Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial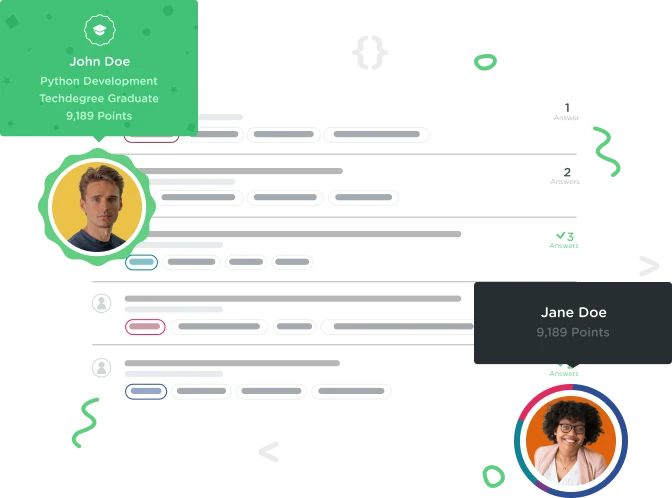
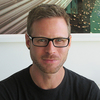
Erik Ralston
1,510 PointsSystem.ArgumentNullException: Value cannot be null. Parameter name: String in C# Basics Final Code Challenge Task 2
Hello, in this step of the code challenge for C# Basics, I keep getting the above error "System.ArgumentNullException" when I submit my code, but the same code runs without error in Workspaces and I can't seem to figure out what value is being set to null and causing the exception to be thrown. The error detail is:
System.ArgumentNullException: Value cannot be null. Parameter name: String at System.Number.StringToNumber (System.String str, NumberStyles options, System.NumberBuffer& number, System.Globalization.NumberFormatInfo info, Boolean parseDecimal) [0x00054] in /builddir/build/BUILD/mono-4.2.1/external/referencesource/mscorlib/system/number.cs:1080 at System.Number.ParseInt32 (System.String s, NumberStyles style, System.Globalization.NumberFormatInfo info) [0x00014] in /builddir/build/BUILD/mono-4.2.1/external/referencesource/mscorlib/system/number.cs:751 at System.Int32.Parse (System.String s) [0x00000] in /builddir/build/BUILD/mono-4.2.1/external/referencesource/mscorlib/system/int32.cs:140 at Treehouse.CodeChallenges.Program.Main () <0x41210830 + 0x0005d> in :0 at MonoTester.Run () [0x00197] in MonoTester.cs:124 at MonoTester.Main (System.String[] args) [0x00013] in MonoTester.cs:27
Thanks for any help
3 Answers
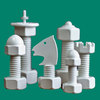
Steven Parker
231,269 PointsI had exactly the same problem, it's because we got a bit over-creative in our solution. The challenge does not require that you re-ask for input if the first one failed the validation. While that produces a better program, it's not good for the challenge. So just eliminate your loop:
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
var count = 0;
Console.Write("Enter the number of times to print \"Yay!\": ");
var entry = Console.ReadLine();
try
{
var numberOfTimes = int.Parse(entry);
while (count < numberOfTimes)
{
Console.WriteLine("Yay!");
count++;
}
}
catch(FormatException)
{
Console.WriteLine("You must enter a whole number.");
}
}
}
}
Now as to why it appears to get a compiler error from a perfectly good piece of code, I have no idea!
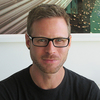
Erik Ralston
1,510 PointsSteven,
Thank you! That was exactly it. It didn't even occur to me that I wouldn't have to ask for new input. But your solution let me get past that step :)
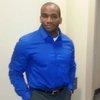
Jonathan Wade Jr
1,051 Pointsi had the same issue found out i put my get set method in wrong place. this is my code.
int counter = 0; int result;
Console.Write("Enter the number of times to print \"Yay!\": ");
try
{
result = Convert.ToInt32(Console.ReadLine());
while (result > counter)
{
Console.WriteLine("Yay!");
counter++;
}
}
catch (FormatException)
{
Console.WriteLine("You must enter a whole number.");
}
Console.ReadLine();
}
}
}

Chloe Viriyapunt
1,300 PointsI had the same issue. Thank you so much Steven Parker.
Erik Ralston
1,510 PointsErik Ralston
1,510 PointsHmm...I thought my code was going to be attached to the post somewhere but I don't see it. Here's what I had in the challenge: