Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial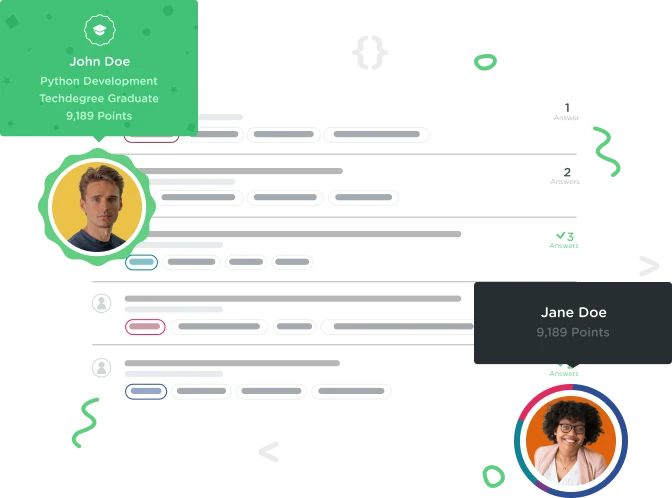

Cesar Manrique
3,708 PointsTask 1 is no longer passing
i dont understand what im doing wrong
public class Order {
private String itemName;
private int priceInCents;
private String discountCode;
public Order(String itemName, int priceInCents) {
this.itemName = itemName;
this.priceInCents = priceInCents;
}
public void applyDiscountCode(String discountCode) {
this.discountCode = normalizeDiscountCode(discountCode);
}
private String normalizeDiscountCode(String discountCode) {
for(int i = 0; i<discountCode.length() ;i++){
char letter = discountCode.charAt(i);
if(!Character.isLetter(letter)){
throw new IllegalArgumentException("Invalid Discount Code");
}else if(discountCode.indexOf("$") == -1) {
throw new IllegalArgumentException("Invalid Discount Code");
}
}
this.discountCode = discountCode.toUpperCase();
return this.discountCode;
}
}
1 Answer
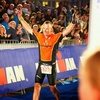
Steve Hunter
57,712 PointsHi Cesar,
You've correctly identified that you want to convert the string to a char array and loop through it. You've gone about that in a longer way than is required, though. Try something like:
for(char letter : discountCode.toCharArray()){
// do stuff
}
Then, you must combine the two conditions else you'll throw an exception when you don't want to. Take your code:
if(!Character.isLetter(letter)){
throw new IllegalArgumentException("Invalid Discount Code");
}
This will throw an exception if the letter
is a '$', which isn't what you want. This test is correct !Character.isLetter(letter)
but this isn't: if(discountCode.indexOf("$") == -1)
. The second test will be true if any character is a '$'. You want to test each letter in turn. So, if your discount code is "1codes$"
and you're testing the first character; it'll fail (correctly) the isLetter
test but the second test will pass as there is a '$' in the string somewhere. Test for the dollar sign at each character, something like letter == '$'
.
You'll need to think about the logic here. You want to throw the exception if any character is not a letter and not a dollar sign. Combine those two together; throw the exception if neither test passes.
Lastly, return
the uppercased code; don't set this.discountCode
- you do that in applyDiscountCode
.
Let me know how you get on.
Steve.