Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial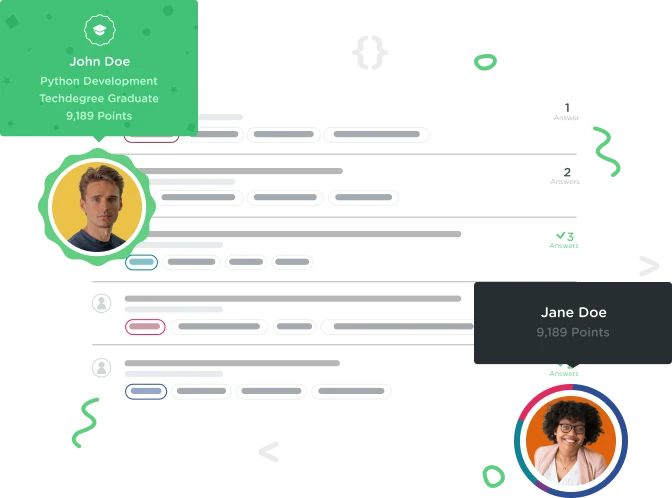
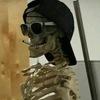
Ian Cole
454 PointsTask 1 suddenly not passing on last step even though Task 1 is literally just importing the random library??????
Exactly what it says on the tin. I get to the last step of this challenge and suddenly Task 1 stops passing even though Task 1 is just importing the random library. I cannot express how frustrated I am in words without breaking community guidelines. Is it something with my code?
import random
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
start = 5
while start not False:
numb = random.randint(1, 99)
if numb % 2 == 0:
print("{} is even".format(numb))
else:
print("{} is odd".format(numb))
start -= 0
3 Answers

Kip Yin
4,847 PointsTask 1 is not passing because you have a SyntaxError
in your while
loop: start not False
. You may either do while start
, which breaks the loop as soon as start == 0
, or do while start != 0
. The former way is preferred in Python.
Also, I noticed that you have start -= 0
at the end. This is not decrementing start
. Typo?
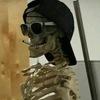
Ian Cole
454 PointsAgh, I had an already existing typo made after I had put in the code for the discussion. Thanks a ton for your help!

Kip Yin
4,847 PointsNo problem! You can click "Best Answer" to mark this question as solved.
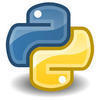
Gerald Wells
12,763 PointsTrue and False are actually have a base type of Int, here is a little trick to exploit that and reduce your overall lines of code.
start = 5
while start > 0:
rand = random.randint(1,99)
print("{} is {}".format(rand, (True,False)[int(even_odd(rand))]))
start -= 1

Kip Yin
4,847 PointsIt's interesting that your answer is even valid since it should be ("even", "odd")
instead of (True, False)
.
However, if (True, False)
is valid, why not just
while start:
rand = random.randint(1, 99)
print("{} is {}".format(rand, even_odd(rand))
start -= 1
Amazingly, this also works:
while start:
print("{0} is {0}".format(random.randint(1, 99)))
start -= 1
And this:
while start:
print("{} is".format(random.randint(1, 99)))
start -= 1
This might be the shortest code possible for this problem.
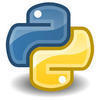
Gerald Wells
12,763 PointsTrue and False base values are 1, 0 respectivley. So by looking for a not true value those values are inversed and being that the predefined values for odd and even now map to the interger values as defined in the function its logical that my solution is valid.
Kip Yin
4,847 PointsKip Yin
4,847 PointsOtherwise, your code is good to go.
Ian Cole
454 PointsIan Cole
454 PointsThanks for pointing that out, knowing me I would have never noticed. Now it's saying that there is too many prints as the error... Go figure, almost done with a course and the only part I have trouble with is the last part
Kip Yin
4,847 PointsKip Yin
4,847 PointsI changed the while-loop condition to
start
and changed the last line tostart -= 1
and nothing else. Passed the challenge without a problem.If you also changed the same things as I did, your code should look exactly like this: