Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial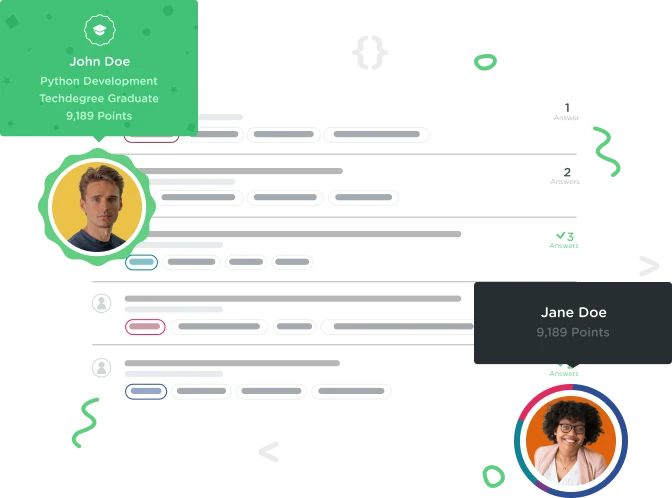

Chris Carr
Front End Web Development Techdegree Student 12,900 Pointstask 1 is no longer passing
What am I doing wrong?
var shanghai = {
population: 14.35e6,
longitude: '31.2000 N',
latitude: '121.5000 E',
country: 'CHN'
};
for (var prop in shanghai) {
console.log (prop);
console.log(shanghai[prop]);
}
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Objects</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
2 Answers
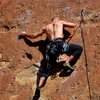
Shawn Flanigan
Courses Plus Student 15,815 PointsHi Chris,
You're almost there, but the challenge asks you to string 2 pieces of information together for each log statement (the name of the property and it's value...separated by a colon). The way you've written your code, you're sending 8 lines to the log, like this:
population
14.35e6
longitude
31.2000 N
latitude
121.5000 E
country
CHN
Instead, you'll want to string your outputs together to send just 4 lines to the log. Remember, to concatenate strings and variables together, you'll use a +. Hope that helps, but let me know if you need a little more clarification on how to get the desired output.

Chris Carr
Front End Web Development Techdegree Student 12,900 PointsThank you that worked. Was not looking at the obvious.
Chris Carr
Front End Web Development Techdegree Student 12,900 PointsChris Carr
Front End Web Development Techdegree Student 12,900 PointsThis passes task one but still does not work.
and this outputs task one no longer passing
Shawn Flanigan
Courses Plus Student 15,815 PointsShawn Flanigan
Courses Plus Student 15,815 PointsChris,
Let's go back to the way you were solving the problem before. Here's the for loop you had originally:
You were close here, you just needed to combine your 2 log statements into one. That would look something like this:
This will give you 4 lines in your log that look something like:
Now, to clean it up and make it readable, they ask us to add a colon and a space in the middle of each log line. So...we'll add a string literal into your console.log command and you end up with something like:
This should give you the desired output.