Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial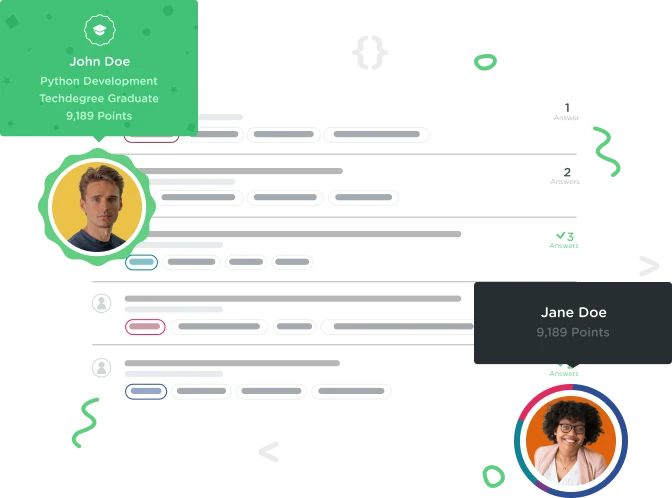

Sebastian Röder
13,878 PointsSwitch Statement to select camera option relies on fall-through behavior
The following code is given to find the menu item that was clicked by the user:
@Override
public boolean onOptionsItemSelected(MenuItem item) {
int itemId = item.getItemId();
switch(itemId) {
case R.id.action_logout:
ParseUser.logOut();
navigateToLogin();
case R.id.action_edit_friends:
Intent intent = new Intent(this, EditFriendsActivity.class);
startActivity(intent);
case R.id.action_camera:
AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setItems(R.array.camera_choices, mDialogListener);
AlertDialog dialog = builder.create();
dialog.show();
}
return super.onOptionsItemSelected(item);
}
I find it confusing that there are no break
or return
statements in the cases of the switch statement. I am also wondering why there is no default
case and the return super.onOptionsItemSelected(item);
is put after the whole switch
statement.
In this form the proper functioning of the switch
statement relies entirely on the fall-through behavior of Java's switch
statement. As far as I know this was never explained in any Treehouse course and it is generally confusing. If you change the order of the cases in this example, it will show unintended side-effects: for example if you put the edit friends case first and the logout case second, tapping the Edit friends button will also logout the current user.
It would be nice if this topic could be cleared up a little, either by changing the code or by explicitly mentioning that the fall-through behavior is used here (although in my opinion this code is a little too clever).
Here is how I wrote the method instead:
@Override
public boolean onOptionsItemSelected(MenuItem item) {
switch (item.getItemId()) {
case R.id.action_edit_friends:
navigateToEditFriendsActivity();
return super.onOptionsItemSelected(item);
case R.id.action_logout:
String username = ParseUser.getCurrentUser().getUsername();
ParseUser.logOut();
Log.i(TAG, String.format("User %s is logged out", username));
navigateToLoginActivity();
return super.onOptionsItemSelected(item);
case R.id.action_camera:
AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setTitle("Camera Options")
.setItems(R.array.camera_options, null)
.create()
.show();
return super.onOptionsItemSelected(item);
default:
return super.onOptionsItemSelected(item);
}
}
Another alternative would be to use break
in each case and keep the return
after the switch
statement.
Which version do you prefer and why?
1 Answer

Stone Preston
42,016 Pointslooking at this post Ben says he forgot to add the break statements in one of the videos. Im not sure which video he is referring to though. I would just add the break statements and keep the return at the end. Using break seems to be pretty standard as opposed to just returning after each case
Looks like it does not mention anything about this in the teachers notes for the video Presenting a list of choices video. Maybe Ben Jakuben can add some
Sebastian Röder
13,878 PointsSebastian Röder
13,878 PointsThanks for the feedback. I agree that the use of break statements might be the best solution in this case because it eliminates the code duplication in my returns.
Ben Jakuben
Treehouse TeacherBen Jakuben
Treehouse TeacherSorry about that! I do add the break statements in when we revisit this code. Stone Preston, I'll go add a Teacher's Note now. :)
Sebastian Röder
13,878 PointsSebastian Röder
13,878 PointsThanks Ben Jakuben.