Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial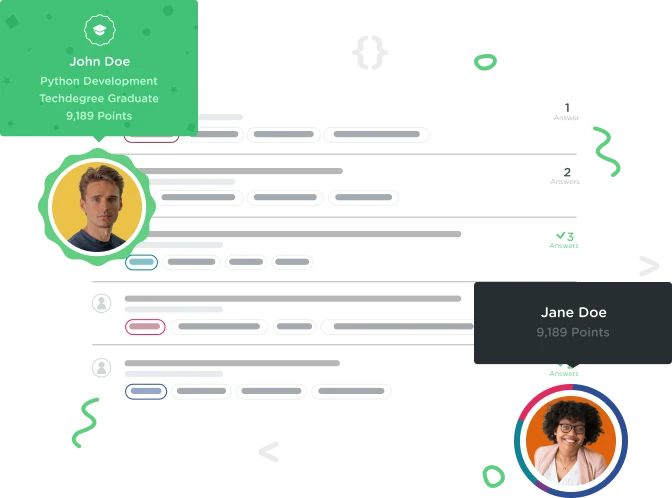
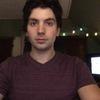
Jimmy Otis
3,228 PointsTeacher's notes: We could probably simplify the movement some if we used a tuple, like (-1, 0), instead of "LEFT".
I'm not sure what this would look like exactly or why it would even be considered simplified. Can someone try to show what this might look like? Thanks
1 Answer
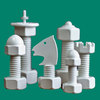
Steven Parker
231,275 PointsSo if "move" were a tuple with offsets instead of a direction word, the function might look like this:
def move_player(player, move):
x, y = player
return x + move[0], y + move[1]
Jimmy Otis
3,228 PointsJimmy Otis
3,228 PointsHmm. Sorry I'm still a bit confused. Move is an input variable that looks for the string 'left', 'right', 'up' or 'down' in a list and acts accordingly. How does this jive with making move a tuple?
Steven Parker
231,275 PointsSteven Parker
231,275 PointsThis is what the simpler function would look like if the program used "move" as a tuple instead of a string.
It would not be more simple to decode strings and convert to a tuple.
Jimmy Otis
3,228 PointsJimmy Otis
3,228 Pointsthis is what I currently have...
Steven Parker
231,275 PointsSteven Parker
231,275 PointsRight, and that makes sense by being more convenient for the user than typing in a pair of offsets to make a tuple. But I believe the comment in the Teacher's Notes was just a "what if" scenario.
Jimmy Otis
3,228 PointsJimmy Otis
3,228 Pointsoh, ok. I see. Basically we could make that function much shorter but than one would have to input (x, y) every time, rather than simply writing left, right, up, or down. The function would then take the index position of said tuple and merge it with the player's x and y and return this value?
However I suppose though that one would then have to write some code to make sure the player doesn't move more than one cell at a time so at the end of the day it's not really very practical, not to my newbie eyes at least.
Thanks for explaining, Steven
Steven Parker
231,275 PointsSteven Parker
231,275 PointsIf I'm understanding that comment correctly, then I agree. Perhaps if there's another interpretation I missed someone else will post an answer.
Chris Freeman
Treehouse Moderator 68,457 PointsChris Freeman
Treehouse Moderator 68,457 PointsThe conversion of user text to tuple could be simplified using a predefined dict:
Jimmy Otis
3,228 PointsJimmy Otis
3,228 PointsAhh! Why didn't I think of using a dictionary.. Wonderful, thanks