Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial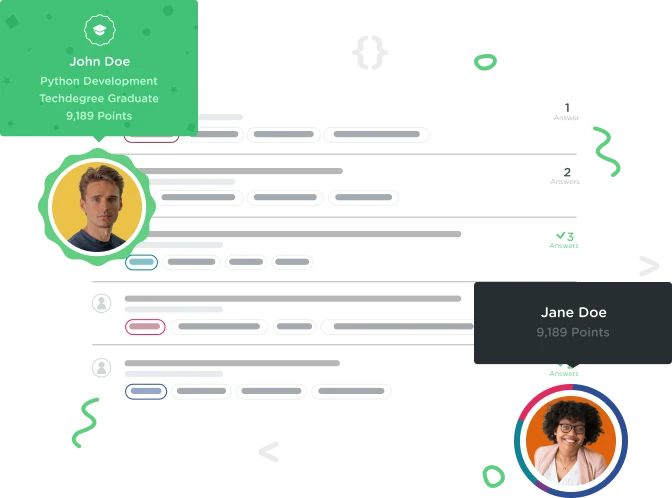
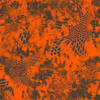
Jake Wright
Courses Plus Student 991 PointsSyntax Error
line 72 syntax error?
if guess in secret_word:
import os
import random
import sys
# make a list of words
words = [
'apple',
'banana',
'orange',
'coconut',
'strawberry',
'lime',
'grapefruit',
'lemon',
'kumquat',
'blueberry',
'melon',
]
def clear():
if os.name =='int':
os.system('cls')
else:
os.system('clear')
def draw(bad_guesses, good_guesses, secret_word):
clear()
print('strikes: {}/7'.format(len(bad_guesses)))
print('')
for letter in bad_guesses:
print(letter, end=' ')
print('\n\n')
for letter in secret_word:
if letter in good_guesses:
print(letter, end='')
else:
print ('_', end='')
print('')
def get_guess(bad_guesses, good_guesses):
while True:
guess = input("guess a letter: ").lower()
if len(guess) != 1:
print("you can only guess a single letter:!")
continue
elif guess in bad_guesses or guess in good_guesses:
print("you've already guess that letter!")
continue
elif not guess.isalpha():
print("you can only guess letters!")
continue
else:
return guess
def play(done):
clear()
secret_word = random.choice(words)
bad_guesses = []
good_guesses = []
while True:
draw(bad_guesses, good_guesses, secret_word)
guess = get_guess(bad_guess(bad_guesses, good_guesses)
if guess in secret_word:
good_guesses.append(guess)
found = True
for letter in secret_word:
if letter not in good_guesses:
found = False
if found:
print("You win!")
print("The secret word was {}".format(secret_word))
else:
bad_guesses.append(guess)
if len(bad_guesses) == 7:
draw(bad_guesses, good_guesses, secret_word))
print("You lost!")
print("the secret word was{}".format(secret_word))
done = True
if done:
play_again = input("Play again? Y/n").lower()
if play_again !+ 'n':
return play(done=False)
else:
sys.exit()
print('Welcome to letter Guess!')
done = False
while True:
clear()
welcome()
play(done)
2 Answers

Nick B
964 PointsThe other answer is incorrect in the context of this script.
guess = get_guess(bad_guess(bad_guesses, good_guesses)
There is no function called bad_guess
, so that line should look like this:
guess = get_guess(bad_guesses, good_guesses)
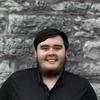
Michael Hulet
47,913 PointsYou have a syntax error, but it's not on line 72. It's actually in the statement before. You simply forgot a closing paren to end the function call above the if
. You currently have this:
get_guess(bad_guess(bad_guesses, good_guesses)
but it should be this:
get_guess(bad_guess(bad_guesses, good_guesses)) #Notice the 2nd closing paren to correspond to the first opening one
The reason Python said it's on line 72 is because Python can only tell you about the place it first detected a syntax error. Sometimes it can detect one right where there's an error, but it's not always like that. In this case, it didn't see a 2nd closing paren before the if
statement started, and so it new at that point that there must've been a syntax error sometime before. Decoding things like that is more of a form of art than a science, and you'll get a lot better at it as you write more code
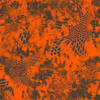
Jake Wright
Courses Plus Student 991 PointsThank you so much Micheal, that really helps.