Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial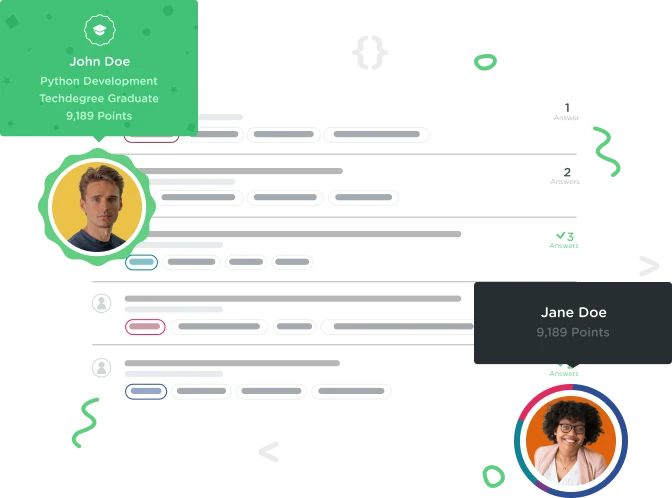

John Weland
42,478 Pointstarget an item in an array returned by a function
Hey guys, I decided to revamp my JavaScript from my previous post found here https://teamtreehouse.com/forum/javascript-utc-time-format where Marcus Parsons and Dave McFarland helped me out.
Previously I have a function googleMapsApi that took in some data did some things and then called another function inside of it to do more work. I wanted to separate them out and make them more flexible, robust and reusable. so I came up with a new function geocoder which is to replace my googleMapsApi function. Geocoder returns an array but now I don't know how to call a specific part of that function for example
the below code is simply pseudo code
geocoder(input).array[0].output;
here is my original go for the googleMapsApi
function googleMapsApi () {
var url = "http://maps.googleapis.com/maps/api/geocode/json?address=" + zipcode + "&sensor=false";
$.ajax(url, {
data : null,
type : "GET",
success : function (response) {
//3.2 Check to see if connection is successful
if (response.status !== "OK") {
//3.2a If connection is unsucessful message
alert("Location Not Found");
} else {
//3.2b If connection is successful, Convert user input to Latitude & Longitude
latitude = response.results[0].geometry.location.lat;
longitude = response.results[0].geometry.location.lng;
var zip = response.results[0].address_components[0].long_name;
var city = response.results[0].address_components[1].long_name;
var state = response.results[0].address_components[2].short_name;
var country = response.results[0].address_components[3].short_name;
loc = city + ", " + state;
}
//console.dir(response);
forecast();
}
});
}
Here is my geocoder
function geocoder (zipcode) {
var url = "http://maps.googleapis.com/maps/api/geocode/json?address=" + zipcode + "&sensor=false";
$.ajax(url, {
data : null,
type : "GET",
success : function (response) {
//3.2 Check to see if connection is successful
if (response.status !== "OK") {
//3.2a If connection is unsucessful message
alert("Location Not Found");
} else {
//3.2b If connection is successful, Convert user input to Latitude & Longitude
var results = [
{
latlng : response.results[0].geometry.location.lat + "," + response.results[0].geometry.location.lng
},
{
"zip" : response.results[0].address_components[0].long_name,
"city" : response.results[0].address_components[1].long_name,
"state" : response.results[0].address_components[2].short_name,
"country" : response.results[0].address_components[3].short_name,
"loc" : "city" + ", " + "state"
}
];
}
console.dir(results[0].latlng);
return results;
}
});
}
1 Answer
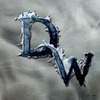
Hugo Paz
15,622 PointsHi John,
I did some changes to your code. Basically i added a callback function which is called when the ajax request receives the response.
This allows you to do something with the data, in this case I just did a console log of the response.
To call ii just go to your console and write geocoder(2000, callback) for example.
function callback(response){
console.log(response);
}
function geocoder (zipcode, callback) {
var url = "http://maps.googleapis.com/maps/api/geocode/json?address=" + zipcode + "&sensor=false";
$.ajax(url, {
data : null,
type : "GET",
success : function (response) {
//3.2 Check to see if connection is successful
if (response.status !== "OK") {
//3.2a If connection is unsucessful message
alert("Location Not Found");
} else {
var city = response.results[0].address_components[1].long_name,
state = response.results[0].address_components[2].short_name,
zip = response.results[0].address_components[0].long_name,
country = response.results[0].address_components[2].long_name;
//3.2b If connection is successful, Convert user input to Latitude & Longitude
var results = {
'latlng' : response.results[0].geometry.location.lat + "," + response.results[0].geometry.location.lng,
'location': {
'zip' : zip,
'city' : city,
'state' : state,
'country' : country,
'loc' : city + ", " + state
}
};
callback(results);
}
}
});
}
John Weland
42,478 PointsJohn Weland
42,478 PointsThanks! I tested it out appending various thins to the response like reponse.latlng and it outputs exactly as I would assume. So The reason I couldn't get this to work is because I needed a callback function which which would take the data out of the call so it could be used else where?
Here is the project in its current state https://github.com/johnweland/weather I am wanting to clean up the code quite a bit and write better more reusable code.
John Weland
42,478 PointsJohn Weland
42,478 PointsI thought I'd be spiffy and re-write my forecast function in the same manner you wrote the geocoder function. It didn't fly. perhaps I need more coffee.
I got a cross-domain issue with this re-write. My original code was.
John Weland
42,478 PointsJohn Weland
42,478 Pointsan update to my current work. Coffee has yielded some results, YAY coffee!
I'd like to reuse the function callback (called geoCB and forecastCB in the above code). I'm not sure if I can create a single callback function that says if the response is coming from function A do this, if its coming from function B do this. then fix up function B enough to call in part of the response of callback (latlng) as of now I have to call forecast with in geoCB to pass that data in to forecast. its a WIP but a lot less code as of now then in the predecessor code.
Hugo Paz
15,622 PointsHugo Paz
15,622 PointsIn the results object you could add an extra property, lets say type: and use its value on the call back function.
something like this:
John Weland
42,478 PointsJohn Weland
42,478 PointsIs that the best practice though? Having a single callback or having a call back defined for each function that requires it? Because now that I've got the data I need to build out my HTML to display it once build for forecast.currently and another block iterated in an each for the forecast.daily
My goal with this rewrite its to make it as modular and as clean as possible.