Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial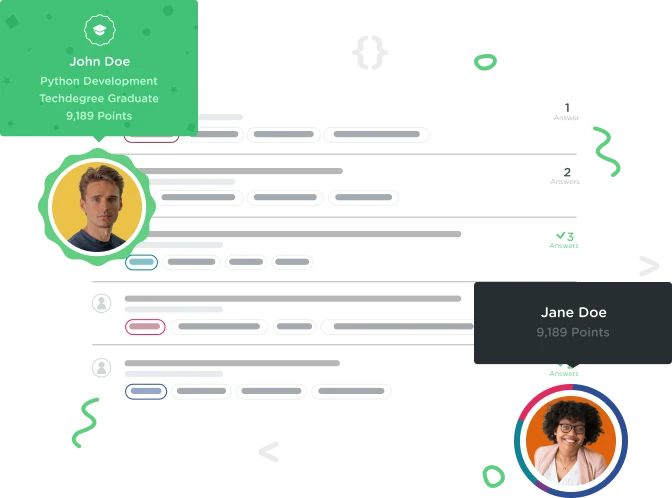
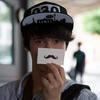
charlesmonaghan
742 PointsTbh, I have absolutely no idea how to do this one
It'd be great to have some help, and to be given some tips for the future! :)
import random
start = 5
while start == True:
number = random.randint(1, 99)
if number == even_odd:
print("{} is even".format(number))
else:
print("{} is odd".format(number))
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
1 Answer
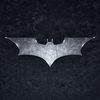
Logan R
22,989 PointsHey!
So you are very close to a right answer! The first thing I would like to point out is that in challenge 3, it explicitly says near the end: "Finally, decrement start by 1.
" You need to subtract 1 from start at the end of loop in your while statement.
The second issue is the statement "if number == even_odd:
".
Take a close look at this. You are asking if the number is equal to a function, not the return value of the function. This is because you are not actually calling the function.
IE:
>>> def even_odd(num):
... return not num % 2
>>> print ( even_odd )
<function even_odd at 0x1091092a8>
>>> print ( even_odd(10) )
True
The other issue with this if statement is that it returns a boolean of True
or False
(True for even, False for odd). You do not want to compare the number to this value.
The last issue is that you are calling the even_odd() function before you declare it. You will want to move the while loop after you declare the function or else Python will say that it has no idea what function you are talking about because it hasn't gotten that far in the script yet.
Hopefully this helps you out some! If not, feel free to leave a comment below and I'll try my best to further explain any issues or someone else may be able to come along and explain it better than I can :)