Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial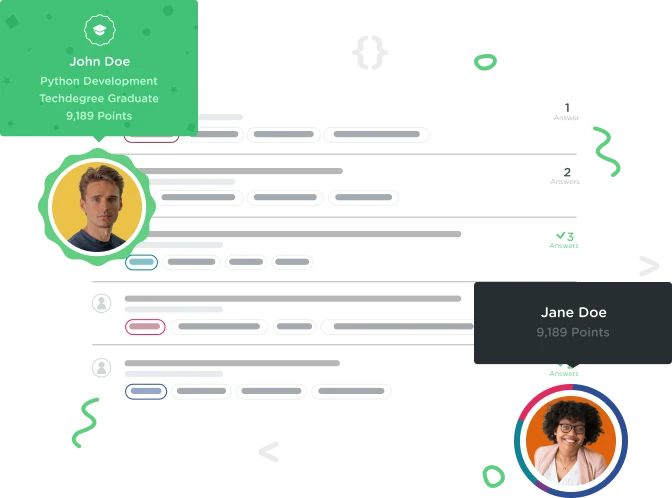

Saif Tase
24,578 PointsTabs not Appearing in Android Studio (Ribbit App)
I've been following along with the Ribbit / Self-Destructing Message app using Android Studio instead of Eclipse/ADT, as shown in the videos.
When I created the main activity of the project, I selected "Tabbed Activity" and chose the swipe-based navigation style. I've been following the videos pretty closely, and made sure to pay attention to the instructions in the notes section, but I can't seem to get tabs to appear in my app at all.
I've tried some of the other solutions suggested here in the forums, but they've only resulted in crashing the app as soon as it starts or loading the app without an action bar (or tabs)..
Could anyone point out what the issue is in my code?
MainActivity.java
package saiftase.com.ribbit;
import android.content.Intent;
import android.os.Bundle;
import android.support.v4.app.Fragment;
import android.support.v4.view.ViewPager;
import android.support.v7.app.ActionBarActivity;
import android.util.Log;
import android.view.LayoutInflater;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.view.ViewGroup;
import com.parse.ParseUser;
public class MainActivity extends ActionBarActivity {
public static final String TAG = MainActivity.class.getSimpleName();
SectionsPagerAdapter mSectionsPagerAdapter;
ViewPager mViewPager;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ParseUser currentUser = ParseUser.getCurrentUser();
if(currentUser == null) {
navigateToLogin();
}else{
Log.i(TAG, currentUser.getUsername());
}
// Create the adapter that will return a fragment for each of the three
// primary sections of the activity.
mSectionsPagerAdapter = new SectionsPagerAdapter(this,
getSupportFragmentManager());
// Set up the ViewPager with the sections adapter.
mViewPager = (ViewPager) findViewById(R.id.pager);
mViewPager.setAdapter(mSectionsPagerAdapter);
}
private void navigateToLogin() {
Intent intent = new Intent(this, LoginActivity.class);
intent.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK);
intent.addFlags(Intent.FLAG_ACTIVITY_CLEAR_TASK);
startActivity(intent);
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.menu_main, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
int itemId = item.getItemId();
if (itemId == R.id.action_logout) {
ParseUser.logOut();
navigateToLogin();
}
return super.onOptionsItemSelected(item);
}
public static class PlaceholderFragment extends Fragment {
private static final String ARG_SECTION_NUMBER = "section_number";
public static PlaceholderFragment newInstance(int sectionNumber) {
PlaceholderFragment fragment = new PlaceholderFragment();
Bundle args = new Bundle();
args.putInt(ARG_SECTION_NUMBER, sectionNumber);
fragment.setArguments(args);
return fragment;
}
public PlaceholderFragment() {
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
View rootView = inflater.inflate(R.layout.fragment_main, container, false);
return rootView;
}
}
}
SectionsPageAdapter.java
package saiftase.com.ribbit;
import android.content.Context;
import android.support.v4.app.Fragment;
import android.support.v4.app.FragmentManager;
import android.support.v4.app.FragmentPagerAdapter;
import java.util.Locale;
/**
* Created by Saif Tase on 1/21/2015.
*/
public class SectionsPagerAdapter extends FragmentPagerAdapter {
protected Context mContext;
public SectionsPagerAdapter(Context context, FragmentManager fm) {
super(fm);
mContext = context;
}
@Override
public Fragment getItem(int position) {
return MainActivity.PlaceholderFragment.newInstance(position + 1);
}
@Override
public int getCount() {
return 2;
}
@Override
public CharSequence getPageTitle(int position) {
Locale l = Locale.getDefault();
switch (position) {
case 0:
return mContext.getString(R.string.title_section1).toUpperCase(l);
case 1:
return mContext.getString(R.string.title_section2).toUpperCase(l);
}
return null;
}
}
2 Answers

Ben Jakuben
Treehouse TeacherThis looks like a frustrating issue with how the project template is set up. When you select "Swipe Views (View Pager)" you need to "Add Tabs to the Action Bar" manually. If you use the 2nd option in the screenshot below, "Action Bar Tabs (with ViewPager)" then this code is generated for you like in our Ribbit project.
Hope this helps!

Graham Gardiner
559 PointsBen Jakuben can you send download links to Eclipse/ADT for Ribbit. It seams like Android Studio is the main option, but I would like to work on what you are using.

Ben Jakuben
Treehouse TeacherI realize this is super late, but in case anyone else wants links to download Eclipse:
- Mac (485 MB)
- Windows 32 bit* (527 MB)
- Windows 64 bit* (526 MB)
Ben Jakuben
Treehouse TeacherBen Jakuben
Treehouse TeacherP.S. I do plan on updating the Ribbit app for Android Studio. I've shared a new public roadmap for Android that you can keep an eye on for details.
Andy Hammond
5,415 PointsAndy Hammond
5,415 PointsIs there anyway to add this mid-project? I started without it, and now its a bit of a mess. may re-do the whole thing :P the review can only help learn though!
Saif Tase
24,578 PointsSaif Tase
24,578 PointsI see - it's kind of confusing because they're all listed as navigation styles in the tabbed activity wizard, and the mechanism for implementing them (Fragments + ViewPager) must be similar, but Swipe Views and the Action Bar Spinner aren't really "tabs" per se.
Here's an example of a Swipe View, from the Android documentation. I've seen it used in the Gmail app and Google Chrome before.
I was previously under the impression it would just be a Tab View bundled with code to handle gestures for swiping between tabs (coming from iOS, where this is not enabled by default in UITabBarControllers).
Here's an example of an Action Bar spinner, also not what we're looking to use here.
Swipe Views
Action Bar - Adding Dropdown Navigation
Hopefully this might help anyone building this project in Android Studio who runs into the same problem.
Georgi Koemdzhiev
12,610 PointsGeorgi Koemdzhiev
12,610 PointsBen, would you consider updating the Ribbit Android App, since I find it really confusing with all of these "deprecated" methods and Activities. Please update the course!