Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial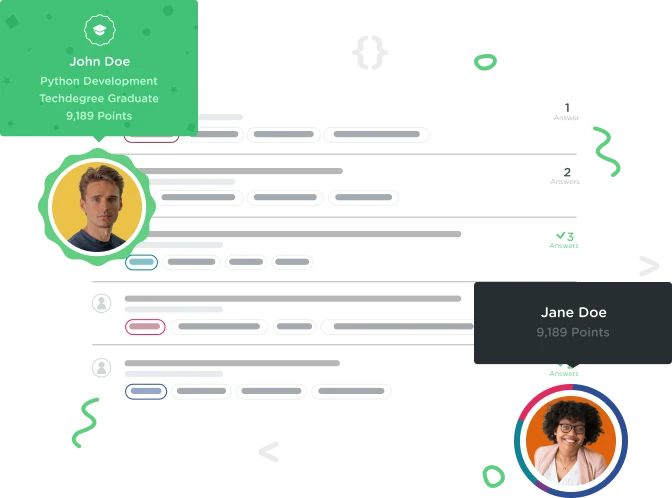

Maya Banitt
674 PointsTask 3 of Slicing with a Step
Can someone please show me the answer to this task?
Make a function name first_and_last_4 that accepts an iterable and returns the first 4 and last 4 items in the iterable.
Thanks!
def first_4(my_variable):
return my_variable[0:4]
def odds(another_variable):
return another_variable[1::2]
def first_and_last_4(task3_variable):
return task3_variable[0:4]
def first_and_last_4(task3_variable):
return task3_variable[0:4:-1]
3 Answers
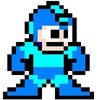
Robert Richey
Courses Plus Student 16,352 PointsHi Maya,
This is a tricky one, but we can get through this.
It looks like you've defined the function first_and_last_4
twice. Although this isn't an error, what ends up happening is the last definition will be used by the program, so let's take a look at that.
def first_and_last_4(task3_variable):
return task3_variable[0:4:-1]
In the slice, you're asking the program to start at index 0, go up to (but not include) index 4, and use a step of -1. What we want, is to return the first four items and last four items of task3_variable
.
You already know how to get the first 4, so now we need to add to that, the last 4 items. How might we get the last 4 items? And then, how would you add it to the first 4?

Gavin Ralston
28,770 PointsYou've got two functions named the same thing.
So one way to do it, rather than re-defining the first_and_last_4 function again, is to just add the next line of code (say, the slice that grabs the last 4 items in the iterable) and then return both of the results you got from your slices.

Maya Banitt
674 PointsI now have return task3_variable[0:4] + task3_variable[45:-1] and it says this returns [1, 2, 3, 4, 46, 47, 48], but the program is looking for [1, 2, 3, 4, 46, 47, 48, 49]. I thought the -1 would make it end on the last variable, but apparently it's not. How do I make it go to 49?

Gavin Ralston
28,770 PointsThink about it like this: If you're working backwards, you won't be starting from an index of 0, so in order to get to something up to and including the index you want, you need to end one index further than the ones you want to capture. Going from beginning to end, since indexes start at 0, going to index 4 actually gets you four items: 0, 1, 2, and 3
Additionally, I'd highly recommend going back through the video(s) on slicing. If the code finishes the challenge, that's great, and it's a learning process, but.... think about how brittle a function like that would be in a "real world" example, where each list could be a different length. You'd want a function that gets the first and last four to be good at all of them, not just one particular example.
For instance, try examining the length of the list and using that to set your "stop index" for the end of the list items, if you want to go the current route you're taking.
Good luck!
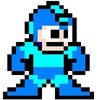
Robert Richey
Courses Plus Student 16,352 PointsAt about 3:58 in Slicing With A Step, Kenneth explains that we can use negative indexes, as well as negative steps.
It's really useful to experiment inside the python REPL, where you can quickly create lists and use slices to see the output.
my_list = list(range(20))
# [0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19]
print(my_list[:2]) # Output: [0, 1]
print(my_list[-2:]) # Output: [18, 19]

Gavin Ralston
28,770 PointsYep, negative index is your friend and doesn't require a negative step at all. :)
It's cool because it works by saying "start at x indeces from the end" and then reads right-to-left just like starting at a positive index would, saving you a ton of trouble.
Still, it can be a good way to grok slicing if you start messing around with start, stop, and step values!
Gavin Ralston
28,770 PointsGavin Ralston
28,770 PointsThere were some really good examples of how to traverse lists with slicing in these videos, if I recall correctly, too.
Maya Banitt, you can back to one of the videos and play around with slices a bit and it'll make a lot more sense. You've got the step portion right, for the last four, but if you want to go backwards from the end of the list, you'll definitely need to start at the end of the list.
Or reverse the order if you've come across that method in the course. :)
Maya Banitt
674 PointsMaya Banitt
674 PointsI now have return task3_variable[0:4] + task3_variable[45:-1] and it says this returns [1, 2, 3, 4, 46, 47, 48], but the program is looking for [1, 2, 3, 4, 46, 47, 48, 49]. I thought the -1 would make it end on the last variable, but apparently it's not. How do I make it go to 49?