Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial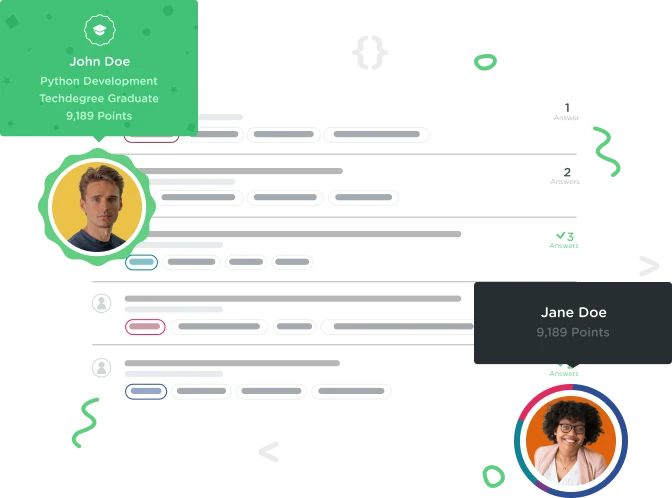
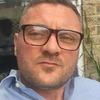
Richard Brown
6,950 PointsSyntaxError: Unexpected token N in JSON at position 0 - Please help
My code:
// Problem: We need a simple way to look at a user's badge count and JavaScript points
// Solution: Use Node.js to connect to Treehouse's API to get profile information to print out
const https = require('https');
function printMessage(username, badgeCount, point){
const message = `${username} has ${badgeCount} total badge(s) and ${point} points in javascript`;
console.log(message);
};
function getProfile(username){
// Connect to the API URL (https://teamtreehouse.com/username.json)
const request = https.get('https://teamtreehouse.com/${username}.json', response => {
let body = "";
// Read the data
response.on('data', data => {
body += data.toString();
});
response.on('end', () =>{
// Parse the data
const profile = JSON.parse(body);
// Print the data
printMessage(username, profile.badges.length, profile.points.JavaScript);
});
});
}
getProfile('chalkers');
what have i done wrong here? Thanks in advance :)
3 Answers
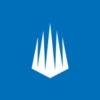
Osama Awan
Courses Plus Student 8,676 PointsInside your getProfile function, you are using Template String but with single quotes 'https://teamtreehouse.com/${username}.json'
in order to make the Template Strings work replace the single quotes with backtick, https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Template_literals

Daniel Paez
Courses Plus Student 4,213 PointsAlso, if anyone else is still running into this issue after evaluating the code it could be the username you are passing into the function. A non-existent one will give you the same error.
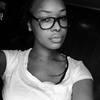
Jasmine Martin
14,307 Pointsoh wow. That was golden thanks! I was using chalker instead of chalkers :(
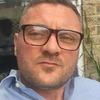
Richard Brown
6,950 PointsMany thanks for your time and input :)