Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial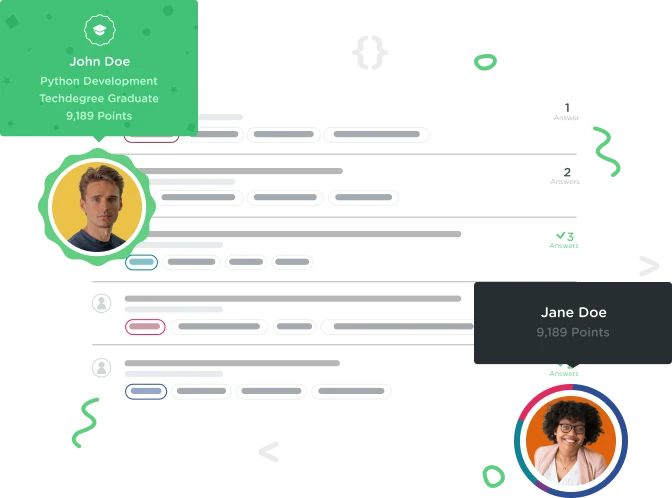
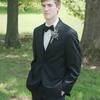
Jamison Imhoff
12,460 PointsSyntax Error Issue with Javascript
I am trying to look at some console logs, but when I do the ctrl+shift+j shortcut on chrome to open the javascript console, it says "SyntaxError: Unexpected end of input".
The weird thing is IT IS ON A COMMENT ON THE 1ST LINE. I do not get how the //comment is being seen as a syntax error, and i cannot look at my console logs because the console stops at the first error.
help... please...
Code is unfinished, but here it is so far:
//Problem: User interaction doesn't provide desired results
//Solution: Add interactivity so the user can manage daily tasks
var taskInput = document.getElementById("new-task"); //new-task
var addButton = document.getElementsByTagName("button")[0]; //first button
var incompleteTasksHolder = document.getElementById("incomplete-tasks"); //incomplete-tasks
var completedTasksHolder = document.getElementById("completed-tasks"); //completed-tasks
//Add a new task
var addTask = function() {
console.log("Add task...");
//When the button is pressed
//Create a new list item with the text from #new-task:
//input checkbox
//label
//input (text)
//button.edit
//button.delete
//Each elements, needs modified and appended
}
//Edit an existing task
var editTask = function() {
console.log("Edit task...");
//When the Edit button is pressed
//if the class of the parent is .editMode
//switch from .editMode
//label text become the input's value
//else
//switch to .editMode
//input value becomes the label's text
//Toggle .editMode
}
//Delete an existing task
var deleteTask = function() {
console.log("Delete task...");
//When the Delete button is pressed
//Remove the parent list item from the ul
}
//Mark a task as complete
var taskCompleted = function() {
console.log("Task complete...");
//When the Checkbox is checked
//Append the task list item to the #completed-tasks
}
//Mark a task as incomplete
var taskIncomplete = function() {
console.log("Task incomplete...");
//When the checkbox is unchecked
//Append the task list item to the #incompleted-tasks
}
var bindTaskEvents = function(taskListItem, checkBoxEventHandler) {
console.log("Bind list item events");
//select it's children
var checkBox = taskListItem.querySelector("input[type=checkbox]");
var editButton = taskListItem.querySelector("button.edit");
var deleteButton = taskListItem.querySelector("button.delete");
//bind editTask to edit button
editButton.onclick = editTask;
//bind deleteTask to delete button
deleteButton.onclick = deleteTask;
//bind taskCompleted to checkbox
checkBox.onchange = checkBoxEventHandler;
}
//Set the click handler to the addTask function
addButton.onclick = addTask;
//cycle over incompleteTasksHolder ul list items
for(var i = 0; i < incompleteTasksHolder.children.length; i++) {
//bind events to list item's children (taskCompleted)
bindTaskEvents(incompleteTasksHolder.children[i], taskCompleted);
}
//cycle over completedTasksHolder ul list items
for(var i = 0; i < incompleteTasksHolder.children.length; i++) {
//bind events to list item's children (taskCompleted)
bindTaskEvents(completedTasksHolder.children[i], taskIncomplete);
1 Answer
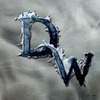
Hugo Paz
15,622 PointsHi Jamison,
You forgot to close the last for loop. Just add the closing curly braces and you should get rid of that error.
Jamison Imhoff
12,460 PointsJamison Imhoff
12,460 PointsOk thanks. That helped, but now line 60 gets "Cannot read property 'querySelector' of undefined" >_>
Hugo Paz
15,622 PointsHugo Paz
15,622 PointsCan you post the HTML as well?
Jamison Imhoff
12,460 PointsJamison Imhoff
12,460 PointsI can post it, but it was not altered in the video lesson:
Hugo Paz
15,622 PointsHugo Paz
15,622 PointsIt seems the issue is in the last for loop,
You have:
but you are supposed to cycle through the completed tasks holder
Jamison Imhoff
12,460 PointsJamison Imhoff
12,460 Pointsfixed the last loop, but line 60 still error
var checkBox = taskListItem.querySelector("input[type=checkbox]");
Hugo Paz
15,622 PointsHugo Paz
15,622 PointsWith all the fixes i have no issues,
For testing purposes i kept all code in the same file
Can you please test this?