Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial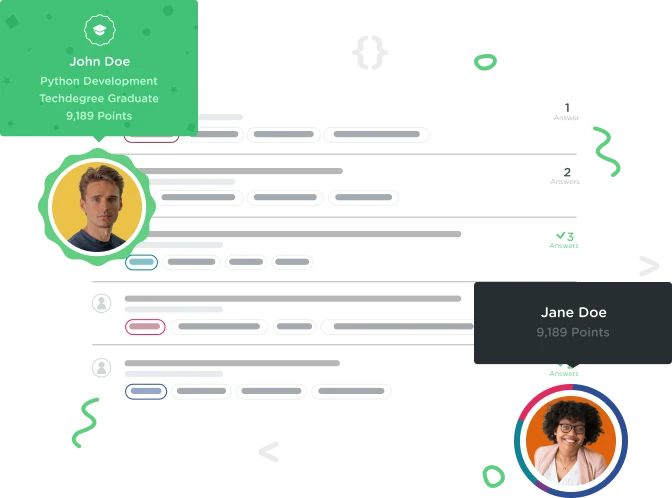

Christine Maxwell
Courses Plus Student 5,103 PointsTeachers and classes challenge
Here is my code, I'm a bit stack, please help.
def most_classes(teachers):
teachers = {'n1': ['y1', 'y2'], 'n2': ['u1', 'u2', 'u3'], 'n3': ['g1']}
for teacher in teachers:
for lessons in teachers.value():
if len(lessons) == 1:
print(teacher)
elif len(lessons) > 1:
print(teacher)
6 Answers
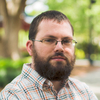
Kenneth Love
Treehouse Guest TeacherCan you walk us through your thought process for the above code? I think I see where you're going, but I'm not certain.
This step of the challenge wants you to give back the name of the teacher with the most classes, so you need a way to keep track of the current high scoring teacher and that high score as you loop through the dictionary. Also, .value()
doesn't exist, you want .values()
if you just want the values from the dictionary (you probably want .items()
instead, though).

Christine Maxwell
Courses Plus Student 5,103 PointsOkay, I will try to explain what I'm having problem with...
1st I define a function called
most_classes(teachersDic):
2nd I have to make empty variables that's going to hold my
teachers_list = []
classes_list = []
max_count = []
teacher_with_max_count = []
3rd I loop in teachersDic to find out my teachers_list
for teachers in teachersDic:
we get teachers_list = [teachers]
but from here I'm stuck, how do I get individual teacher as variable with classes number, I know to get classes number I have to do: len(classes_list)
, however that will give me back the list of numbers.
also to compare the values can I use <, >, ==, <=, >= like in C code? If no, how should I compare the values to find out the largest?
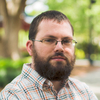
Kenneth Love
Treehouse Guest Teacheredited your post a bit for readability.
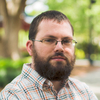
Kenneth Love
Treehouse Guest TeacherTo answer your last question first, yes, you can compare with <
, >
, ==
, <=
, and >=
just like in C.
We loop through a dictionary just like we do a list, but each step is only the key, not the key and the value. To get the value of a key, we have to ask the dictionary for it. So, for example, if I have a dict named teachers
and a key named 'Kenneth Love'
, I can get the value for that key with teachers['Kenneth Love']
.
In our challenge, each key (teacher's name) has a list of classes. So when I get teachers['Kenneth Love']
, I'm getting a list, which I can find the length of by using len()
. The basic start of the challenge's solution is:
def most_classes(teachers):
high_classes = 0
high_teacher = ''
Now, loop through teachers
, and on each step get the length of that teacher's classes (not the length of the teacher's name, be sure to get the value from the dictionary). If that length is higher than the current high_classes
variable, change high_classes
and change high_teacher
to that teacher's name. When you've gone through all of the teachers, return high_teacher
.

Christine Maxwell
Courses Plus Student 5,103 PointsI'm doing the Challenge Task 4 of 4
"Write a function named courses that takes the dictionary of teachers. It should return a list of all of the courses offered by all of the teachers."
With this code I get an error: "Bummer! You returned 5 courses, you should have returned 18." However when I run the test on Sublime it does the trick.
my code:
def courses(teachers_list):
all_courses = []
all_courses.extend(teachers_list.values())
return all_courses
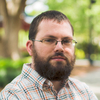
Kenneth Love
Treehouse Guest Teacher(I updated your answer for syntax highlighting)
You're adding a copy of each list to your list, so your returned list only has 5 items (one list per teacher, there are 5 teachers in the dictionary). You need to add the contents of each list.

Christine Maxwell
Courses Plus Student 5,103 Pointswhen I try to loop, like so:
def courses(teachers_list):
all_courses = []
for course in teachers_list.values():
all_courses.extend(course)
return all_courses
it returns courses one by one, with every next one adding the previous and with last one it gives the right result. How do I skip the adding process and get the result?
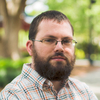
Kenneth Love
Treehouse Guest TeacherI'm not sure what you're asking here. You'll have to add to the all_courses
list to solve the problem.
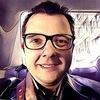
durul
62,690 PointsWhat is my code blog's problem ? Can someone fix for me.
def courses(teachers):
all_courses = []
for course in teachers.values():
all_courses.extend(course)
return all_courses
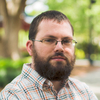
Kenneth Love
Treehouse Guest Teacherdurul dalkanat You need to indent the content of your for
loop.

Christine Maxwell
Courses Plus Student 5,103 PointsFor exp:
teachers = {'Jo': ['a1', 'a2', 'a3', 'a4', 'a5'], 'Mo': ['s1', 's2', 's3', 's4'], 'Lol': ['d1', 'd2']}
list_all = []
for list_of_classes in teachers.values():
list_all.extend(list_of_classes)
print (list_all)
in return I get this:
['s1', 's2', 's3', 's4']
['s1', 's2', 's3', 's4', 'a1', 'a2', 'a3', 'a4', 'a5']
['s1', 's2', 's3', 's4', 'a1', 'a2', 'a3', 'a4', 'a5', 'd1', 'd2']
the last thing it gives back is our right answer, however since it's looping through the dictionary the program adds values one by one from each key.
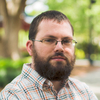
Kenneth Love
Treehouse Guest TeacherRight, but you're print()
ing at each step. We only want the list after the entire for
loop is finished.