Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial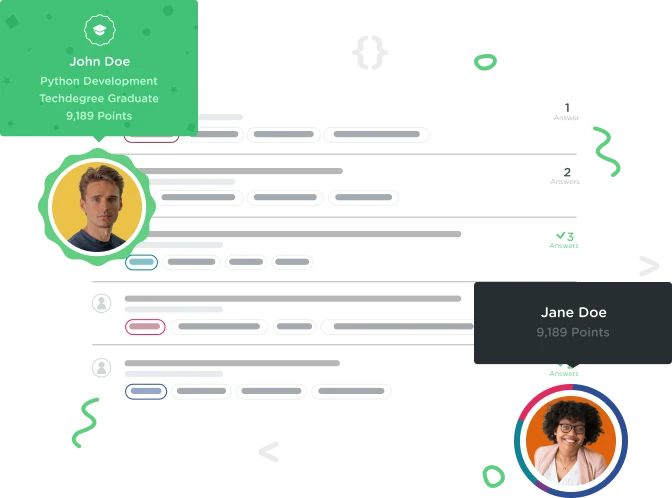
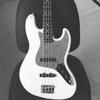
Lee Puckett
12,539 PointsTask 3 of 3 Not sure if I am updating the correct properties.
part 1: Update the Courses navigation collection property to be of type CourseStudent.
So my thought was to change public ICollection<Course> Courses {get; set;} to... public CourseStudent Courses {get; set;}
part 2: Update the default constructor to initialize the Courses property to an instance of List<CourseStudent>
....what is the "default constructor"?
I listened for that key word int the video but never hear it.
using System.Collections.Generic;
namespace Treehouse.CodeChallenges
{
public class Course
{
public Course()
{
Students = new List<CourseStudent>();
}
public int Id { get; set; }
public int TeacherId { get; set; }
public string Title { get; set; }
public string Description { get; set; }
public int Length { get; set; }
public Teacher Teacher { get; set; }
public ICollection<CourseStudent> Students { get; set; }
}
}
namespace Treehouse.CodeChallenges
{
public class CourseStudent
{
public int Id {get; set;}
public int CourseId {get;set;}
public int StudentId {get;set;}
public Course Course {get;set;}
public Student Student {get;set;}
public decimal Grade {get; set;}
}
}
using System.Collections.Generic;
namespace Treehouse.CodeChallenges
{
public class Student
{
public Student()
{
Courses = new List<Course>();
}
public int Id { get; set; }
public string FirstName { get; set; }
public string LastName { get; set; }
public ICollection<Course> Courses { get; set; }
}
}
6 Answers
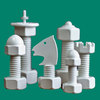
Steven Parker
231,269 PointsYou said, "change public ICollection<Course> Courses {get; set;} to... public CourseStudent Courses {get; set;}"
This is like what you already did to the other class in task 2. It's still a collection, but of a different type:
public ICollection<CourseStudent> Courses { get; set; }
And the "default constructor" is the one which has no parameters. But that's the only constructor either of these classes have, so there's no opportunity for confusion.
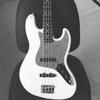
Lee Puckett
12,539 PointsOkay I changed the first part. My confusion was based on:
I thought the type was ICollection<Course> since in every other example, the type precedes the property (e.g. int for ID, string, for FirstName, etc.) therefore I assumed ICollection<Course> was the type. But you are saying in the case of Courses, the type is within the <>? Why is that?
Thanks
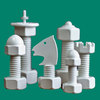
Steven Parker
231,269 PointsBefore the change, you're right that the type of "Courses" is "ICollection<Course>", which is a collection of "Course" objects. The challenge is asking you to change it to a collection of "CourseStudent" objects. It is still a "ICollection", the brackets enclose what type of thing is in the collection.
This is exactly the same change you already made to the other class in task 2.
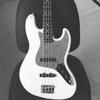
Lee Puckett
12,539 PointsNow if I understand correctly, for the second part of this challenge I need to enter Courses = new List<CoursesStudent>() But where does this go? Does it stand alone or do I paste inside of ()?
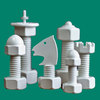
Steven Parker
231,269 PointsYou're not inserting a new line, just changing the List type in the existing constructor code.
Again, you already did exactly the same exact thing to the other class in task 2.
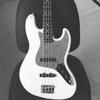
Lee Puckett
12,539 PointsI don't know what the list type is.
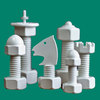
Steven Parker
231,269 PointsThe code is provided initially is:
Courses = new List<Course>();
So the list type is "Course" (inside the <>'s). So you'll be changing that, just like you already did in the other class.
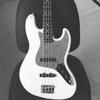
Lee Puckett
12,539 PointsDo I replace something with it?
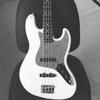
Lee Puckett
12,539 PointsGot it. Thanks!
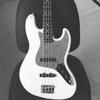
Lee Puckett
12,539 PointsI've learned that the body of the constructor is the { } below public Student().
That's good to know because before I thought {Courses = new List<Course>(); } was its own entity and therefore separate from the constructor, causing me to wonder whether I had to add a body ...{ }, that the body was ( ), or that the body was located in open space either above or below the constructor.
Lack of knowledge about the vocabulary and theory force me to use visual patterns for problem solving tools, but like all disciplines, that will only take you so far.