Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial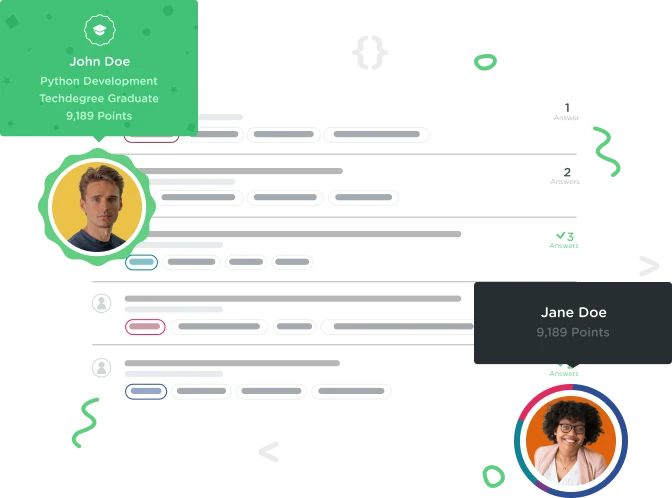

Eric Park
1,063 PointsTask Incomplete not displaying in console
I am having some trouble with the Javascript ToDo App. Whenever I uncheck a list item, the console does not log "task incomplete" as I have specified. Here is some of my code:
index.html
<!DOCTYPE html>
<html>
<head>
<title>Todo App</title>
<link href='http://fonts.googleapis.com/css?family=Lato:300,400,700' rel='stylesheet' type='text/css'>
<link rel="stylesheet" href="css/style.css" type="text/css" media="screen" charset="utf-8">
</head>
<body>
<div class="container">
<p>
<label for="new-task">Add Item</label><input id="new-task" type="text"><button>Add</button>
</p>
<h3>Todo</h3>
<ul id="incomplete-tasks">
<li><input type="checkbox"><label>Pay Bills</label><input type="text"><button class="edit">Edit</button><button class="delete">Delete</button></li>
<li class="editMode"><input type="checkbox"><label>Go Shopping</label><input type="text" value="Go Shopping"><button class="edit">Edit</button><button class="delete">Delete</button></li>
</ul>
<h3>Completed</h3>
<ul id="completed-tasks">
<li><input type="checkbox" checked><label>See the Doctor</label><input type="text"><button class="edit">Edit</button><button class="delete">Delete</button></li>
</ul>
</div>
<script type="text/javascript" src="js/app.js"></script>
</body>
</html>
app.js
//Problem: User interaction doesn't provide desired results.
//Solution: Add interactivity so that the user can manage daily tasks
var taskInput = document.getElementById("new-task"); //new-task
var addButton = document.getElementsByTagName("button")[0]; //first button on page
var incompleteTasksHolder = document.getElementById("incomplete-tasks"); //incomplete-tasks
var completedTasksHolder = document.getElementById("completed-tasks"); //completed-tasks
//New Task List Item
var createNewTaskElement = function(taskString) {
//Create List Item
var listItem;
//input {checkbox}
//label
//input {{text}}
//button.edit
//button.delete
//Each element will need to be modified and appended
return listItem;
}
//Add a new task
var addTask = function(){
console.log("Add task");
//When the button is pressed, we want to add a task to create a task
//Create a new list item with the text from #new-task:
createNewTaskElement("Some New Task");
}
//Edit an existing task
var editTask = function(){
console.log("Edit task");
//When the edit button is pressed
//if the class of the parent is .editMode
//Switch from editMode
//Label text become the input's value
//else
//Switch to .editMode
//input value becomes the label's text
//Toggle .editMode on the parent
}
//Delete an existing task
var deleteTask = function(){
console.log("Delete task");
//When delete button is pressed
//Remove the parent list item from the ul
}
//Mark a task as complete
var taskCompleted = function(){
console.log("Task complete");
//When the checkbox is checked
//Append the task list item to the #completed-tasks
}
//Mark a task as incomplete
var taskIncomplete = function(){
console.log("Incomplete task");
//When the checkbox is unchecked
//Append the task list item to the #incomplete-tasks
}
var bindTaskEvents=function(taskListItem, checkBoxEventHandler){
console.log("Bind list item events");
//Select listItem's its children
var checkBox = taskListItem.querySelector("input[type=checkbox]");
var editButton = taskListItem.querySelector("button.edit");
var deleteButton = taskListItem.querySelector("button.delete");
//Bind editTask to edit button
editButton.onclick = editTask;
//Bind deleteTask to delete button
deleteButton.onclick = deleteTask;
//Bind checkBoxEventHandler to checkbox
checkBox.onchange = checkBoxEventHandler;
}
//Set the click handler to the addTask function
addButton.onclick = addTask;
//Cycle over the incompleteTasksHolder ul list
for(var i = 0; i < incompleteTasksHolder.children.length; i++){
bindTaskEvents(incompleteTasksHolder.children[i],taskCompleted)
}
//For each list item
//Bind events to list item's children(taskCompleted)
//Cycle over the completedTasksHolder ul list
for(var i = 1; i < completedTasksHolder.children.length; i++){
bindTaskEvents(completedTasksHolder.children[i], taskIncomplete)
}
Thanks
Julian Gutierrez
19,201 PointsJulian Gutierrez
19,201 Points*Fixed markdown