see a couple of things that should help you. Make sure you properly override the move function:
func override move(direction: String) {
}
Your switch control expression should be "direction" rather than "location" since "Up, Down, Right, and Left" are the directions.
Also try location.y instead of just y.
Hopefully this helps you.
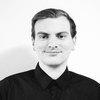
Christoph Hellmuth
11,604 Pointsfunc override move(direction: String) { }
Didn't work for me but: override func move(direction: String) {} did.
Also thank you for the direction tip.

Alexander Tucker
Courses Plus Student 1,262 PointsRobert Cadorette thats a good point
i think there need to be some changes to the given code as well as the new
-------------given code needs-------------
class Machine {
var location: Point
// var direction: String ///
init() {
self.location = Point(x: 0, y: 0)
// not sure what code would in be used here//
}
func move(_ direction: String) {
print("Do nothing! I am a machine!")
}
}
--------------------new code--------------------
/// the code need to do///
///direction = location(move)///
/// for example "Up" = (location(x: 0, y: 0) + y: 1)///
/// the switch case would give the value the class would change to///
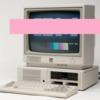
kols
27,007 PointsUsing a switch statement is probably a good way to go for this challenge; however, I'm providing an alternative answer below for those wanting to solve this using an 'if' statement:
class Point {
var x: Int
var y: Int
init(x: Int, y: Int) {
self.x = x
self.y = y
}
}
class Machine {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(_ direction: String) {
print("Do nothing! I am a machine!")
}
}
class Robot: Machine {
override func move(_ direction: String) {
if direction == "Up" {
self.location.y += 1
} else if direction == "Down" {
self.location.y -= 1
} else if direction == "Left" {
self.location.x -= 1
} else if direction == "Right" {
self.location.x += 1
} else {
self.location = location
}
}
}

alexander88
10,824 PointsRandom question, why is it that when I put
''' case "Up": y += 1 '''
versus
''' case "Up": location.y += 1'''
The first fails while the second succeeds? is it related to scope? The only reasoning I can really piece together is that x and y exist as variables within point, but inside the class Machine they only exist within location and Point, therefore they cannot be altered as individual variables. However, if we tried to alter them within the class Point, or simply outside any sort of object it would work? Trying to get a handle on scope and classes in general, any explanation or help is appreciated. Thanks in advanced.
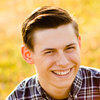
Caleb Kleveter
Treehouse Moderator 37,862 PointsHey Alexander, this should be posted as a new question instead of as an answer. You'll have a better chance of getting an answer and it keeps this thread clean. Thanks!
Christoph Hellmuth
11,604 PointsChristoph Hellmuth
11,604 PointsGood job :) Was literally coming to the same solution just 5 minutes before you posted it. Took me a while but was worth the learnings.