Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial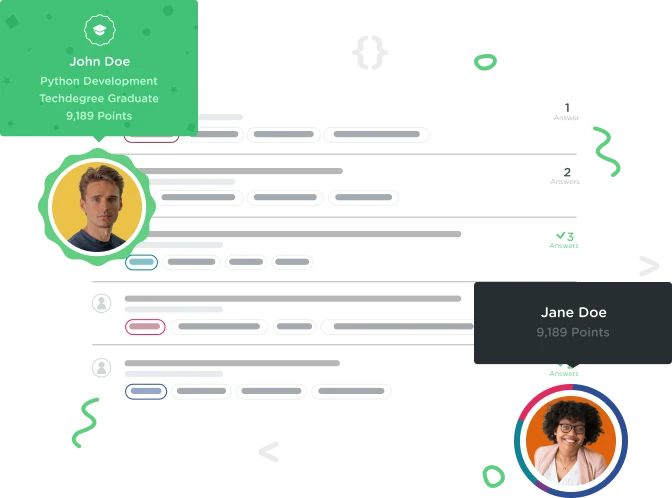

Daven Hietala
8,040 PointsSyntaxError: Unexpected end of input
I have rewatched this video several times and retyped everything just as it says to in the video..
I do not understand what I am doing wrong.
Here is my code..
// Problem: We need a simple way to look at a user's badge count and JavaScript points
// Solution: Use Node.js to connect to Treehouse's API to get profile information to print out
// Require https module
const https = require('https');
const username = "chalkers";
// Function to print message to console
function printMessage(username, badgeCount, points) {
const message = `${username} has ${badgeCount} total badge(s) and ${points} points in JavaScript`;
console.log(message);
}
// Connect to the API URL (https://teamtreehouse.com/username.json)
const request = https.get(`https://teamtreehouse.com/${username}.json`, response => {
let body = "";
// Read the data
response.on('data', data => {
body += data.toString();
});
response.on('end', () => {
// Parse the data
console.log(body);
console.log(typeof body);
// Print the data
});
After running "node app.js" in the console I receive an error that reads as follows..
treehouse:~/workspace$ node app.js
/home/treehouse/workspace/app.js:28
});
^
SyntaxError: Unexpected end of input
at createScript (vm.js:80:10)
at Object.runInThisContext (vm.js:139:10)
at Module._compile (module.js:617:28)
at Object.Module._extensions..js (module.js:664:10)
at Module.load (module.js:566:32)
at tryModuleLoad (module.js:506:12)
at Function.Module._load (module.js:498:3)
at Function.Module.runMain (module.js:694:10)
at startup (bootstrap_node.js:204:16)
at bootstrap_node.js:625:3
I do not know where that semicolon is. Again I have typed everything exactly as instructed as far as I can see.
Can anyone see what I am doing wrong? Thank you in advance!
Daven
2 Answers
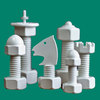
Steven Parker
231,268 PointsCongratulations on resolving your issue. For future reference, the most common cause of "Unexpected end of input" is unbalanced parentheses or braces (or both, as in this case).
If you follow "best practice" intenting, issues like this will be easier to spot since the matching end of a section will be indented the same as the opening.
Giving the function above that indenting style would make it look like this:
// Connect to the API URL (https://teamtreehouse.com/username.json)
const request = https.get(
`https://teamtreehouse.com/${username}.json`,
response => {
let body = "";
// Read the data
response.on("data", data => {
body += data.toString();
});
response.on("end", () => {
// Parse the data
console.log(body);
console.log(typeof body);
// Print the data
});
}
);
Once you get used to this style, the fact that the last line was indented would be a sure sign that the function was uncomplete.

Daven Hietala
8,040 PointsThank you Steven, I will keep that in mind.
By the way, how do you make your code retain the colored text? I am using three back ticks before and after my code "```", yet my code is black and white.
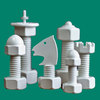
Steven Parker
231,268 PointsFor syntax coloring, put the language name or abbreviation on the line with the first three accents.
Happy coding!
Daven Hietala
8,040 PointsDaven Hietala
8,040 PointsFor anyone else that had this issue, I figured out what I did wrong.
I deleted the final closing curly bracket, parenthesis and semicolon.
When I added the final curly bracket, parenthesis and semicolon, "});", the code executed without any issues.
Programmatically speaking..
When I should have added an additional closing curly bracket, parenthesis and semicolon like this...
Hope this helps someone down the road!