Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial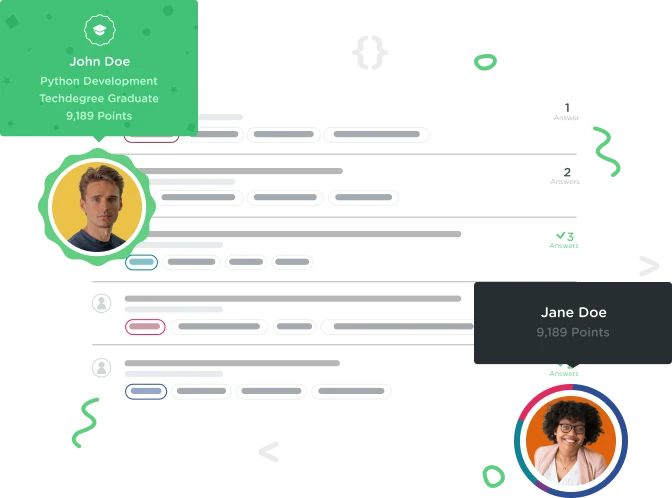
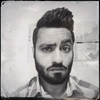
Mahesh Patel
3,376 PointsSwift tuples challenge task 2 of 3
what am I doing wrong here?
func greeting(person: String) -> (greeting: String, language: String) { let language = "English" let greeting = "Hello (person)"
return (greeting, language)
}
var result = greeting(person: "Tom")
2 Answers

Stone Preston
42,016 Pointsremove the argument name from the greeting function call
func greeting(person: String) -> (greeting: String, language: String) {
let language = "English"
let greeting = "Hello \(person)"
return (greeting, language)
}
var result = greeting("Tom")
If you want to give your parameters external names to be used in the function call you will have to use external parameters.
func greeting(#person: String) -> (greeting: String, language: String) {
let language = "English"
let greeting = "Hello \(person)"
return (greeting, language)
}
var result = greeting(person: "Tom")
You can use both regular and shorthand notation.
below is an excerpt on external parameters from the swift eBook:
if you want users of your function to provide parameter names when they call your function, define an external parameter name for each parameter, in addition to the local parameter name. You write an external parameter name before the local parameter name it supports, separated by a space:
func someFunction(externalParameterName localParameterName: Int) {
// function body goes here, and can use localParameterName
// to refer to the argument value for that parameter
}
below is an excerpt on shorthand external parameter names from the swift eBook.:
If you want to provide an external parameter name for a function parameter, and the local parameter name is already an appropriate name to use, you do not need to write the same name twice for that parameter. Instead, write the name once, and prefix the name with a hash symbol (#). This tells Swift to use that name as both the local parameter name and the external parameter name.
This example defines a function called containsCharacter, which defines external parameter names for both of its parameters by placing a hash symbol before their local parameter names:
func containsCharacter(#string: String, #characterToFind: Character) -> Bool {
for character in string {
if character == characterToFind {
return true
}
}
return false
}
This function’s choice of parameter names makes for a clear, readable function body, while also enabling the function to be called without ambiguity:
let containsAVee = containsCharacter(string: "aardvark", characterToFind: "v")
// containsAVee equals true, because "aardvark" contains a "v"

Jack Yi
8,450 PointsI believe, the hash symbol (#) for doubling up external and local parameters has been removed from the latest version of swift, you'll have to name them specifically now (i.e. string string).