Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial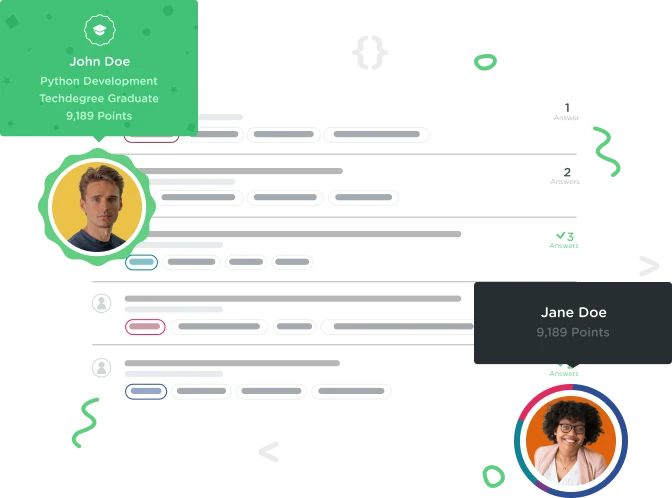
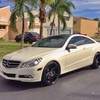
Mohamed KE
Courses Plus Student 1,583 PointsTask is completed but im curious to know if there are any shortcuts or "Better Practices"
/*
....:::::::......::::::.....:: QUIZ OF 3 CROWNS ::......:::::::.....::::::....
BY: Mohd K-Eddine
Ver: 1.0
*/
// List of User Answers + Correct Answers
var userAnswer1 = prompt('What programming language is this written in?');
var correctAnswer1 = 'JavaScript'; // 2 OPTIONS ----------- JavaScript - JS
var userAnswer2 = prompt('In what City can the Eiffel Tower be found?');
var correctAnswer2 = 'Paris'; // 1 OPTION ----------------- Paris
var userAnswer3 = prompt('The highest mountain in the world?');
var correctAnswer3 = 'Everest'; // 3 OPTIONS -------------- Everest / Mount Everest / Everest Mountain -----
var userAnswer4 = prompt('The Currency used in the United States of America?');
var correctAnswer4 = 'Dollars'; // 3 OPTIONS -------------- DOLLARS / $ / USD -----
var userAnswer5 = prompt('How many planets does the Solar System hold?');
var correctAnswer5 = 'Eight'; // 4 OPTIONS ---------------- Eight / Eight planets / 8 / 8 planets -----
// Question 1 - (IF ELSE STATEMENT)
if ( userAnswer1.toUpperCase() === correctAnswer1.toUpperCase() || userAnswer1.toUpperCase() === 'JS') {
document.write('<p>Correct! ' + '<strong>' + correctAnswer1 + '</strong>' + ' is the Programming language.</p>');
var idAnswer1 = 1;
}
else {
var idAnswer1 = 0;
}
// Question 2 - (IF ELSE STATEMENT)
if ( userAnswer2.toUpperCase() === correctAnswer2.toUpperCase()) {
document.write('<p>Correct! ' + '<strong>' + correctAnswer2 + '</strong>' + ' is where the Eiffel Tower is located.</p>');
var idAnswer2 = 1;
}
else {
var idAnswer2 = 0;
}
// Question 3 - (IF ELSE STATEMENT)
if ( userAnswer3.toUpperCase() === correctAnswer3.toUpperCase() ||
userAnswer3.toUpperCase() === 'Mount Everest'.toUpperCase() ||
userAnswer3.toUpperCase() === 'Mt Everest'.toUpperCase() ||
userAnswer3.toUpperCase() === 'Mt. Everest'.toUpperCase() ||
userAnswer3.toUpperCase() === 'Everest mountain'.toUpperCase()
) {
document.write('<p>Correct! ' + '<strong>' + correctAnswer3 + '</strong>' + ' is the tallest Mountain in the World.</p>');
var idAnswer3 = 1;
}
else {
var idAnswer3 = 0;
}
// Question 4 - (IF ELSE STATEMENT)
if ( userAnswer4.toUpperCase() === correctAnswer4.toUpperCase() ||
userAnswer4 === '$' ||
userAnswer4.toUpperCase() === 'USD'.toUpperCase()
) {
document.write('<p>Correct! ' + '<strong>' + correctAnswer4 + '</strong>' + ' is the Currency of the U.S.A.</p>');
var idAnswer4 = 1;
}
else {
var idAnswer4 = 0;
}
// Question 5 - (IF ELSE STATEMENT)
if ( userAnswer5.toUpperCase() === correctAnswer5.toUpperCase() ||
userAnswer5.toUpperCase() === 'Eight Planets'.toUpperCase() ||
userAnswer5.toUpperCase() === '8' ||
userAnswer5.toUpperCase() === '8' + ' Planets'.toUpperCase()
) {
document.write('<p>Correct! ' + 'That is the correct number for the planets on the Solar System, ' + '<strong>' + correctAnswer5 + '.</strong></p>');
var idAnswer5 = 1;
}
else {
var idAnswer5 = 0;
}
// USER SCORE - CORRECT QUESTIONS
var totalQuestions = 5;
var totalCorrectQuestions = idAnswer1 + idAnswer2 + idAnswer3 + idAnswer4 + idAnswer5;
document.write('<p>You just scored ' + '<strong>' + totalCorrectQuestions + '</strong>' + ' out of ' + '<strong>' + totalQuestions + '</strong> questions.</p>' )
// USER GRADE - GOLD CROWN (5/5) | SILVER CROWN (3-4/5) | BROWN CROWN (1-2/5) | NO CROWN (0/0)
if ( totalCorrectQuestions === 5) {
alert('GOLD CROWN attained - You born winner !');
}
else if (totalCorrectQuestions === 4 || totalCorrectQuestions === 3 ) {
alert('SILVER CROWN attained - Congratulations !');
}
else if (totalCorrectQuestions === 2 || totalCorrectQuestions === 1 ) {
alert('BROWN CROWN attained - Not bad !');
}
else if (totalCorrectQuestions === 0) {
alert('You loser. Come back next time. I mean come on, not even 1 question right??');
}
I appreciate any comments/suggestions from the 'more experienced'
Thanks in advance
MsKe,
1 Answer
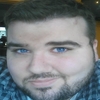
Marcus Parsons
15,719 PointsHey Mohamed Kamar eddine,
The way you're doing your program is fine. But, if you want to take a look at alternative way, that is shorter, here is something you can do. This is what I modified your question 5 to be and it works the same way as yours does, but with just less memory. The only difference is that I had taken out all of the idAnswer variables and just initialized totalCorrectQuestions
at the very top and then added to it every time you got the correct answer:
([correctAnswer5.toUpperCase(), "8"].indexOf(userAnswer5.toUpperCase()) > -1) ? (totalCorrectQuestions++ && document.write('<p>Correct! ' + 'That is the correct number for the planets on the Solar System, ' + '<strong>' + correctAnswer5 + '.</strong></p>')) : return false;
If you've never seen shorthand logic before, this might seem really confusing. Here is a simple example of it:
var number = 10;
(number > 9) ? alert("The number is greater than 9! W00t!") : alert("The number is not greater than 9! Bummer!");
which is the same as saying:
var number = 10;
if (number > 9){
alert("The number is greater than 9! W00t!");
}
else{
alert("The number is not greater than 9! Bummer!");
}
So, number is 10, and the (number > 9) ?
checks to see if number is greater than 9. If whatever is within those () evaluates to true, then it executes alert("The number is greater than 9! W00t!")
and if it evaluates to false, it executes alert("The number is not greater than 9! Bummer!")
. Try running that code in your browser's console and playing with it. And then move on to the next part of this answer.
The first part:
([correctAnswer5.toUpperCase(), "8"].indexOf(userAnswer5.toUpperCase()) > -1)
checks to see if the upper case version of "userAnswer5" has an index of whatever is in the array ("EIGHT" or "8") because if it finds an index of "EIGHT" or "8", it will be greater than -1 and will return true.
Now, I know you had added two more special cases to your if statement checking for "Eight planets" or "8 planets". But, since both of those strings have an answer we're looking for ("eight" or "8") we don't need to include them in our check. When you are checking strings with indexOf() you only need to include absolutely unique strings that don't contain anything we've previously checked.
The second part:
? (totalCorrectQuestions++ && document.write('<p>Correct! ' + 'That is the correct number for the planets on the Solar System, ' + '<strong>' + correctAnswer5 + '.</strong></p>')) : return false;
is just like saying:
if ([correctAnswer5.toUpperCase(), "8"].indexOf(userAnswer5.toUpperCase()) > -1) {
totalCorrectQuestions++;
document.write('<p>Correct! ' + 'That is the correct number for the planets on the Solar System, ' + '<strong>' + correctAnswer5 + '.</strong></p>');
}
else{
return false;
}
Once you get used to shorthand notation, it's not bad and in fact can save some memory, although by today's standards its more than likely going to be a negligible amount.
I hope that wasn't too confusing! If it was, look up "shorthand javascript logic" and you can see tons of examples online for it.
Cheers! :)