Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial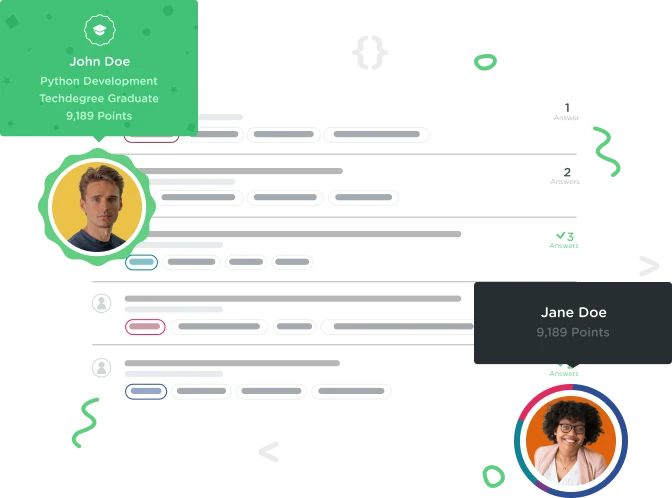

Mike Ulvila
16,268 PointsTask 2 of 3: Implement the multiply method.
Will someone please help me with the code for the multiply method. I keep getting errors and can't figure it out. Thanks

Mike Ulvila
16,268 Pointsthis is one of many i've tried. i know it's not right because it would be passing the same number as the value. var calculator = { sum: 0, add: function(value) { this.sum += value; }, subtract: function(value) { this.sum -= value; }, multiply: function(value) { this.sum = this.add(value) * value; },
2 Answers
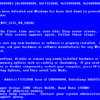
Daniel Powell
23,321 PointsYou appear to be making the task more complicated than it needs to be.
Try to keep it simple:
function(value) {
this.sum *= value
}
You can do the same thing with the '/=' operator as well.
If you want to make it more verbose, you could do:
function(value) {
this.sum = (this.sum * value)
}

Mike Ulvila
16,268 PointsOk, thank you, that works. I guess in my mind I was thinking since the sum = 0, no matter what value you put it it's going to return 0 right???
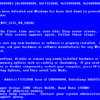
Daniel Powell
23,321 PointsYou can't assume the sum will always be equal to 0. It could very well end up holding a running total that will be updated many times. In that case, your original formula could produce incorrect results.
By keeping the multiply function's code very narrow in functionality you end up programming defensively against possible future errors. And that's always a good thing.
I also edited my answer to include another example if you want to see the non-shorthand code.

Mike Ulvila
16,268 PointsOk, thanks again for your help!
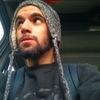
Cosimo Scarpa
14,047 PointsThe result need to be something like this
var calculator = {
sum: 0,
add: function(value) {
this.sum = (this.sum + value)
},
subtract: function(value) {
this.sum = (this.sum - value)
},
multiply: function(value) {
this.sum = (this.sum * value)
},
divide: function(value) {
this.sum = (this.sum / value)
},
clear: function() {
this.sum = 0;
},
equals: function() {
return this.sum;
}
}
Daniel Powell
23,321 PointsDaniel Powell
23,321 PointsPlease post the code you have tried so far.