Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial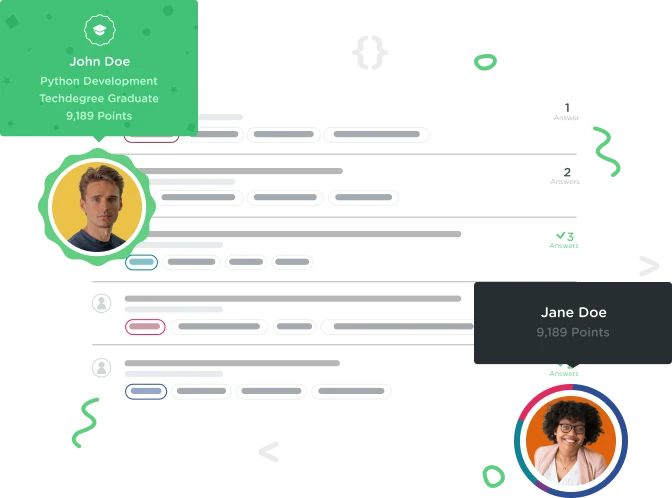
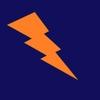
Saeed Gohar
3,066 PointsTeacher Stat Challenge Task 4 of 5.... Need help getting number of teachers and comparing them
Alright for my code, I need help with the most_courses function. This is where I added a skeleton structure but will need help figuring out how to compare multiple teachers instead of just 2. Any suggestions/help?
# The dictionary will look something like:
# {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Each key will be a Teacher and the value will be a list of courses.
#
# Your code goes below here.
def num_teachers(arg):
return len(arg)
def num_courses(arg):
return sum(len(v) for v in arg.values())
def courses(teachers):
# create empty list named 'stuff'
stuff = []
# extend the values into the empty list--now it should have 2 lists in the list
stuff.extend(teachers.values())
# Sketchy/Questionable part: Created a variable with the number of values that was in the dictionary
# Need to figure out how to loop that as many times as the values and add it to the list
numbers = len(teachers)
index = 0
stuffed = []
while index != numbers:
stuffed += stuff[index]
index += 1
return stuffed
def most_courses(teachers):
# Create empty list
stuffed3 = []
# Append the keys to the empty list
stuffed3.extend(teachers.keys())
# Sketchy/Questionable part: Return teacher name with the most courses
# Get number of teachers, subtracting 1 to take into account index starting at 0
numbers = len(teachers) - 1
index = 0
while index != numbers:
if len(stuffed3[0]) > len(stuffed3[1]):
return stuffed3[0]
else:
return stuffed3[1]
1 Answer

Øyvind Andreassen
16,839 PointsThis solution will solve the challenge
def most_courses(arg):
max_count = 0
teachers = " "
for teacher, listOfCourses in arg.items():
if len(listOfCourses) > (max_count):
max_count = len(listOfCourses)
teachers = teacher
return teachers
This function will return the first teacher in the dict with the most courses, and will not take into consideration that there can be more teachers that have the same amount of courses. But it will give you a pass on the challenge.