Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial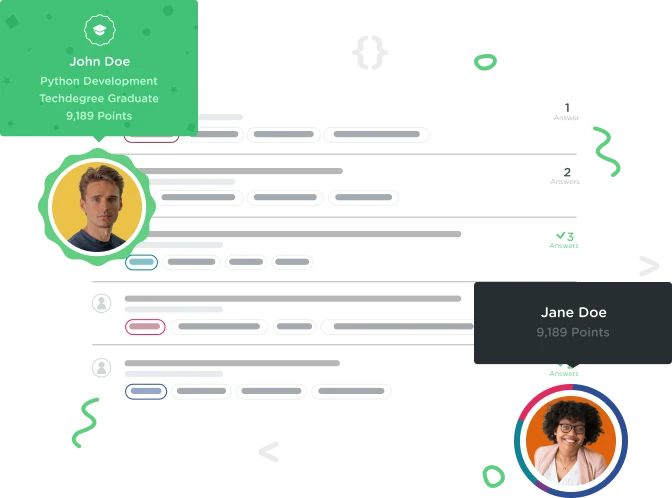

Tinashe Gwariro
4,691 PointsTask 4 of challenge uncomment comments
I have tried everything i keep getting an error not sure what i am doing wrong
This is the error: Are you sure you used the arguments passed in from the main method in Main.java? args[0] is the firstName and args[1] should be the last name
public class Forum {
private String topic;
// TODO: add a constructor that accepts a topic and sets the private field topic
public Forum(String topic) {
//set mTopic to the topic passed in to the constructor
topic = topic;
}
public String getTopic() {
return topic;
}
public void addPost(ForumPost post) {
System.out.printf("A new post in %s topic from %s %s about %s is available",
topic,
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle()
);
}
}
public class User {
// TODO: add private fields for firstName and lastName
private String firstName;
private String lastName;
public User(String firstName, String lastName) {
// TODO: set and add the private fields
firstName = firstName;
lastName = lastName;
}
// TODO: add getters for firstName and lastName
public String getFirstName(){
return firstName;
}
public String getLastName(){
return lastName;
}
}
public class ForumPost {
private User author;
private String title;
private String description;
// TODO: add a constructor that accepts the author, title and description
public ForumPost(User author, String title, String description) {
this.author = author;
this.title = title;
this.description = description;
}
public User getAuthor() {
return author;
}
public String getTitle() {
return title;
}
public String getDescription() {
return description;
}
}
public class Main {
public static void main(String[] args) {
System.out.println("Beginning forum example");
if (args.length < 2) {
System.out.println("Usage: java Main <first name> <last name>");
System.err.println("<first name> and <last name> are required");
System.exit(1);
}
Forum forum = new Forum("Java");
String firstName = args[0];
String lastName = args[1];
User author = new User(firstName, lastName);
ForumPost post = new ForumPost(author, "Programming is awesome", "Cuz it makes us create new stuff");
forum.addPost(post);
}
}
1 Answer

andren
28,558 PointsThe issue is the constructor you have written for the Forum
and User
class.
Take a look at the constructor for ForumPost
(which is correct):
public ForumPost(User author, String title, String description) {
this.author = author;
this.title = title;
this.description = description;
}
And then compare it to the constructor for User
(which is incorrect):
public User(String firstName, String lastName) {
// TODO: set and add the private fields
firstName = firstName;
lastName = lastName;
}
Can you notice any difference between those two constructors beyond the names used?
The difference is the lack of the this
keyword. When you have a field variable and a parameter that has the same name, you have to use the this
keyword to help Java distinguish between the two.
So you have to change the User
constructor like this:
public User(String firstName, String lastName) {
// TODO: set and add the private fields
this.firstName = firstName;
this.lastName = lastName;
}
The same issue applies to the Forum
constructor, which also has to be fixed like this:
public Forum(String topic) {
//set mTopic to the topic passed in to the constructor
this.topic = topic;
}
Once you fix those constructors your code should work fine.
Tinashe Gwariro
4,691 PointsTinashe Gwariro
4,691 PointsAwesome it actually worked thank you