Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial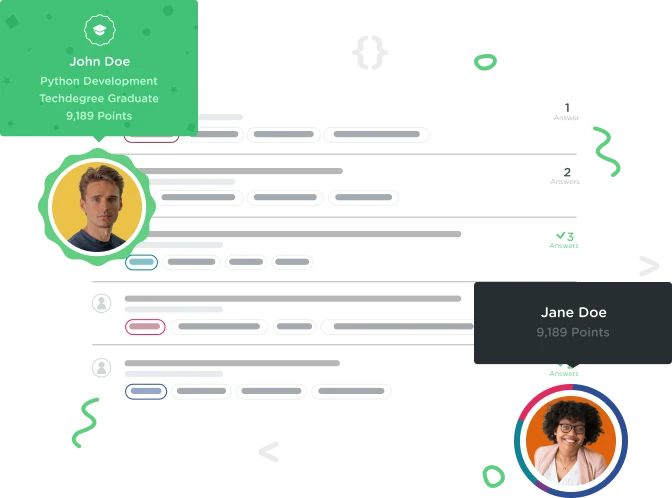

Stephen Igwebuike
805 PointsSwitch statements challenge
My problem with this challenge is converting the second if statement into a switch statement. It's NOT equal routine, so how do I change it to a switch statement.
<?php
//Available roles: admin, editor, author, subscriber
if (!isset($role)) {
$role = 'subscriber';
}
//change to switch statement
if ($role != 'admin') {
echo "You do not have access to this page. Please contact your administrator.";
}
1 Answer

Collin Halliday
Full Stack JavaScript Techdegree Student 17,491 PointsHey Stephen,
Switch statements can, in some cases, be used in place of a series of if statements, usually when you want to compare the same variable (or expression) with multiple values. In this case, the variable we want to compare with multiple values is the $role variable. The values with which we wish to compare our $role variable are: admin, editor, author, subscriber.
The switch statement makes use of case statements to accomplish these comparisons. The variable we wish to compare with each case statement's value or expression is passed as an argument to the switch statement. The switch statement then compares the argument's value with that of each case statement until it finds a match. Upon a match, the code in the case statement will run,Β and the switch statement will continue until the end of the statement or until it encounters a break statement.
If you want a certain piece of code to run in the event there are no matches with any of your case statements, you can include a default statement. In this case, where $role does not equal "admin", we can use a default statement to echo to the user the message, "You do not have access to this page. Please contact your administrator."
If the value of the $role variable does match "admin", however, we can set up our switch statement to echo the phrase, "As an admin, you can add, edit, or delete any post."
The code should look something like the following, at least for the first part of the challenge:
switch($role) { case "admin": echo "As an admin, you can add, edit, or delete any post."; break; default: echo "You do not have access to this page. Please contact your administrator."; break; }
Even though the switch statement does not make use of comparison operators, under the hood, it is still making comparisons between values.
I hope that is somewhat helpful. Best of luck!