Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial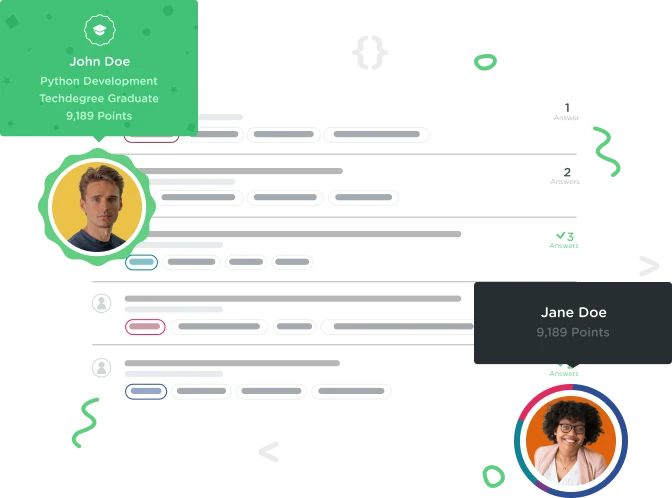
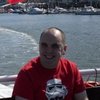
Sean Flanagan
33,236 PointsTeacher Stats, Task 3
Hi.
Can anyone see what's wrong with this please?
I'd appreciate any help.
Cheers
Sean :-)
# The dictionary will be something like:
# {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Often, it's a good idea to hold onto a max_count variable.
# Update it when you find a teacher with more classes than
# the current count. Better hold onto the teacher name somewhere
# too!
#
# Your code goes below here.
def most_classes(teachers_dict):
count = 0
for key in teachers_dict:
if len(teachers_dict[key]) > count:
count = len(teachers_dict[key])
answer = key
return answer
def num_teachers(teachers_dict):
return len(teachers_dict)
def stats(teachers_dict):
int num_of_classes = []
for teacher_name in teachers_dict:
new_list = [teacher_name, len(num_of_classes)]
num_of_classes.append(new_list)
return num_of_classes
1 Answer

Ryan S
27,276 PointsHi Sean,
You are very close but there are a couple things to note
First you don't have to declare a variable as an "int" as you are doing with num_of_classes. Python doesn't require the definition of variable types. This variable is an empty list, so its simply:
num_of_classes = []
The second thing is in your for loop with the variable "new_list". You have the right idea, but notice how you are using len(num_of_classes)
. You have initially defined num_of_classes as an empty list so its length will be zero right from the start. What you actually want to extract out of teachers_dict, along with teacher_name, is the associated value in the dictionary.
So it would look like this:
new_list = [teacher_name, len(teachers_dict[teacher_name])]
The rest of your code looks good and changing these two things should make it work.
Hope this helps.
Sean Flanagan
33,236 PointsSean Flanagan
33,236 PointsHi Ryan.
That worked perfectly. I've up-voted your post and given you Best Answer.
Thanks for your help.
Sean :-)