Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial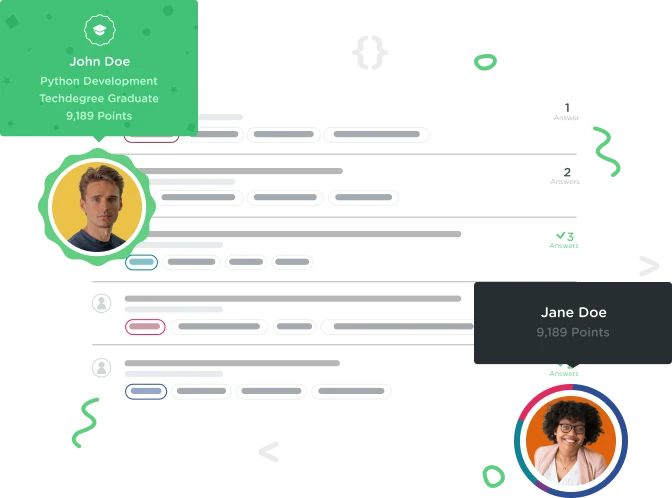
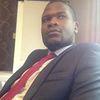
Tapiwanashe Taurayi
15,889 Pointstask 1 of 3 handler
Task 1 of 3We're currently creating a new Thread each time we want to update our Twitter app. Instead, let's now use a Handler. Start by creating a new inner class extending Handler, and move the call to 'twitterClient.update()' into this new Handler's 'handleMessage' method.
public class MainActivity extends Activity {
Button button;
TwitterClient twitterClient = new TwitterClient();
@Override
public void onCreate(Bundle savedInstanceState) {
setContentView(R.layout.activity_main);
final TwitterThread twitterThread = new TwitterThread();
twitterThread.setName("TwitterThread");
twitterThread.start();
button = (Button) findViewById(R.id.update_button);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v){
}
});
}
class TwitterThread extends Thread {
@Override
public void run() {
twitterClient.update();
}
}
}
2 Answers
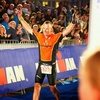
Steve Hunter
57,712 PointsHi there,
There are a few steps to this first task - it isn't a simple one to figure out! Let's break it down into small parts.
The first part of the question is Start by creating a new inner class extending Handler. This will look like the TwitterThread
class that's there already, i.e. it is inside the MainActivity
class. I've called it TwitterHandler
. The skeleton of it will look like:
class TwitterHandler extends Handler{
}
So, we have a class now. The next part of the question is move the call to 'twitterClient.update()' into this new Handler's 'handleMessage' method. So, we need a method called handleMessage
inside this new class. Let's just put an empty method in there and work our way through the errors that throws up:
class TwitterHandler extends Handler{
public handleMessage(){
}
}
That gives an error about the return type. Let's guess that it returns nothing, so add void
to see if that's accepted.
class TwitterHandler extends Handler{
public void handleMessage(){
}
}
That clears the error in Preview but the code challenge says Bummer! Don't forget to override the 'handleMessage(Message msg)' method of you new Handler. Aha! So we're dealing with an overriden method from the extends Handler
and we now it know it takes a parameter too, so let's add those two things - one is the @Override
keyword and the other is the parameter, Message msg
:
class TwitterHandler extends Handler{
@Override
public void handleMessage(Message msg){
}
}
Now we're making some progress! Adding that clears any issues in Preview again, but the next error on checking the code is Bummer! Don't forget to move the 'twitterClient.update()' call out of the Thread and into the Handler. Tat's the last part of the task! So, move the update
code out of TwitterThread
and into this new method.
The final code looks like:
class TwitterHandler extends Handler{
@Override
public void handleMessage(Message msg){
twitterClient.update();
}
}
And that's Task 1 passed!! Good luck!
Steve.

Gift Nyamapfene
5,409 Pointspublic class MainActivity extends Activity { Button button; TwitterClient twitterClient = new TwitterClient();
@Override public void onCreate(Bundle savedInstanceState) { setContentView(R.layout.activity_main);
final TwitterThread twitterThread = new TwitterThread();
twitterThread.setName("TwitterThread");
twitterThread.start();
button = (Button) findViewById(R.id.update_button);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v){
}
});
}
class TwitterThread extends Thread { @Override public void run() {
}
}
class TwitterHandler extends Handler{
@Override
public void handleMessage(Message msg){
twitterClient.update();
}
}
}