Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial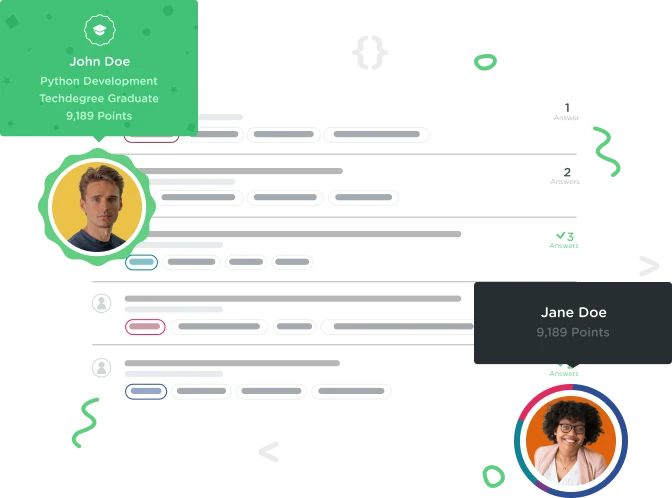

Razvan Zamfir
1,282 PointsSwitch between hover and click events, depending on window width.
I have made an HTML5 responsive, 2 levels menu you can see at http://jsfiddle.net/Lsam4k3L/17/ The wide screen version shows submenus upon hovering over the main menu items; the narrow screen (less then 768px) version shows submenus upon clicking the main menu items.
The problem is that, if I resize the window, the switch between hover and click events does not happen properly.
What shall I change in my script? Or is there an already made solution that I can use?
Thank you!
1 Answer

Jeff Lemay
14,268 PointsYou could detect whenever the screensize changes and call your functions based on whether you're above or below a certain screensize:
$(window).resize(function() {
if ($(window).width() < 960) {
// use click events
}
else {
// use hover events
}
});
This is a simple example, you might want to add some functionality to detect when the user finishes resizing the screen so you aren't making function calls after every pixel increase/decrease. http://stackoverflow.com/questions/4298612/jquery-how-to-call-resize-event-only-once-its-finished-resizing
Razvan Zamfir
1,282 PointsRazvan Zamfir
1,282 PointsHow would the code above look if I wanted to make sure the events will be used by the browser after the resizeing is finished and according to the window width resulting from the resize?
Jeff Lemay
14,268 PointsJeff Lemay
14,268 PointsThere's a fiddle with an example in the link I posted above.