Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial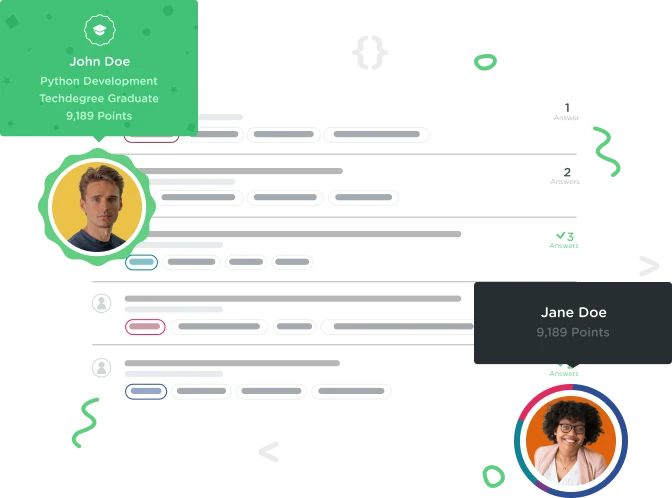
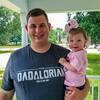
Eric Wilson
9,380 PointsSystem.NullReferenceException when calling GetNewsForPlayer() method.
I have followed the code, but I keep getting a System.NullReferenceException when calling the GetNewsForPlayer() method. I had the method working as expected yesterday, but today it just isn't working. I tried going back to the previous version of my code that was working, and now it's not working. Help!
Here is my code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
using Newtonsoft.Json;
using System.Net;
using System.Net.Http.Headers;
using System.Net.Http;
namespace SoccerStats
{
class Program
{
static void Main(string[] args)
{
string currentDirectory = Directory.GetCurrentDirectory();
DirectoryInfo directory = new DirectoryInfo(currentDirectory);
var fileName = Path.Combine(directory.FullName, "SoccerGameResults.csv");
var fileContents = ReadSoccerResults(fileName);
fileName = Path.Combine(directory.FullName, "players.json");
var players = DeserializePlayers(fileName);
var topTenPlayers = GetTopTenPlayers(players);
foreach (var player in topTenPlayers)
{
var playerName = $"{player.FirstName} {player.SecondName}";
Console.WriteLine(playerName);
List<NewsResult> newsResults = GetNewsForPlayer(string.Format("{0} {1}", player.FirstName, player.SecondName));
foreach(var result in newsResults)
{
Console.WriteLine(string.Format("Date: {0}, Headline: {1}, Summary: {2} \r\n", result.DateLastCrawled, result.Headline, result.Summary));
Console.ReadKey();
}
}
fileName = Path.Combine(directory.FullName, "topten.json");
SerializePlayersToFile(topTenPlayers, fileName);
}
public static string ReadFile(string fileName)
{
using(var reader = new StreamReader(fileName))
{
return reader.ReadToEnd();
}
}
public static List<GameResult> ReadSoccerResults(string fileName)
{
var soccerResults = new List<GameResult>();
using(var reader = new StreamReader(fileName))
{
string line = "";
reader.ReadLine();
while((line = reader.ReadLine()) != null)
{
var gameResult = new GameResult();
string[] values = line.Split(',');
DateTime gameDate;
if (DateTime.TryParse(values[0], out gameDate))
{
gameResult.GameDate = gameDate;
}
gameResult.TeamName = values[1];
HomeOrAway homeOrAway;
if (Enum.TryParse(values[2], out homeOrAway))
{
gameResult.HomeOrAway = homeOrAway;
}
int parseInt;
if (int.TryParse(values[3], out parseInt))
{
gameResult.Goals = parseInt;
}
if (int.TryParse(values[4], out parseInt))
{
gameResult.GoalAttempts = parseInt;
}
if (int.TryParse(values[5], out parseInt))
{
gameResult.ShotsOnGoal = parseInt;
}
if (int.TryParse(values[6], out parseInt))
{
gameResult.ShotsOnGoal = parseInt;
}
double possessionPercent;
if ( double.TryParse(values[7], out possessionPercent))
{
gameResult.PosessionPercent = possessionPercent;
}
soccerResults.Add(gameResult);
}
}
return soccerResults;
}
public static List<Player> DeserializePlayers(string fileName)
{
var players = new List<Player>();
var serializer = new JsonSerializer();
using (var reader = new StreamReader(fileName))
using (var jsonReader = new JsonTextReader(reader))
{
players = serializer.Deserialize<List<Player>>(jsonReader);
}
return players;
}
public static List<Player> GetTopTenPlayers(List<Player> players)
{
var topTenPlayers = new List<Player>();
players.Sort(new PlayerComparer());
int counter = 0;
foreach(var player in players)
{
topTenPlayers.Add(player);
counter++;
if (counter == 10)
break;
}
return topTenPlayers;
}
public static void SerializePlayersToFile(List<Player> players, string fileName)
{
var serializer = new JsonSerializer();
using (var writer = new StreamWriter(fileName))
using (var jsonWriter = new JsonTextWriter(writer))
{
serializer.Serialize(jsonWriter, players);
}
}
public static string GetGoogleHomePage()
{
using (var webClient = new WebClient())
{
byte[] googleHome = webClient.DownloadData("https://www.google.com");
using (var stream = new MemoryStream(googleHome))
using (var reader = new StreamReader(stream))
{
return reader.ReadToEnd();
}
}
}
public static List<NewsResult> GetNewsForPlayer(string playerName)
{
var results = new List<NewsResult>();
using var webClient = new WebClient();
webClient.Headers.Add("Ocp-Apim-Subscription-Key", "7ea759046e5343218afe9da0599ac724");
byte[] searchResults = webClient.DownloadData(string.Format("https://api.bing.microsoft.com/v7.0/news/search?q={0}&mkt-en-us", playerName));
var serializer = new JsonSerializer();
using (var stream = new MemoryStream(searchResults))
using (var reader = new StreamReader(stream))
using (var jsonReader = new JsonTextReader(reader))
{
results = serializer.Deserialize<NewsSearch>(jsonReader).WebPages.NewsResults;
}
return results;
}
}
}
1 Answer
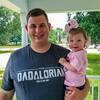
Eric Wilson
9,380 PointsAfter a lot of trial and error, I figured out the issue was with my NewsResults class. I based that class on the sample JSON from the Bing News Results API. I had to back my code up to pull a single JSON search result so I could rebuild my deserialize class.