Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial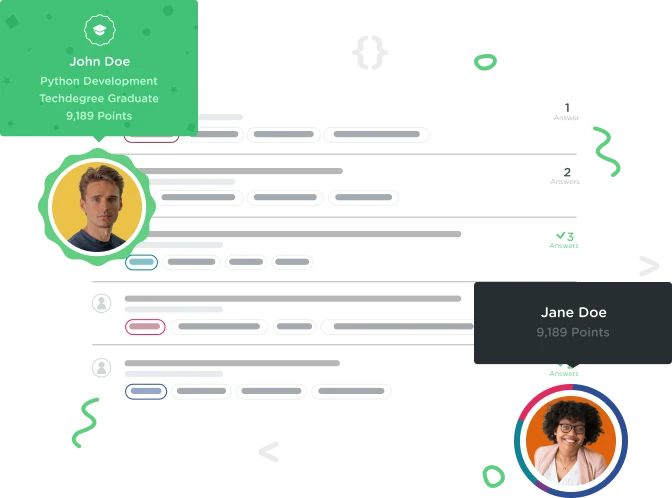

Frank Sherlotti
Courses Plus Student 1,952 PointsTeacher stats: Challenge 4 of 4.. Could use a some help please(:.
QUESTION: -- Write a function named courses that takes the dictionary of teachers. It should return a list of all of the courses offered by all of the teachers. -- I can't figure out how i'm supposed to return all 18 courses it's asking for. I've gotten this one which only returns one course. Any help would be greatly appreciated, thanks!
def courses(a_dict):
classes = []
for key, value in a_dict.items():
classes = [len(value)]
return classes
And this one which returns 5 of the 18 courses.
def courses(a_dict):
classes = []
courses = []
for key, value in a_dict.items():
classes = [len(value)]
courses.append(classes)
return courses
1 Answer

Dan Johnson
40,533 PointsThe courses list is expected to contain the names of all courses, so you don't need to use the len function.
Your first function only returns one value because each time you call:
classes = [len(value)]
You're assigning a new list to classes which will cause you to lose the reference to the last one.
You're next function is creating a list of lists where each nested list contains the number of courses for one teacher.
Here's an outline of a solution to grab all the course names:
def courses(teachers):
all_courses = []
# For every course list (value) in the teachers dictionary
# Add each item from the course list to the all_courses list
return all_courses
You may want to use the extend method to avoid extra loops.
Frank Sherlotti
Courses Plus Student 1,952 PointsFrank Sherlotti
Courses Plus Student 1,952 PointsNow it's saying i'm returning 25 classes instead of the requested 18, i'm confused.
Dan Johnson
40,533 PointsDan Johnson
40,533 PointsYou want to extend the values, you're extending the keys. Try something like this:
for value in teachers.values():
Frank Sherlotti
Courses Plus Student 1,952 PointsFrank Sherlotti
Courses Plus Student 1,952 PointsStill at 25 courses. I feel like i'm missing something inside the for loop but I don't know what it is.
Dan Johnson
40,533 PointsDan Johnson
40,533 PointsI just noticed you're extending the dictionary itself. You'll want to use the value variable as that is what is referencing the elements during the iteration:
Frank Sherlotti
Courses Plus Student 1,952 PointsFrank Sherlotti
Courses Plus Student 1,952 PointsThanks I really do appreciate the help!