Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial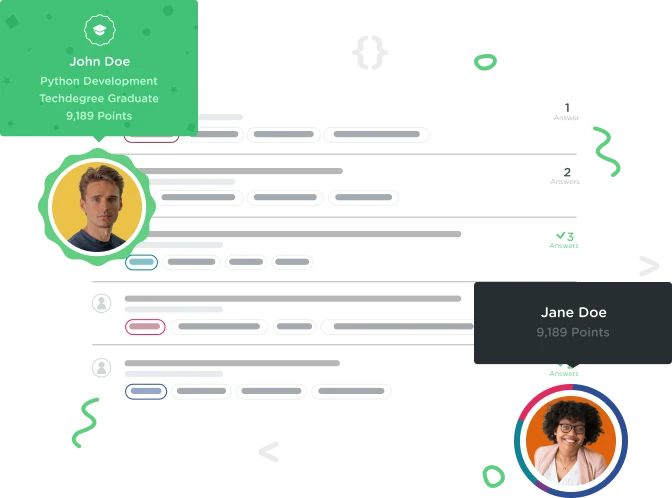

Zachary Martin
3,545 Pointstask 2 why does my code not run?
See below
public class Order {
private String itemName;
private int priceInCents;
private String discountCode;
public Order(String itemName, int priceInCents) {
this.itemName = itemName;
this.priceInCents = priceInCents;
}
public String getItemName() {
return itemName;
}
public int getPriceInCents() {
return priceInCents;
}
public String getDiscountCode() {
return discountCode;
}
private String normalizeDiscountCode (String discountCode) {
for(char discountValid : discountCode.toCharArray){
if(!discountValid == char || $) {
throw new IllegalArgumentException("Invalid discount code");
}
}
return discountCode.toUpperCase();
}
public void applyDiscountCode(String discountCode) {
this.discountCode = normalizeDiscountCode(discountCode);
}
}
1 Answer
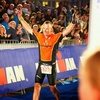
Steve Hunter
57,712 PointsI've added some comments against your code and some ideas below:
private String normalizeDiscountCode (String discountCode) {
for(char discountValid : discountCode.toCharArray){ // good but toCharArray is a method - add () after it
if(!discountValid == char || $) { // you can't compare to a data type and a char should be surrounded by ''
throw new IllegalArgumentException("Invalid discount code");
}
}
return discountCode.toUpperCase(); // correct
}
So, the key issue is your conditional statement.
You have a for
loop that pulls an individual char
one at a time. It is called discountValid
. You want to see if
it is a letter. I Googled java see if a char is a letter
and got this result in the Java docs.
This details the use of a method called isLetter()
. Have a read and try using that.
You have tried to compare to a data type. You can't do that. Also, if you have more than one condition, you must repeat the whole test. You can't say:
if(number > 9 && < 11){
// do something
}
It must be explicit:
if(number > 9 & number < 11){
// do something
}
So, you need to see if
your variable discountValid
is not a letter, see the method above, and is not a $
. Note the language there. You have used ||
. So, if the discountValid
character is a $
, this isn't a letter, so your use of a logical or will give the wrong result. It wants to see if the character isn't a letter and isn't a dollara sign.
Have a crack at that and let me know how you get on.
Steve.