Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial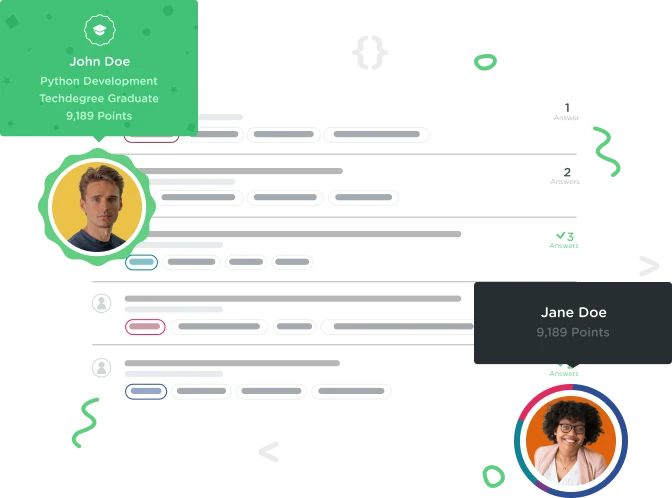
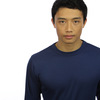
AJ Perez
Courses Plus Student 969 Pointstask #2
can someone help me figure this one out? how do you call the greeting and pass the string "jerry" to it??
4 Answers

Kyle Pontius
6,190 PointsGreat question, Swift is a little different than many here:
If it's not using the method labels: greeting("jerry")
If you program is using method labels: greeting(labelHere: "jerry")
I hope this is helpful. I've included a couple references in case you would like extra clarification: https://developer.apple.com/library/ios/documentation/swift/conceptual/swift_programming_language/Functions.html http://stackoverflow.com/questions/24045890/why-does-a-function-call-require-the-parameter-name-in-swift
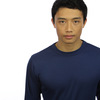
AJ Perez
Courses Plus Student 969 Pointsthank you for your reply! i got this and something is wrong with my second line: func greeting(#person: String) { greeting(person: "Jerry") println("Hello (person)") } i even tried replacing "person" on the second line with "String" and it was still wrong. any ideas?

Kyle Pontius
6,190 PointsIf I'm reading your function correctly it's going something like?
greeting(#person: String) {
greeting(person: "Jerry")
println("Hello (person)")
}
While I don't know exactly what the requirements are for the challenge, I'd recommend changing the following to be syntactically correct:
1 - the 2nd lines that says: greeting(person: "Jerry")
needs to be moved outside the greeting(#person: String)
function. You shouldn't be calling the greeting(#person: String)
function from inside itself. The principle of calling a method/function within itself is called recursion, and I'm sure it's not what you're trying to do.
2 - After you've moved greeting(person: "Jerry")
outside of the greeting(#person: String)
method, check the spelling of "Jerry" to ensure that it matches the requirements. By this I mean the "J" may need to be lowercase.
3 - Also, the last line where it says println("Hello (person)")
, you'll want to change it to println("Hello \(person)")
. The extra slash tells Swift you're inserting a variable into a string, and you want to read out whatever's in the variable. Does that make sense?
Please let me know if this doesn't iron it out for you. These challenges can be a little frustrating sometimes since they're either hit or miss. Good luck!
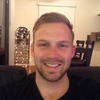
Taylor Smith
iOS Development Techdegree Graduate 14,153 Pointstry this:
func greeting(person: String) -> (String) {
let greeting = "Hello \(person)"
return (greeting)
}
var result = greeting("Jerry")
println(result.0)