Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial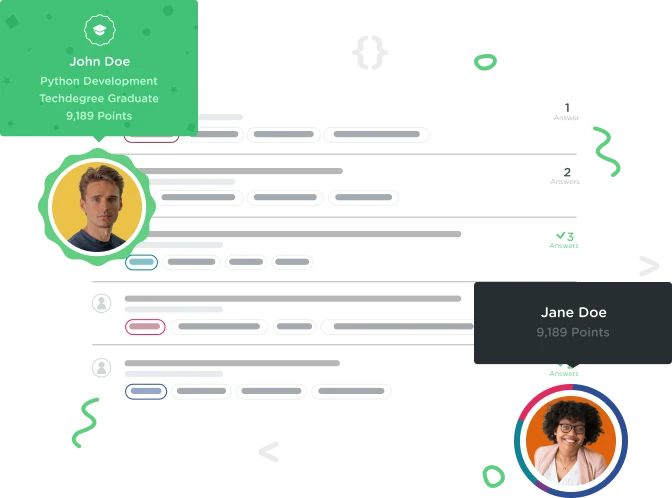

Harrison Cassedy
Java Web Development Techdegree Graduate 12,031 Pointstask 1 of 3. Currently trying to see where I went wrong with my implementation.
In the ContactDao and ContactService interfaces, add a save method method that has a void return type and accepts a Contact object as its only parameter. I added the save method but don't see where I went wrong. It could be an oversight or something very simple. either way please look over it and provide some feedback for this novice coder. Thank you to those that contribute in advance.
package com.teamtreehouse.contactmgr.dao;
import com.teamtreehouse.contactmgr.model.Contact;
import java.util.List;
public interface ContactDao {
List<Contact> findAll();
Contact findById(Long id);
}
package com.teamtreehouse.contactmgr.dao;
import com.teamtreehouse.contactmgr.model.Contact;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Repository;
import java.util.List;
@Repository
public class ContactDaoImpl implements ContactDao {
@Autowired
private SessionFactory sessionFactory;
@Override
public List<Contact> findAll() {
Session session = sessionFactory.openSession();
List<Contact> contacts = session.createCriteria(Contact.class).list();
session.close();
return contacts;
}
@Override
public Contact findById(Long id) {
Session session = sessionFactory.openSession();
Contact c = session.get(Contact.class, id);
session.close();
return c;
}
@Override
public void save(Contact contact) {
contactDAO.save(contact);
}
package com.teamtreehouse.contactmgr.service;
import com.teamtreehouse.contactmgr.model.Contact;
import java.util.List;
public interface ContactService {
List<Contact> findAll();
Contact findById(Long id);
}
package com.teamtreehouse.contactmgr.service;
import com.teamtreehouse.contactmgr.dao.ContactDao;
import com.teamtreehouse.contactmgr.model.Contact;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
@Service
public class ContactServiceImpl implements ContactService {
@Autowired
private ContactDao contactDao;
@Override
public List<Contact> findAll() {
return contactDao.findAll();
}
@Override
public Contact findById(Long id) {
return contactDao.findById(id);
}
@Override
public void save(Contact contact) {
contactDAO.save(contact);
}
1 Answer
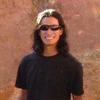
Daniel Vargas
29,184 PointsHi Harrison,
You should just create those methods in the interfaces first (at least in the task one):
public interface ContactService {
List<Contact> findAll();
Contact findById(Long id);
void save(Contact contact);
}
public interface ContactDao {
List<Contact> findAll();
Contact findById(Long id);
void save(Contact contact);
}
In the tasks 2 and 3 they then ask you to implement those methods in the ContactServiceImpl
and ContactDaoImpl
clases
Daniel Vargas
29,184 PointsDaniel Vargas
29,184 PointsAnd in tasks 2 and 3 be careful with this:
contactDao.save(contact)
ContactDaoImpl
you can't call thecontactDao.save(contact)
method, because you are just defined it (it would be an infite loop). You should implement the save method opening a session, saving the contact and the closing the session (there are more steps between opening and closing the session but I don't want to give the answer away).I hope I was clear enough, if you have any trouble let me know.