Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial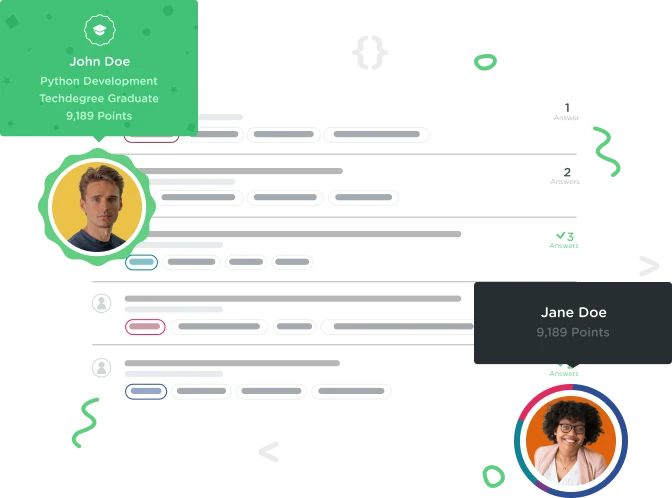

Alexander Estefanous
Courses Plus Student 3,579 PointsSwitch statements Help
Hi all,
I've just started out learning how to use swift, its all a bit confusing but I am enjoying the complexity of it.
however, I'm slightly stuck on this FizzBuzz Problem. I've tried to do it myself and this is as far as I've got:
for n in 1...100 { switch n { case 1: n%3 == 0; print("Fizz"); case 2: n%5 == 0; print("Buzz"); case 3: n%3 == 0 && n%5 == 0; print("FizzBuzz"); default: print("n") } }
my code doesn't seem to work. I've gone through the solution video, and the code is similar to mine. I understand it but would love to see where I went wrong, and if I'm grasping the switch statements.
Thank you so much for your time!
Best,
Alex
3 Answers
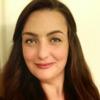
Jennifer Nordell
Treehouse TeacherHi there! I'm just going to give a hint here. Your logic is a little off in your switch statement. It needs to set the most restrictive condition first. Let's put it this way. If I were to test 30 with your code, it would hit the first case statement and ask if it's evenly divisible by 3. It is, so it will print out "Fizz". But it should be printing out "FizzBuzz" as 30 is both evenly divisible by 5 and 3. Try rearranging things a bit and see if you can get it to work.
Hope this helps!

Anthony Boutinov
13,844 PointsIf you put line breaks, your code reads as follows:
for n in 1...100 {
switch n {
case 1:
n%3 == 0 && n%5 == 0
print("FizzBuzz")
case 2:
n%3 == 0
print("Fizz")
case 3:
n%5 == 0
print("Buzz")
default:
print("n")
}
}
Now it's obvious what is wrong here.

Alexander Estefanous
Courses Plus Student 3,579 Pointsnot very elaborative, considering I am a beginner and all...

Anthony Boutinov
13,844 Pointsswitch n {
case /*your case here*/:
// code for that case
// and so on
}
As you can see, your cases are as follows: n == 1, n == 2, n == 3, and default case.

Anthony Boutinov
13,844 PointsAnd what should be happening is this:
case n%3 == 0 && n%5 == 0:
print("FizzBuzz")
case n%3 == 0:
print("Fizz")
case n%5 == 0:
print("Buzz")
default:
print("\(n)")
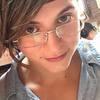
Leslie Chicoine
3,655 PointsThat last answer is almost correct... But... consider that a switch is testing for a case. The above answer shows cases that resolve to true or false (because we're using "&&" and "=="). So there's still something wrong with the way it's written... as it's currently testing for the value of "n", because "n" is written after "switch". Is that helpful, without giving it away?
Rock on!
Alexander Estefanous
Courses Plus Student 3,579 PointsAlexander Estefanous
Courses Plus Student 3,579 PointsThank you so much for your reply!
i see where i went wrong in that regard, but is there an issue the syntax?
even when rearranged, it pulls up the same result :(
for n in 1...100 { switch n { case 1: n%3 == 0 && n%5 == 0; print("FizzBuzz"); case 2: n%3 == 0; print("Fizz"); case 3: n%5 == 0; print("Buzz"); default: print("n") } }
sorry for the trouble!
Alex
Jennifer Nordell
Treehouse TeacherJennifer Nordell
Treehouse TeacherAlexander Estefanous is this for a challenge and if so could you link it? Because I'm fairly sure that challenge is going to require you to rework this as a set of if/else statements and
return
the value instead of print it. Check the instructions again (if this is a challenge) and see if it says anything about print being changed to return.