Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial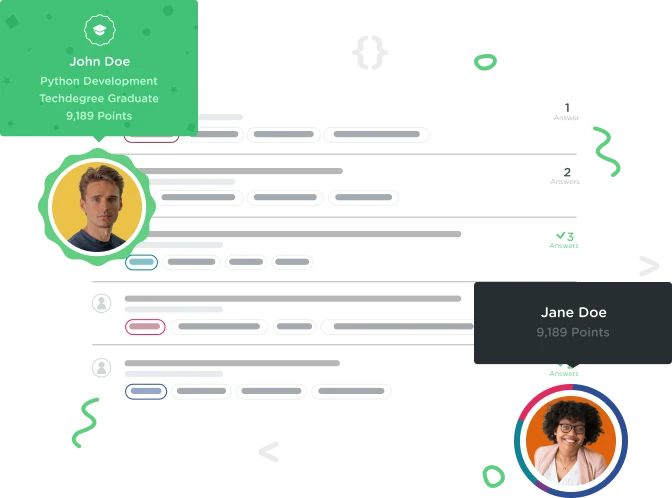
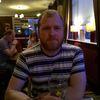
Jamie Wyton
3,011 PointsTask #2 on C# basics....
Can someone spot what ive done wrong here....
using System;
namespace Treehouse.CodeChallenges { class Program { static void Main() { while(true) { Console.Write("Enter the number of times to print \"Yay!\": "); string times = Console.ReadLine(); try { int number = int.Parse(times); } catch(FormatException) { Console.WriteLine(“You must enter a whole number.”); continue; } while(number != 0) { Console.WriteLine("Yay!"); number -= 1; } break; } } } }
6 Answers
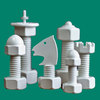
Steven Parker
230,274 PointsIt looks like you have an outer loop that prevents the program from ending.
The challenge expects the program to end after it outputs either they sequence of "Yay!" messages or an error message. But you have an outer loop that prevents it from ending after an error.
In future, please use the instructions for code formatting in the Markdown Cheatsheet pop-up below the "Add an Answer" area. And also provide a link to the course page you are working with.
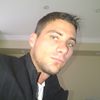
John Lukacs
26,806 PointsWhat is an Outer Loop? The question asks for it doesnent it. How do I stop the thing Im so close but so far
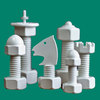
Steven Parker
230,274 PointsThe only loop you need is the one that prints out "Yay!" a number of times. By "outer loop" I mean one that encloses the other loop (and much of the program). In particular you do not want a loop that might cause the program to ask for input more than one time.
If you format your code I could give you specific line number references.
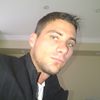
John Lukacs
26,806 Pointsusing System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.Write("Enter the number of times to print \"Yay!\": ");
string numofthing = Console.ReadLine();
try{
int num = int.Parse(numofthing);
for (var i = 0; i<num; i++){
Console.WriteLine("\"Yay!\"");
}
}
catch(FormatException){
Console.WriteLine("You must enter a whole number.");
}
}
}
}
The only thing in the loop is the Console.WriteLine ("\"Yay!\"") this is the error I get Program.cs(16,21): error CS0139: No enclosing loop out of which to break or continue
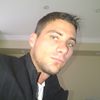
John Lukacs
26,806 PointsOk I looked it up sorry its my second day learning C#
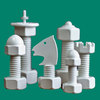
Steven Parker
230,274 PointsYou're zooming along pretty fast then. Is this resolved now?
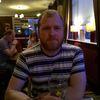
Jamie Wyton
3,011 Pointsusing System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
while(true)
{
Console.Write("Enter the number of times to print \"Yay!\": ");
string notimes = Console.ReadLine();
try
{
int noyays = int.Parse(notimes);
}
catch(FormatException)
{
Console.WriteLine(“You must enter a whole number.”);
Continue;
}
Break;
}
while (noyays != 0)
{
Console.WriteLine("Yay!");
noyays -= 1;
}
}
}
}
Ive given it another shot but....
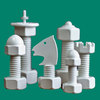
Steven Parker
230,274 PointsYou still have a loop around the part that asks for input.
As I said yesterday, you do not want a loop that might cause the program to ask for input more than one time.
If the input is valid, you get some "Yay!"s. If the input is not valid, you get an error message. Either way, the program should end.
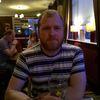
Jamie Wyton
3,011 PointsGot it.