Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial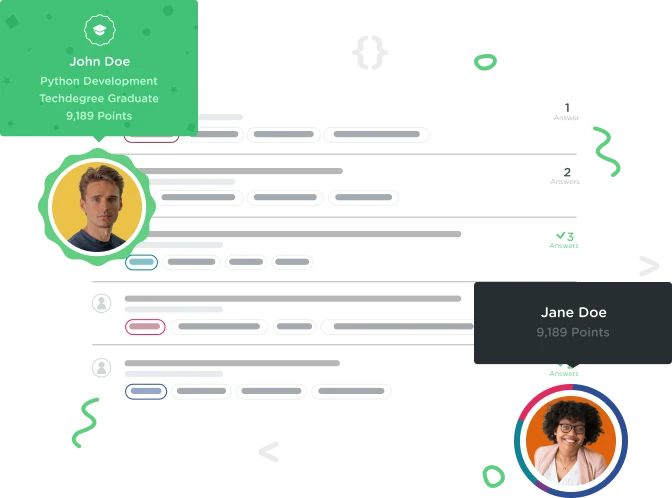
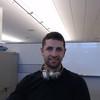
Joel Sprunger
5,448 PointsSyntax question for boolean decisions
I am looking at the Reduce functional programming and Kenneth makes a recursive function to demonstrate reduce().
His code looks something like this
def long_total(a=None, b=None, books=None):
if a is None and b is None and books is None:
print('0')
return None
if a is None and b is None and books is not None:
a = books.pop(0)
b = books.pop(0)
print('1')
return long_total(a, b, books)
if a is not None and b is None and books:
print('2')
b = books.pop(0)
return long_total(a, b, books)
if a is not None and b is not None and books is not None:
print('3')
return long_total(a+b, None, books)
if a is not None and b is not None and not books:
print('4')
return long_total(a+b, None, None)
if a is not None and b is None and not books or books is None:
print('5')
return a
print(long_total(books=[b.price for b in BOOKS]))
I "reduced" the amount of words in the boolean expressions and seem to be getting the same result with the following.
def long_total(a=None, b=None, books=None):
if not a and not b and not books:
print('0')
return None
if not a and not b and books:
a = books.pop(0)
b = books.pop(0)
print('1')
return long_total(a, b, books)
if a and not b and books:
print('2')
b = books.pop(0)
return long_total(a, b, books)
if a and b and books:
print('3')
return long_total(a+b, None, books)
if a and b and not books:
print('4')
return long_total(a+b, None, None)
if (a and not b and not books) or books is None:
print('5')
return a
print(long_total(books=[b.price for b in BOOKS]))
Is this just a matter of taste? Or have I changed how the code operates?
1 Answer
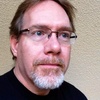
Chris Freeman
Treehouse Moderator 68,460 PointsIt’s the boundary condition that needs to be considered. If zero is a valid value for a variable then
# this
if not a:
# is not equivalent to
if a is None:
The first is checking its “truthiness”, the second checks if the object is the None object. Consider the following comparisons
def t_f_none(obj):
if obj is True:
print('{} is True'.format(obj))
else:
print('{} is not True'.format(obj))
if obj is False:
print('{} is False'.format(obj))
else:
print('{} is not False'.format(obj))
if obj:
print('{} seems truthy'.format(obj))
else:
print('{} is not truthy'.format(obj))
if obj is None:
print('{} is None'.format(obj))
else:
print('{} is not None'.format(obj))
t_f_none(True)
t_f_none(False)
t_f_none(None)
t_f_none(0)
t_f_none(1)
t_f_none(object())
t_f_none('')
t_f_none('a')
Output
True is True
True is not False
True seems truthy
True is not None
False is not True
False is False
False is not truthy
False is not None
None is not True
None is not False
None is not truthy
None is None
0 is not True
0 is not False
0 is not truthy
0 is not None
1 is not True
1 is not False
1 seems truthy
1 is not None
<object object at 0x12d7960a0> is not True
<object object at 0x12d7960a0> is not False
<object object at 0x12d7960a0> seems truthy
<object object at 0x12d7960a0> is not None
is not True
is not False
is not truthy
is not None
a is not True
a is not False
a seems truthy
a is not None
Post back if you need more help. Good luck!!!
Joel Sprunger
5,448 PointsJoel Sprunger
5,448 PointsAwesome. Very thorough.