Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial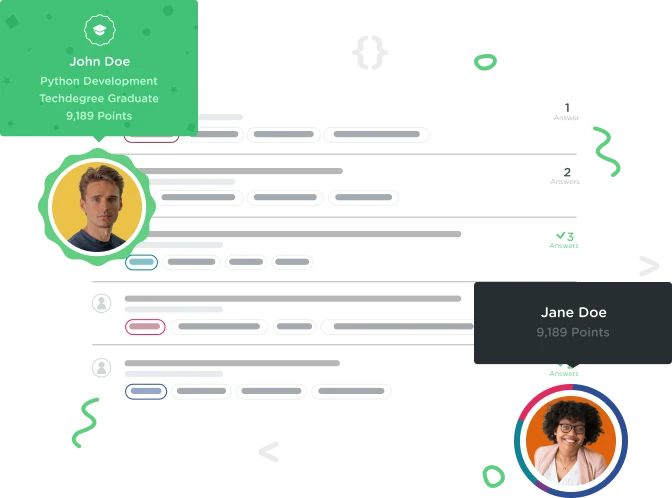
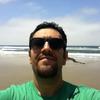
Michael Escoto
30,028 PointsTeacher Stats Task 1 of 3
I'm completely stumped on how to make this work.
Am I on the right path or can I refactor this code to make this easier?
def most_classes(dicts):
teachers = list(dicts.keys())
classes = list(dicts.values())
max_count = 0
i = 0
j = 0
for items in classes:
if len(classes[i]) > max_count:
max_count = len(classes[i])
i += 1
for teacher in teachers:
if ____ == max_count:
return teacher[j]
j += 1
2 Answers
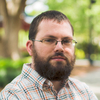
Kenneth Love
Treehouse Guest TeacherI don't want to give the whole answer away. :D Take a look at this:
counts = []
for key in my_dict:
counts.append(len(my_dict[key]))
Now counts
will hold onto the length of the value of each key in the dictionary. You'll need some functionality similar to this to solve the code challenge.
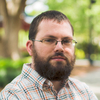
Kenneth Love
Treehouse Guest TeacherLet's break it down outside of programming.
You have a directory of teachers with all of their classes listed after them. Or maybe it's actors and their awards. Or car manufacturers and their models of cars. As you read through the list, you count the number of things they have and keep track of who has the most and how many they had.
So do that here. Hold onto the biggest number of classes (this would start at 0) and what teacher has those (this would start as None
or just an empty string). Now go through each teacher in the dictionary and see if they have a larger roster of classes than your current highest count. If so, up the high count and change the saved teacher name. If not, move on to the next teacher.
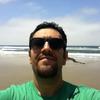
Michael Escoto
30,028 PointsOkay, conceptually I get the idea but I'm not sure how to get there programmatically.
One problem I keep running into is counting the number of values inside the list inside the dictionary. If I run the program one time it will come back with a count of 2 the first time and 3 the next time.
Michael Escoto
30,028 PointsMichael Escoto
30,028 PointsIt took a while for it to sink in but I finally got it. Thank you.
Kenneth Love
Treehouse Guest TeacherKenneth Love
Treehouse Guest TeacherMichael Escoto Awesome!
I know it can be a little frustrating sometimes but great job sticking with it. If there was any part of it that you felt was way too confusing/hard, let me know. I'm more than happy to improve the course as much as I can.
Kevin Lozandier
Courses Plus Student 53,747 PointsKevin Lozandier
Courses Plus Student 53,747 PointsI found the dictionary confusing since it's eccentrically modeled?:
Shouldn't it be designed the following way to be more usable, more believable (that it came from a database and that they're related to each other, and more easily programmable towards being more applicable beyond the challenge?
{name: "Teacher Name", classes: ["class1", "class2", "class3", "class4" }
I'm still confused by this challenge, but that's great: I never had a problem with such a max count challenge before (It's humbling even).
To clarify I was able to get the following to work:
The problem with this is python3 gives me the following error outside of the challenge (I prefer completing the challenges with Vim):
TypeError: tuple indices must be integers, not dict.
This may need to be considered with future challenges involving iterating through a dictionary; I again think an array of dictionaries with
name
andclasses
more intuitive and more pragmatic than the current dictionary for this problem.Kenneth Love
Treehouse Guest TeacherKenneth Love
Treehouse Guest TeacherKevin Lozandier if it was modeled that way, we'd need one dict per teacher. As it is, we only need one dict for the whole teaching staff.