Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial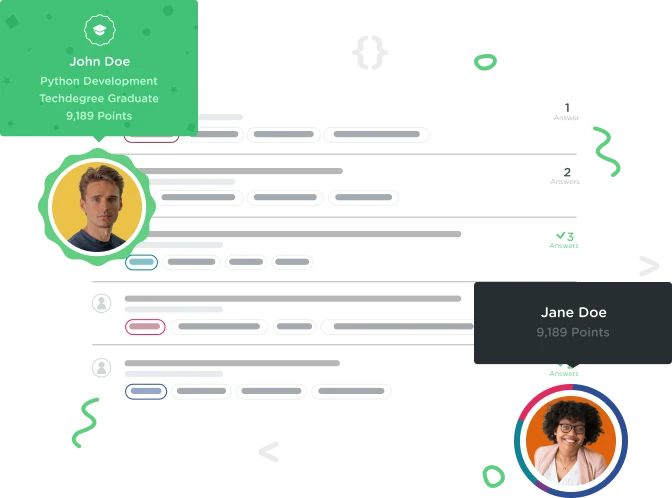

Hugo Madge Leon
4,143 PointsTask 4 (most_courses), I never get the right teacher name.
I have tried several ways of going about this problem, from very basic to more complex code, and none of them worked. Attached is my most recent attempt, which is not the cleanest but I have tried all the good ones. Any help appreciated xx
# The dictionary will look something like:
# {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
# Your code goes below here.
def num_teachers(teachers):
return len(teachers)
def num_courses(teachers):
total = 0
for course in teachers.values():
total += len(course)
return total
def courses(teachers):
courses = []
for course in teachers.values():
courses.extend(course)
return courses
def most_courses(teachers):
v = list(teachers.values())
k = list(teachers.keys())
most = 0
i = 0
counter = 0
for course_list in v:
number = len(course_list)
if number > most:
i = counter
most = number
counter += 1
return k[i]
2 Answers

Abdul Qadir
3,297 PointsThis is the solution I came up with, not sure if its the shortest one but worked for me:
def most_courses(dict):
max_count = 0
best_teacher = ""
for key,value in dict.items():
if len(value) > max_count:
max_count = len(value)
best_teacher = key
else:
continue
print(best_teacher)
return(best_teacher)

turaboy holmirzaev
19,198 Points-
num_teachers: this function takes a dictionary as input so you have to return the length of dictionary keys' list because the teacher names are the keys for this dictionary
Ex: mydict = {'a': "one", 'b': "two"} mydict.keys() # returns keys list
-
num_courses this function also takes a dictionary but its values are lists. So to get the number of courses in each list inside the dictionary you have to do nested "for loop". First iterate over the values of the dictionary and each iteration, iterate over the items in each list.
#Example mydict = {"a": [1, 2, 3], "b": [4, 5, 6]} for item in mydict.keys(): for number in item: print(number) #this way just keep track of the total number of courses
-
courses this function is similar to the second one. do the same nested for loop but this time instead of keeping track of total number of courses, just define empty list up top and append each course when you iterate over values
#Example mydict = {"a": [1, 2, 3], "b": [4, 5, 6]} numlist = [] for item in mydict.values(): for num in item: numlist.append(num)
-
most_courses this function also takes the same dictionary but you will have to keep track of max_count variable and teacher.
#example mydict = {"a": [1, 2, 3], "b": [4, 5, 6]} max_count = 0 my_letter = "" for letter in mydict.key(): if len(mydict[letter]) > max_count: my_letter = letter max_count = len(mydict[letter]) print(my_letter)
-
stats this function also takes same dictionary. This time you will have to iterate over dictionary.items() which return tuple from this tuple make a list and append this list to the main list.
#Example mydict = {"a": [1, 2, 3], "b": [4, 5, 6]} main_list = [] for item in mydict.items(): temp_list - [] temp_list.append(item[0]) temp_list.append(len(item[1])) main_list.append(temp_list) print(main_list)

Hugo Madge Leon
4,143 PointsThat didn't really help me.

Hugo Jeffreys
Python Web Development Techdegree Student 1,738 PointsHi turaboy holmirzaev. Thanks for that breakdown of this task. I found that really helpful!