Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial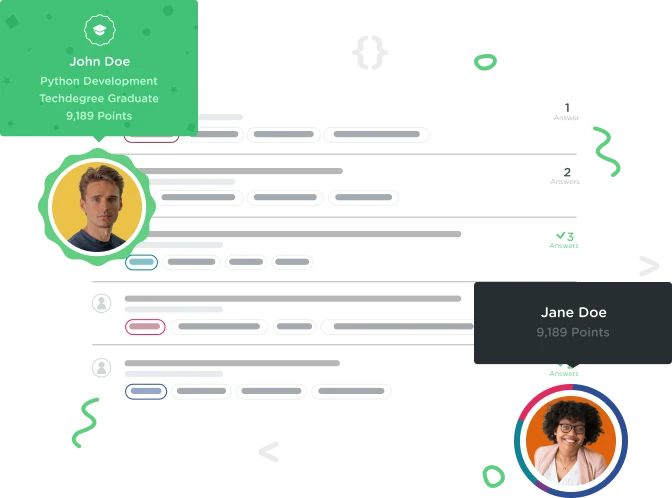

Mayank Sharma
3,025 PointsSyntax error
I was not able to use System.out.println("..........."); statement for "catch"? Why is it so? Can anybody help?
2 Answers

Derek Markman
16,291 PointsI see your syntax problem on your println statement. The reason the syntax is incorrect is because of the %s String modifier, you can only use that inside of a printf statement. To print out your message from the getMessage method on a println statement you use + like I did in the example above.
try {
dispenser.load(400);
System.out.println("This will never happen");
} catch(IllegalArgumentException iae) {
System.out.println("Whoa there!!");
System.out.println("The error was: %s\n", iae.getMessage()); //%s wont work on a println statement.
//Also, you can still use \n on both a println and printf statement.
//instead you need to do:
System.out.println("The error was: " + iae.getMessage());
//if you wanted to use a printf statement:
System.out.printf("The error was: %s", iae.getMessage());
}

Derek Markman
16,291 PointsCould you please post your code so I can get a better understanding of your problem. Also, if you mean "catch" as in a try-catch block you most certainly can use a println statement like so:
public class TryCatchBlocks {
public static void main(String[] args) {
try {
methodThatThrowsAnException();
} catch (Exception e) {
System.out.println("an exception occurred"); //this is redundant if you're using the 'getMessage' method. Unless you want to print out an additional String.
System.out.println("Yikes: " + e.getMessage()); //this is a much better way of printing a statement inside of a try-catch block.
}
}
public static void methodThatThrowsAnException()throws Exception {
throw new Exception("AN EXCEPTION OCCURRED");
}
}
This will output:
an exception occurred
Yikes: AN EXCEPTION OCCURRED

Mayank Sharma
3,025 PointsThis is the code: public class Example {
public static void main(String[] args) {
// Your amazing code goes here...
System.out.println("We are making a new Pez Dispenser.");
PezDispenser dispenser = new PezDispenser("Donatello");
System.out.printf("The dispenser character is %s\n",
dispenser.getCharacterName());
if (dispenser.isEmpty()){
System.out.println("The dispenser is empty.");
}
System.out.println("Loading....");
dispenser.load();
if (!dispenser.isEmpty()){
System.out.println("The dispenser is not empty");
}
while(dispenser.dispense()){
System.out.println("Chomp!");
} if (dispenser.isEmpty()){ System.out.println("Ate all the PEZ!"); } dispenser.load(4); dispenser.load(3); while(dispenser.dispense()){ System.out.println("Chomp!"); } try{ dispenser.load(400); System.out.println("This will never happen"); } catch(IllegalArgumentException iae){ System.out.println("Whoa there!!"); System.out.println("The error was: %s\n", iae.getMessage()); }
}
}
Mayank Sharma
3,025 PointsMayank Sharma
3,025 PointsThanks.
Derek Markman
16,291 PointsDerek Markman
16,291 PointsNo problem, let me know if you have any other questions.