Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial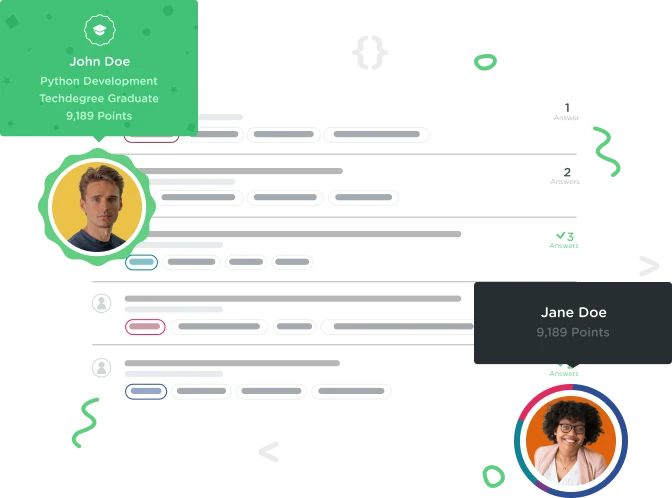
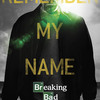
Timothy Johnson
22,480 Pointstable display to another page
I’m building a check in page that connects to a data base and renders the information in a table into an empty div on the page with checkbox embedded into render info. The question is how do i get the checked items once checked and submit button is pressed a popup windows appears with checked items in a table. How would this be done? To pull checked item on one page to the popup page. -Help please
<!--data.php-->
<?php
include 'dbConnect.php';
try {
// prepare query
$query = "select
firstname, lastname
from
users
where
id = ?";
$stmt = $con->prepare( $query );
// this is the first question mark above
$stmt->bindParam(1, $_REQUEST['id']);
// execute our query
$stmt->execute();
// store retrieved row to a variable
$row = $stmt->fetch(PDO::FETCH_ASSOC);
// values to fill up our table
$firstname = $row['firstname'];
$lastname = $row['lastname'];
$id = $row['id'];
//$ = $row[''];
// the table
echo "<table>";
echo "<tr>";
echo "<th>Select: </th>";
echo "<th>Firstname: </th>";
echo "<th>Lastname: </th>";
//echo "<th></th>";
echo "</tr>";
echo "<tr>";
echo "<td><input type='checkbox'
name='checkbox[]' value='<{$id}>'></td>";
echo "<td>{$firstname}</td>";
echo "<td>{$lastname}</td>";
//echo "<td></td>";
echo "</tr>";
echo "</table>";
}catch(PDOException $exception){
// to handle error
echo "Error: " . $exception->getMessage();
}
?>
<!--ck_in.php-->
<html><!--check in page, pulls from data.php-->
<head>
<title></title>
<style>
body{
font-family:arial,sans-serif;
}
select{
margin:0 0 10px 0;
padding:10px;
}
td,th {
background-color:#e8edff;
padding: 10px;
}
</style>
</head>
<body>
<form action= 'post' method = 'selectAll.php'>
<input type = 'submit' value = 'Select All' name = 'selectAll'>
</form>
<?php
// connect to database
include "dbConnect.php";
// retrieve list of users and put it in the select box
$query = "SELECT id, firstname, lastname FROM users";
$stmt = $con->prepare( $query );
$stmt->execute();
//this is how to get number of rows returned
$num = $stmt->rowCount();
// make sure there are records on the database
if($num > 0){
// this will create selec box / dropdown list with user records
echo "<select id='users'>";
// make a default selection
echo "<option value='0'>Select a Student...</option>";
while ($row = $stmt->fetch(PDO::FETCH_ASSOC)){
extract($row);
echo "<option value='{$id}'>{$firstname} {$lastname}</option>";
}
echo "</select>";
}
// if no user records
else{
echo "<div>No records found</div>";
}
// this is where the related info will be loaded
echo "<div id='userInfo'></div>";
?>
<input type = "submit" name = "submit" onclick='window.open("gsu/index.php","","width=500, height=250");'>
<input type = "submit" name = "back" value = "back">
<!--<script src="js/jquery-1.9.1.min.js" ></script>-->
<script>
$(document).ready(function(){
$("#users").change(function(){
// get the selected user's id
var id = $(this).find(":selected").val();
// load it in the userInfo div above
$('#userInfo').load('modules/home/data.php?id=' + id);
});
});
</script>
</body>
</html>