Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial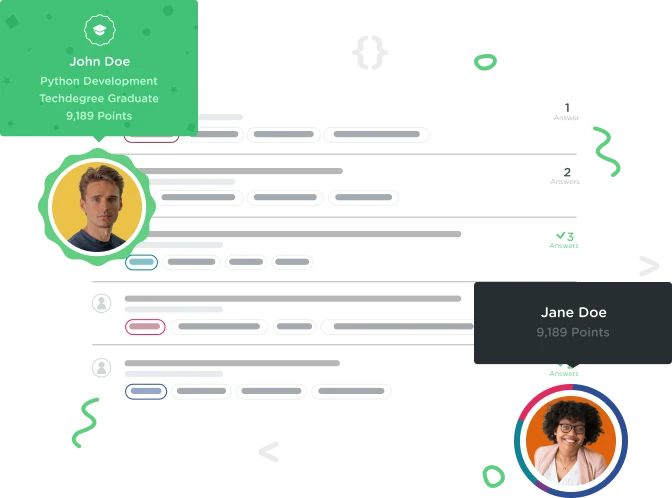
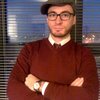
Frank D
14,042 PointsSwitch Case Condition?
In this video Hampton Paulk mentioned about going to explain the Switch Case Condition. Where is this explained? Am i missing something?
Thank you in advance! Frank
5 Answers
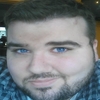
Marcus Parsons
15,719 PointsHey Frank,
I heard him mention it in the video, but he doesn't go over it. But no worries, I'll help you out here!
I'm not sure if you've ever encountered switch statements but they are easy to get a grasp on. The switch conditional starts off looking very closely like an if statement.
<?php
switch ($variableName) {
//Code here
}
?>
What switch does is look for cases of a variable you pass into it. Let's say for example we have a variable named $name
and we want to do something different for each name that might be passed into $name
.
So, with that in mind we will start by setting $name
to be some name like 'Frank' and then start switch off with that $name
variable:
<?php
$name = 'Frank';
switch ($name) {
//Code here
}
?>
Now, what we want to do is to define cases for what $name
could be. Let's say we we want to do something specific with 3 names: Frank, Marcus, or Bobbert. We define these specific cases inside of the switch conditional. We should also add a default
case for any other value that $name might be that isn't listed in the cases.
And, we add a break
statement right after each case (for right now) because each case must have a break
command attached to it. The break
command prevents switch from going on to the next case and executing code:
<?php
$name = 'Frank';
switch ($name) {
//Specific action if $name is Frank
//Also notice the colon : after each case
case 'Frank':
break;
//Specific action if $name is Marcus
case 'Marcus':
break;
//Specific action if $name is Bobbert
case 'Bobbert':
break;
//Action if $name is anything else not listed in cases
default:
break;
}
?>
Next, we'll add some code so that we do something for each case. For Frank and Marcus we'll echo out "You're awesome!". For Bobbert, we'll echo out "You smell terrible!". For everyone else, we'll echo out "I don't know you! :O"
<?php
$name = 'Frank';
switch ($name) {
case 'Frank':
echo "You're awesome!";
break;
case 'Marcus':
echo "You're awesome!";
break;
case 'Bobbert':
echo "You smell terrible!";
break;
default:
echo "I don't know you! :O";
break;
}
?>
Now, when you run this on your PHP page, PHP will see that the name is "Frank" and will echo out "You're awesome!"
Let's say you put another name in there, like we do below, that isn't listed in the cases, it will echo that default statement "I don't know you! :O"
<?php
$name = 'Bartleby';
switch ($name) {
case 'Frank':
echo "You're awesome!";
break;
case 'Marcus':
echo "You're awesome!";
break;
case 'Bobbert':
echo "You smell terrible!";
break;
default:
echo "I don't know you! :O";
break;
}
?>
Does that make sense?
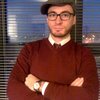
Frank D
14,042 PointsThanks Marcus for this brilliant explanation!! You are awesome ;-) However, it seems to me that the Switch Case condition is similar to the if/elseif/else condition but in addition we can have much more different "cases" to check/switch, right?
Can we "switch case", for instance, through an array as the if condition does? If yes, how could be applied on this example?
<?php
$name = array(
'Marcus' => 'Awesome',
'Frank' => 'Awesome',
'Bobbert' => 'Terrible',
);
?>
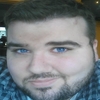
Marcus Parsons
15,719 PointsWhat I would do would be to iterate over each name contained and then get each key and value from the array. In this case, we want to use switch on the key and echo out the value.
<?php
$name = array(
'Marcus' => 'Awesome',
'Frank' => 'Awesome',
'Bobbert' => 'Terrible',
);
//$firstname is key and $feeling is the value
foreach($name as $firstname => $feeling) {
switch ($firstname) {
case "Frank":
case "Marcus":
case "Bobbert":
//Echo out the value from the array, so we're awesome
//and Bobbert is terrible of course! :D
echo $feeling;
break;
default:
echo "I don't know you! :O";
break;
}
}
?>
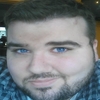
Marcus Parsons
15,719 PointsOh, thank you, by the way! We're awesome! :D And to answer your question, yes, it is very much like an if/else-if/else long conditional but it can save quite a bit of physical memory by having you type less on to the page (and saves your cramped fingers)!
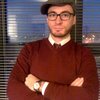
Frank D
14,042 PointsI've tried that with this option: $name = 'Frank'; and I got this result:
AwesomeAwesomeTerrible
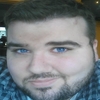
Marcus Parsons
15,719 PointsIn your question, $name is an array with 3 keys and 3 values, not just 'Frank'. What I did was placed a foreach loop outside of the switch statement that goes over each key and value in the array $name. Each iteration of the loop results in the key it is on being mapped to $firstname and the value being mapped to $feeling.
So, in those cases, each of those specific names are located as key values in the array. I specified those 3 cases within the switch to echo out the $feeling (value) that would correspond with them. So, for Frank it was "Awesome". For Marcus, it is "Awesome". And finally Bobbert is "Terrible". They are clustered together in the code because each of those are going to have a value associated with them and there is no reason to separate them when each one of them would echo out the value associated with it.
The reason why they are clustered together on the page is that there is no HTML formatting done to them. A simple formatting measure would be to add a block level element that contains the $feeling text:
<?php
$name = array(
'Marcus' => 'Awesome',
'Frank' => 'Awesome',
'Bobbert' => 'Terrible',
);
//$firstname is key and $feeling is the value
foreach($name as $firstname => $feeling) {
switch ($firstname) {
case "Frank":
case "Marcus":
case "Bobbert":
//Echo out the value from the array, so we're awesome
//and Bobbert is terrible of course! :D
echo "<p>".$feeling."</p>";
break;
default:
echo "I don't know you! :O";
break;
}
}
?>
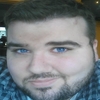
Marcus Parsons
15,719 PointsBy the way, the best way you can look at cases being used in the way above is to look at them as "or" statements in an if conditional and the default as the final "else" statement.
<?php
$name = array(
'Marcus' => 'Awesome',
'Frank' => 'Awesome',
'Bobbert' => 'Terrible',
);
//$firstname is key and $feeling is the value
foreach($name as $firstname => $feeling) {
//same as clustering cases in switch statement
if ($firstname == "Frank" || $firstname == "Marcus" || $firstname == "Bobbert") {
//Echo out the value from the array, so we're awesome
//and Bobbert is terrible of course! :D
echo "<p>".$feeling."</p>";
}
//Same as default case in switch statement
else {
echo "I don't know you! :O";
}
}
?>
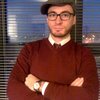
Frank D
14,042 Pointsyeah, I agree, that seems the best option so far.
I was thinking more something about this though:
<?php
$name = array(
'Marcus' => 'Awesome',
'Frank' => 'Awesome',
'Bobbert' => 'Terrible',
);
foreach($name as $firstname => $feeling) {
switch ($firstname) {
case 'Marcus' == 'Awesome':
case 'Frank' == 'Awesome':
case 'Bobbert' == 'Terrible':
//Echo out the correct combination (key/value) from the array
echo 'You won the Game!';
break;
case 'Marcus' == 'Terrible':
case 'Frank' == 'Terrible':
case 'Bobbert' == 'Awesome':
//Echo out the all wrong combinations (key/value) from the array
echo 'Wrong answers, please try again!!';
break;
case 'Marcus' == 'Awesome':
case 'Frank' == 'Terrible':
case 'Bobbert' == 'Awesome':
//Echo out the 2 wrong combinations and 1 right (key/value) from the array
echo '2 Wrong answers, you can do better, please try again!!';
break;
case 'Marcus' == 'Awesome':
case 'Frank' == 'Awesome':
case 'Bobbert' == 'Awesome':
//Echo out the 1 wrong combination and 2 right (key/value) from the array
echo 'Only 1 Wrong answers, you are close to win the game!!';
break;
default:
echo "Are you serious?!! :O";
break;
}
}
?>
is that possible this way?
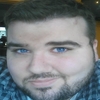
Marcus Parsons
15,719 PointsUnfortunately, you can't use a switch statement that way because you can't use comparison operators in cases. They are for specific values of the thing being switched.
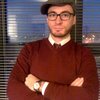
Frank D
14,042 PointsThat's clear! Thank you very much! very helpful!! ;)
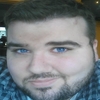
Marcus Parsons
15,719 PointsIf you would like to go ahead and select a best answer, we can get this resolved. :) You're very welcome! Any more questions, don't hesitate to come back and ask, but it will likely be in the morning as Sleepy Town in Lala Land is calling my name!
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsAnother reason why you use
break
statements is that you can pair cases together when two or more cases do the same thing (such as our code which both just echo out the same exact thing). All you have to do is put the first case, a colon, and then the second case, colon (same for any other cases that you wish to add) and the statements to be executed followed by abreak
as usual: