Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial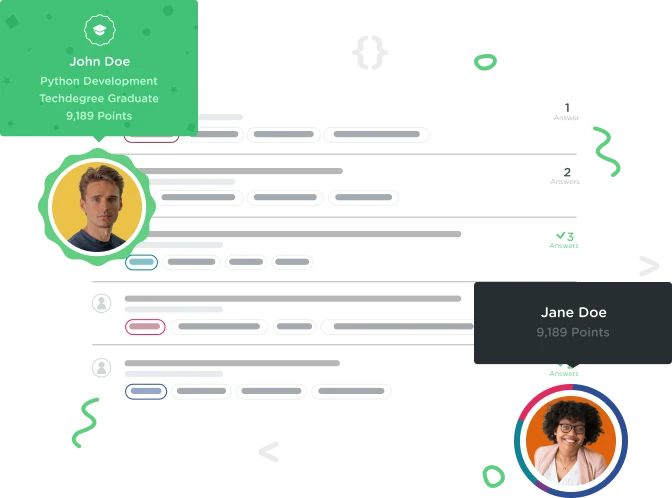
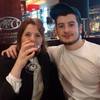
Oliver Sewell
16,425 PointsSyntax error in JSON
Hi i'm following along in my text editor and when i run node it says i have a syntax error, unexpected token
SyntaxError: Unexpected token { in JSON at position 54358
at Object.parse (native)
at IncomingMessage.response.on (/Users/oliver/Desktop/node_practice/app.js:24:24)
at emitNone (events.js:91:20)
at IncomingMessage.emit (events.js:185:7)
at endReadableNT (_stream_readable.js:974:12)
at _combinedTickCallback (internal/process/next_tick.js:80:11)
at process._tickCallback (internal/process/next_tick.js:104:9)
// Problem: We need a simple way to look at a user's badge count and JavaScript points
// Solution: Use Node.js to connect to Treehouse's API to get profile information to print out
const https = require('https');
let username = 'chalkers';
//Function to print message to console
function printMessage(username, badgeCount, points) {
const message = `${username} has ${badgeCount} total badges and ${points} points in Javascript`;
console.log(message);
}
let body = "";
function getProfile(username) {
//Connect to the API Url (https://teamtreehouse.com/username.json)
const request = https.get(`https://teamtreehouse.com/${username}.json`, (response) => {
console.log(response.statusCode);
//Read the data
response.on('data', (dataChunk) => {
body += dataChunk.toString()
});
//Parse the data
response.on('end', () => {
const profile = JSON.parse(body);
console.log(profile);
console.log(typeof profile);
//print the data
printMessage(username,profile.badges.length,profile.points.JavaScript);
});
})
}
getProfile('chalkers');
getProfile('alenaholligan');
1 Answer

Joe Beltramo
Courses Plus Student 22,191 PointsYou are attempting to parse a JavaScript object/string concatenation with the JSON parser. This is happening because your body
variable is not scoped in the function.
// Problem: We need a simple way to look at a user's badge count and JavaScript points
// Solution: Use Node.js to connect to Treehouse's API to get profile information to print out
const https = require('https');
let username = 'chalkers';
//Function to print message to console
function printMessage(username, badgeCount, points) {
const message = `${username} has ${badgeCount} total badges and ${points} points in Javascript`;
console.log(message);
}
let body = ""; // Move this line into the function below
function getProfile(username) {
// Move the `body` line to here <------------
//Connect to the API Url (https://teamtreehouse.com/username.json)
const request = https.get(`https://teamtreehouse.com/${username}.json`, (response) => {
console.log(response.statusCode);
//Read the data
response.on('data', (dataChunk) => {
body += dataChunk.toString()
});
//Parse the data
response.on('end', () => {
const profile = JSON.parse(body);
console.log(profile);
console.log(typeof profile);
//print the data
printMessage(username,profile.badges.length,profile.points.JavaScript);
});
})
}
getProfile('chalkers');
getProfile('alenaholligan');
So since the body is outside of the scope of the function, the first time you call the function, there is no issue. But the second time, body has already been turned into an object that has then been appended with strings. When the JSON parser tries to evaluate the body string, it doesn't know what it is looking at. It sees something like this:
[object Object]{"key": "value"} // Cannot interpret [object Object]