Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial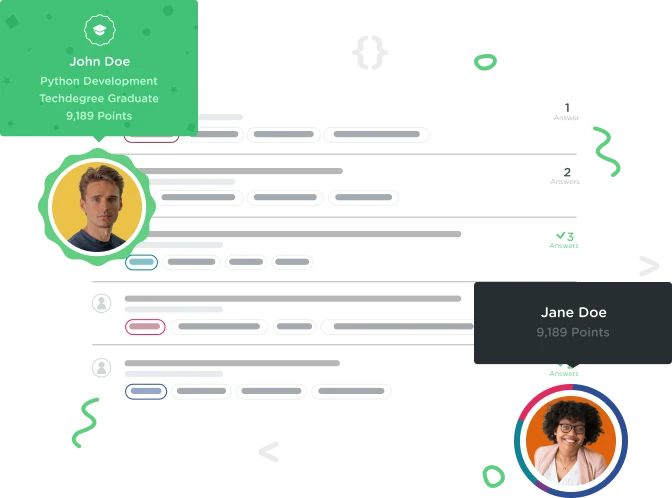
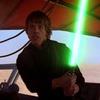
darrian thomas
8,481 PointsTeacher stats
I'm having a lot of trouble with this code challenge. Up until this challenge I thought I understood everything, but trying to figure out how to loop through the dictionary, find the teacher with the most classes then assign that value to 'max_count' to find and return the 'busiest_teacher' is rly kicking this noob around
here is my code
teachers = {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'], 'Kenneth Love': ['Python Basics', 'Python Collections']}
def most_classes(teachers):
busy_teacher = ""
max_count = 0
for teacher in teachers:
if len(teachers[teacher]) > max_count:
max_count = len(teachers[teacher])
busy_teacher = teacher
return teacher
print(most_classes(teachers))
i guess you could say i understand what to do just not how to do it. i dont understand why this code is returning back both keys in workspaces. also i didnt even know how to really set this up and had to look for help on the forums here and i still can't get it lol
someone pls help
3 Answers
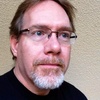
Chris Freeman
Treehouse Moderator 68,428 PointsYour code is actually very close. Your return
statement is inside your for
loop and is returning from the function most_classes
with the first teacher seen. Due to dictionary hashing, it might not always be the same teacher.
The fix is to unindent the return, and return busy_teacher
not teacher
:
def most_classes(teachers):
busy_teacher = ""
max_count = 0
for teacher in teachers:
if len(teachers[teacher]) > max_count:
max_count = len(teachers[teacher])
busy_teacher = teacher
return busy_teacher # <-- fixed return
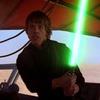
darrian thomas
8,481 Pointsthanks. can you explain in a little more depth what the for loop is doing?
i know at first im looping through both keys then seeing if the length of the values inside each key are greater than max_count which it will be since max_count is zero then im passing the same value into max_count and assigning the busiest teacher but how am i getting the teacher with the greatest value or the most classes?
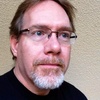
Chris Freeman
Treehouse Moderator 68,428 PointsThe code for teacher in teachers:
is shorthand for for teacher in teachers.keys():
so you are looping over the keys in the dict teachers
. The value of the dict key is the teachers name, so looping over the keys is actually looping over the teachers names. Thus, capturing the current teacher with the new max is done by reference the loop variable teacher
.
To see this a different way, you could also loop over both the key and the value of each dict entry:
for teacher, courses in teachers.items(): # <-- return key, value pair for each dict entry
if len(courses) > max_count:
max_count = len(courses)
busy_teacher = teacher
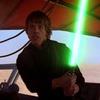
darrian thomas
8,481 Pointsi found the first step was hard to start but thanks that makes things a little more clear